

Python List: How To Create, Sort, Append, Remove, And More
The Python list is one of the most used data structures, together with dictionaries . The Python list is not just a list, but can also be used as a stack and even a queue. In this article, I’ll explain everything you might possibly want to know about Python lists:
- how to create lists,
- modify them,
- how to sort lists,
- loop over elements of a list with a for-loop or a list comprehension ,
- how to slice a list,
- append to Python lists,
- … and more!
I’ve included lots of working code examples to demonstrate.
Table of Contents
- 1 How to create a Python list
- 2 Accessing Python list elements
- 3 Adding and removing elements
- 4 How to get List length in Python
- 5 Counting element occurrence in a list
- 6 Check if an item is in a list
- 7 Find the index of an item in a list
- 8 Loop over list elements
- 9 Python list to string
- 10 Sorting Python lists
- 12 Reversing Python lists
- 13 Learn more about Python lists
How to create a Python list
Let’s start by creating a list:
Lists contain regular Python objects, separated by commas and surrounded by brackets. The elements in a list can have any data type , and they can be mixed. You can even create a list of lists. The following lists are all valid:
Using the list() function
Python lists, like all Python data types, are objects. The class of a list is called ‘list’, with a lower case L. If you want to convert another Python object to a list, you can use the list() function, which is actually the constructor of the list class itself. This function takes one argument: an object that is iterable.
So you can convert anything that is iterable into a list. E.g., you can materialize the range function into a list of actual values, or convert a Python set or tuple into a list:
Accessing Python list elements
To access an individual list element, you need to know the position of that element. Since computers start counting at 0, the first element is in position 0, the second element is in position 1, etcetera.
Here are a few examples:
As you can see, you can’t access elements that don’t exist. In this case, Python throws an IndexError exception , with the explanation ‘list index out of range’. In my article on exceptions and the try and except statements , I’ve written about this subject more in-depth in the section on best practices. I recommend you to read it.
Get the last element of a list
If you want to get elements from the end of the list, you can supply a negative value. In this case, we start counting at -1 instead of 0. E.g., to get the last element of a list, you can do this:
Accessing nested list elements
Accessing nested list elements is not that much different. When you access an element that is a list, that list is returned. So to request an element in that list, you need to again use a couple of brackets:
Adding and removing elements
Let’s see how we can add and remove data. There are several ways to remove data from a list. What you use, depends on the situation you’re in. I’ll describe and demonstrate them all in this section.
Append to a Python list
List objects have a number of useful built-in methods, one of which is the append method. When calling append on a list, we append an object to the end of the list:
Combine or merge two lists
Another way of adding elements is adding all the elements from one list to the other. There are two ways to combine lists:
- ‘Add’ them together with the + operator.
- Add all elements of one list to the other with the extend method.
Here’s how you can add two lists together. The result is a new, third list:
The original lists are kept intact. The alternative is to extend one list with another, using the extend method:
While l1 got extended with the elements of l2, l2 stayed the same. Extend appended all values from l2 to l1.
Pop items from a list
The pop() method removes and returns the last item by default unless you give it an index argument.
Here are a couple of examples that demonstrate both the default behavior and the behavior when given an index:
If you’re familiar with the concept of a stack , you can now build one using only the append and pop methods on a list!
Using del() to delete items
There are multiple ways to delete or remove items from a list. While pop returns the item that is deleted from the list, del removes it without returning anything. In fact, you can delete any object, including the entire list, using del:
Remove specific values from a Python list
If you want to remove a specific value instead, you use the remove method. E.g. if you want to remove the first occurrence of the number 2 in a list, you can do so as follows:
As you can see, repeated calls to remove will remove additional twos, until there are none left, in which case Python throws a ValueError exception .
Remove or clear all items from a Python list
To remove all items from a list, use the clear() method:
Remove duplicates from a list
There is no special function or method to remove duplicates from a list, but there are multiple tricks that we can use to do so anyway. The simplest, in my opinion, is using a Python set . Sets are collections of objects, like lists, but can only contain one of each element. More formally, sets are unordered collections of distinct objects.
By converting the list to a set and then back to a list again, we’ve effectively removed all duplicates:
In your own code, you probably want to use this more compact version:
Since sets are very similar to lists, you may not even have to convert them back into a list. If the set offers what you need, use it instead to prevent a double conversion, making your program a little bit faster and more efficient.
Replace items in a list
To replace list items, we assign a new value to a given list index, like so:
How to get List length in Python
In Python, we use the len function to get the length of objects. This is true for lists as well:
If you’re familiar with other programming languages, like Java, you might wonder why Python has a function for this. After all, it could have been one of the built-in methods of a list too, like my_list.len() . This is because, in other languages, this often results in various ways to get an object’s length. E.g., some will call this function len , others will call it length, and yet someone else won’t even implement the function but simply offer a public member variable. And this is exactly the reason why Python chose to standardize the naming of such a common operation!
Counting element occurrence in a list
Don’t confuse the count function with getting the list length, it’s totally different. The built-in count function counts occurrences of a particular value inside that list. Here’s an example:
Since the number 1 occurs three times in the list, my_list.count(1) returned 3.
Check if an item is in a list
To check if an item is in a list, use the following syntax:
Find the index of an item in a list
We can find where an item is inside a list with the index method. For example, in the following list the 4 is located at position 3 (remember that we start counting at zero):
The index method takes two optional parameters: start and stop. With these, we can continue looking for more of the same values. We don’t need to supply an end value if we supply a start value. Now, let’s find both 4’s in the list below:
If you want to do more advanced filtering of lists, you should read my article on list comprehensions .
Loop over list elements
A list is iterable , so we can use a for-loop over the elements of a list just like we can with any other iterable with the ‘for <element> in <iterable>’ syntax:
Python list to string
In Python, you can convert most objects to a string with the str function:
If you’re interested, str is actually the Python string ‘s base class and calling str() constructs a new str object by calling the constructor of the str class. This constructor inspects the provided object and looks for a special method (also called dunder method) called __str__ . If it is present, this method is called. There’s nothing more to it.
If you create your own classes and objects , you can implement the __str__ function yourself to offer a printable version of your objects.
Sorting Python lists
To sort a Python list, we have two options:
- Use the built-in sort method of the list itself.
- Use Python’s built-in sorted() function.
Option one, the built-in method, offers an in-place sort, which means the list itself is modified. In other words, this function does not return anything. Instead, it modifies the list itself.
Option two returns a new list, leaving the original intact. Which one you use depends on the situation you’re in.
In-place list sort in ascending order
Let’s start with the simplest use-case: sorting in ascending order:
In-place list sort in descending order
We can call the sort method with a reverse parameter. If this is set to True, sort reverses the order:
Using sorted()
The following example demonstrates how to sort lists in ascending order, returning a new list with the result:
As you can see from the last statement, the original list is unchanged. Let’s do that again, but now in descending order:
Unsortable lists
We can not sort all lists, since Python can not compare all types with each other. For example, we can sort a list of numbers, like integers and floats, because they have a defined order. We can sort a list of strings as well since Python is able to compare strings too.
However, lists can contain any type of object, and we can’t compare completely different objects, like numbers and strings, to each other. In such cases, Python throws a TypeError :
Although the error might look a bit cryptic, it’s only logical when you know what’s going on. To sort a list, Python needs to compare the objects to each other. So in its sorting algorithm, at some point, it checks if ‘a’ < 1, hence the error: '<' not supported between instances of 'str' and 'int' .
Sometimes you need to get parts of a list. Python has a powerful syntax to do so, called slicing, and it makes working with lists a lot easier compared to some other programming languages. Slicing works on Python lists, but it also works on any other sequence type like strings , tuples , and ranges .
The slicing syntax is as follows:
my_list[start:stop:step]
A couple of notes:
- start is the first element position to include
- stop is exclusive, meaning that the element at position stop won’t be included.
- step is the step size. more on this later.
- start , stop , and step are all optional.
- Negative values can be used too.
To explain how slicing works, it’s best to just look at examples, and try for yourself, so that’s what we’ll do:
The step value
The step value is 1 by default. If you increase it, you can step over elements. Let’s try this:
Going backward
Like with list indexing, we can also supply negative numbers with slicing. Here’s a little ASCII art to show you how counting backward in a list works:
Just remember that you need to set a negative step size in order to go backward:
Reversing Python lists
There are actually three methods you can use to reverse a list in Python:
- An in-place reverse, using the built-in reverse method that every list has natively
- Using list slicing with a negative step size results in a new list
- Create a reverse iterator , with the reversed() function
In the following code crumb, I demonstrate all three. They are explained in detail in the following sections:
Using the built-in reverse method
The list.reverse() method does an in-place reverse, meaning it reorders the list. In other words, the method does not return a new list object, with a reversed order. Here’s how to use reverse() :
Reverse a list with list slicing
Although you can reverse a list with the list.reverse() method that every list has, you can do it with list slicing too, using a negative step size of -1. The difference here is that list slicing results in a new, second list. It keeps the original list intact:
Creating a reverse iterator
Finally, you can use the reversed() built-in function, which creates an iterator that returns all elements of the given iterable (our list) in reverse. This method is quite cheap in terms of CPU and memory usage. All it needs to do is walk backward over the iterable object. It’s doesn’t need to move around data and it doesn’t need to reserve extra memory for a second list. So if you need to iterate over a (large) list in reverse, this should be your choice.
Here’s how you can use this function. Keep in mind, that you can only use the iterator once, but that it’s cheap to make a new one:
Learn more about Python lists
Because there’s a lot to tell about list comprehensions, I created a dedicated article for the topic. A Python list comprehension is a language construct that we use to create a list based on an existing list, without creating for-loops .
Some other resources you might like:
- The official Python docs about lists.
- If you are interested in the internals: lists are often implemented internally as a linked list .
- Python also has arrays , which are very similar and the term will be familiar to people coming from other programming languages. They are more efficient at storing data, but they can only store one type of data.
Get certified with our courses
Learn Python properly through small, easy-to-digest lessons, progress tracking, quizzes to test your knowledge, and practice sessions. Each course will earn you a downloadable course certificate.
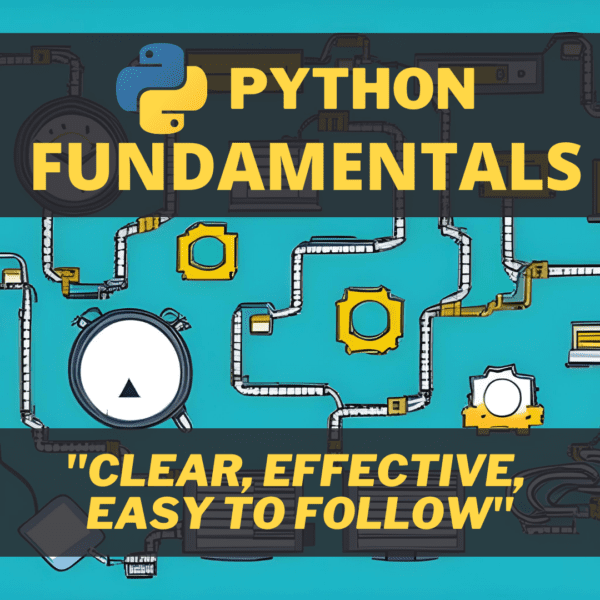
Related articles
- Python List Comprehension: Tutorial With Examples
- Python For Loop and While Loop
- Python Tuple: How to Create, Use, and Convert
- Pip Install: How To Install and Remove Python Packages
Python Newsletter
Before you leave, you may want to sign up for my newsletter.
Tips & Tricks News Course discounts
No spam & you can unsubscribe at any time.
- Google for Education
- Español – América Latina
- Português – Brasil
- Tiếng Việt
Python Lists
Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len() function and square brackets [ ] to access data, with the first element at index 0. (See the official python.org list docs .)

Assignment with an = on lists does not make a copy. Instead, assignment makes the two variables point to the one list in memory.

The "empty list" is just an empty pair of brackets [ ]. The '+' works to append two lists, so [1, 2] + [3, 4] yields [1, 2, 3, 4] (this is just like + with strings).
Python's *for* and *in* constructs are extremely useful, and the first use of them we'll see is with lists. The *for* construct -- for var in list -- is an easy way to look at each element in a list (or other collection). Do not add or remove from the list during iteration.
If you know what sort of thing is in the list, use a variable name in the loop that captures that information such as "num", or "name", or "url". Since Python code does not have other syntax to remind you of types, your variable names are a key way for you to keep straight what is going on. (This is a little misleading. As you gain more exposure to python, you'll see references to type hints which allow you to add typing information to your function definitions. Python doesn't use these type hints when it runs your programs. They are used by other programs such as IDEs (integrated development environments) and static analysis tools like linters/type checkers to validate if your functions are called with compatible arguments.)
The *in* construct on its own is an easy way to test if an element appears in a list (or other collection) -- value in collection -- tests if the value is in the collection, returning True/False.
The for/in constructs are very commonly used in Python code and work on data types other than list, so you should just memorize their syntax. You may have habits from other languages where you start manually iterating over a collection, where in Python you should just use for/in.
You can also use for/in to work on a string. The string acts like a list of its chars, so for ch in s: print(ch) prints all the chars in a string.
The range(n) function yields the numbers 0, 1, ... n-1, and range(a, b) returns a, a+1, ... b-1 -- up to but not including the last number. The combination of the for-loop and the range() function allow you to build a traditional numeric for loop:
There is a variant xrange() which avoids the cost of building the whole list for performance sensitive cases (in Python 3, range() will have the good performance behavior and you can forget about xrange()).
Python also has the standard while-loop, and the *break* and *continue* statements work as in C++ and Java, altering the course of the innermost loop. The above for/in loops solves the common case of iterating over every element in a list, but the while loop gives you total control over the index numbers. Here's a while loop which accesses every 3rd element in a list:
List Methods
Here are some other common list methods.
- list.append(elem) -- adds a single element to the end of the list. Common error: does not return the new list, just modifies the original.
- list.insert(index, elem) -- inserts the element at the given index, shifting elements to the right.
- list.extend(list2) adds the elements in list2 to the end of the list. Using + or += on a list is similar to using extend().
- list.index(elem) -- searches for the given element from the start of the list and returns its index. Throws a ValueError if the element does not appear (use "in" to check without a ValueError).
- list.remove(elem) -- searches for the first instance of the given element and removes it (throws ValueError if not present)
- list.sort() -- sorts the list in place (does not return it). (The sorted() function shown later is preferred.)
- list.reverse() -- reverses the list in place (does not return it)
- list.pop(index) -- removes and returns the element at the given index. Returns the rightmost element if index is omitted (roughly the opposite of append()).
Notice that these are *methods* on a list object, while len() is a function that takes the list (or string or whatever) as an argument.
Common error: note that the above methods do not *return* the modified list, they just modify the original list.
List Build Up
One common pattern is to start a list as the empty list [], then use append() or extend() to add elements to it:
List Slices
Slices work on lists just as with strings, and can also be used to change sub-parts of the list.
Exercise: list1.py
To practice the material in this section, try the problems in list1.py that do not use sorting (in the Basic Exercises ).
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-07-23 UTC.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
Python List
Python Tuple
- Python String
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python List pop()
Python List extend()
Python List insert()
- Python List append()
- Python List index()
- Python List remove()
There are many built-in types in Python that allow us to group and store multiple items. Python lists are the most versatile among them.
For example, we can use a Python list to store a playlist of songs so that we can easily add, remove, and update songs as needed.
- Create a Python List
We create a list by placing elements inside square brackets [] , separated by commas. For example,
Here, the ages list has three items.
We can store data of different data types in a Python list. For example,
We can use the built-in list() function to convert other iterables (strings, dictionaries, tuples, etc.) to a list.
List Characteristics
- Ordered - They maintain the order of elements.
- Mutable - Items can be changed after creation.
- Allow duplicates - They can contain duplicate values.
- Access List Elements
Each element in a list is associated with a number, known as an index .
The index of first item is 0 , the index of second item is 1 , and so on.

We use these index numbers to access list items. For example,

More on Accessing List Elements
Python also supports negative indexing. The index of the last element is -1 , the second-last element is -2 , and so on.
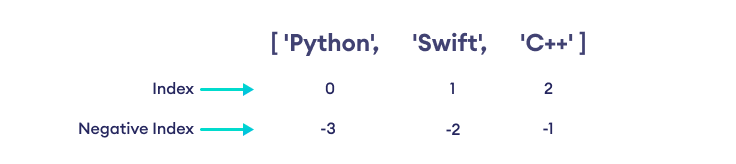
Negative indexing makes it easy to access list items from last.
Let's see an example,
In Python, it is possible to access a section of items from the list using the slicing operator : . For example,
To learn more about slicing, visit Python program to slice lists .
Note : If the specified index does not exist in a list, Python throws the IndexError exception.
- Add Elements to a Python List
We use the append() method to add an element to the end of a Python list. For example,
The insert() method adds an element at the specified index. For example,
We use the extend() method to add elements to a list from other iterables. For example,
- Change List Items
We can change the items of a list by assigning new values using the = operator. For example,
Here, we have replaced the element at index 2: 'Green' with 'Blue' .
- Remove an Item From a List
We can remove an item from a list using the remove() method. For example,
The del statement removes one or more items from a list. For example,
Note : We can also use the del statement to delete the entire list. For example,
- Python List Length
We can use the built-in len() function to find the number of elements in a list. For example,
- Iterating Through a List
We can use a for loop to iterate over the elements of a list. For example,
- Python List Methods
Python has many useful list methods that make it really easy to work with lists.
Method | Description |
---|---|
Adds an item to the end of the list | |
Adds items of lists and other iterables to the end of the list | |
Inserts an item at the specified index | |
Removes the specified value from the list | |
Returns and removes item present at the given index | |
Removes all items from the list | |
Returns the index of the first matched item | |
Returns the count of the specified item in the list | |
Sorts the list in ascending/descending order | |
Reverses the item of the list | |
Returns the shallow copy of the list |
More on Python Lists
List Comprehension is a concise and elegant way to create a list. For example,
To learn more, visit Python List Comprehension .
We use the in keyword to check if an item exists in the list. For example,
- orange is not present in fruits , so, 'orange' in fruits evaluates to False .
- cherry is present in fruits , so, 'cherry' in fruits evaluates to True .
Note: Lists are similar to arrays (or dynamic arrays) in other programming languages. When people refer to arrays in Python, they often mean lists, even though there is a numeric array type in Python.
- Python list()
Table of Contents
- Introduction
Before we wrap up, let’s put your knowledge of Python list to the test! Can you solve the following challenge?
Write a function to find the largest number in a list.
- For input [1, 2, 9, 4, 5] , the return value should be 9 .
Video: Python Lists and Tuples
Sorry about that.
Our premium learning platform, created with over a decade of experience and thousands of feedbacks .
Learn and improve your coding skills like never before.
- Interactive Courses
- Certificates
- 2000+ Challenges
Related Tutorials
Python Library
Python Tutorial
Python Programming
Python Lists
Updated on: April 9, 2021 | 35 Comments
Python list is an ordered sequence of items .
In this article you will learn the different methods of creating a list, adding, modifying, and deleting elements in the list. Also, learn how to iterate the list and access the elements in the list in detail. Nested Lists and List Comprehension are also discussed in detail with examples.
- Python List Exercise
- Python List Quiz
- Summary of List Operations
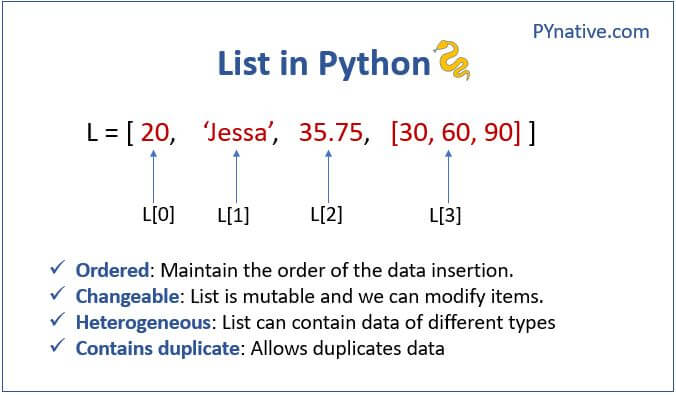
The following are the properties of a list .
- Mutable: The elements of the list can be modified. We can add or remove items to the list after it has been created.
- Ordered: The items in the lists are ordered. Each item has a unique index value. The new items will be added to the end of the list.
- Heterogenous : The list can contain different kinds of elements i.e; they can contain elements of string, integer, boolean, or any type.
- Duplicates: The list can contain duplicates i.e., lists can have two items with the same values.
Why use a list?
- The list data structure is very flexible It has many unique inbuilt functionalities like pop() , append() , etc which makes it easier, where the data keeps changing.
- Also, the list can contain duplicate elements i.e two or more items can have the same values.
- Lists are Heterogeneous i.e, different kinds of objects/elements can be added
- As Lists are mutable it is used in applications where the values of the items change frequently.
Table of contents
Creating a python list, length of a list, negative indexing, list slicing, iterate along with an index number, append item at the end of the list, add item at the specified position in the list, using extend(), modify all items, remove all occurrence of a specific item, remove item present at given index, remove the range of items, remove all items, finding an element in the list, concatenation of two lists.
- Using assignment operator (=)
Using the copy() method
Sort list using sort(), reverse a list using reverse(), using max() & min(), using sum(), nested list, list comprehension, summary of list operations.
The list can be created using either the list constructor or using square brackets [] .
- Using list() constructor: In general, the constructor of a class has its class name. Similarly, Create a list by passing the comma-separated values inside the list() .
- Using square bracket ( [] ) : In this method, we can create a list simply by enclosing the items inside the square brackets.
Let us see examples for creating the list using the above methods
In order to find the number of items present in a list, we can use the len() function.
Accessing items of a List
The items in a list can be accessed through indexing and slicing. This section will guide you by accessing the list using the following two ways
- Using indexing , we can access any item from a list using its index number
- Using slicing , we can access a range of items from a list
The list elements can be accessed using the “indexing” technique. Lists are ordered collections with unique indexes for each item. We can access the items in the list using this index number.
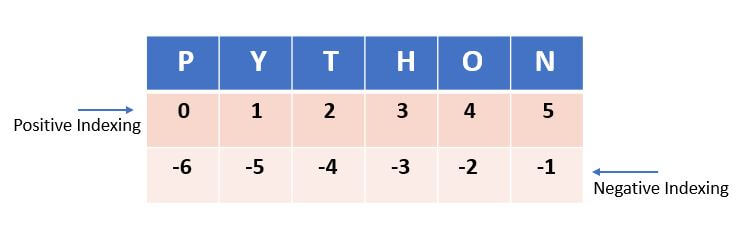
To access the elements in the list from left to right, the index value starts from zero to (length of the list-1) can be used. For example, if we want to access the 3rd element we need to use 2 since the index value starts from 0.
- As Lists are ordered sequences of items, the index values start from 0 to the Lists length.
- Whenever we try to access an item with an index more than the Lists length, it will throw the 'Index Error' .
- Similarly, the index values are always an integer. If we give any other type, then it will throw Type Error .
As seen in the above example we accessed the second element in the list by passing the index value as 1. Similarly, we passed index 4 to access the 5th element in the list.
The elements in the list can be accessed from right to left by using negative indexing . The negative value starts from -1 to -length of the list. It indicates that the list is indexed from the reverse/backward.
As seen in the above example to access the 4th element from the last (right to left) we pass ‘-4’ in the index value.
Slicing a list implies, accessing a range of elements in a list. For example, if we want to get the elements in the position from 3 to 7, we can use the slicing method. We can even modify the values in a range by using this slicing technique.
The below is the syntax for list slicing.
- The start_index denotes the index position from where the slicing should begin and the end_index parameter denotes the index positions till which the slicing should be done.
- The step allows you to take each nth-element within a start_index:end_index range.
Let us see few more examples of slicing a list such as
- Extract a portion of the list
- Reverse a list
- Slicing with a step
- Slice without specifying start or end position
Iterating a List
The objects in the list can be iterated over one by one, by using a for a loop.
The index value starts from 0 to (length of the list-1). Hence using the function range() is ideal for this scenario.
The range function returns a sequence of numbers. By default, it returns starting from 0 to the specified number (increments by 1). The starting and ending values can be passed according to our needs.
Adding elements to the list
We can add a new element/list of elements to the list using the list methods such as append() , insert() , and extend() .
The append() method will accept only one parameter and add it at the end of the list.
Let’s see the example to add the element ‘Emma’ at the end of the list.
Use the insert() method to add the object/item at the specified position in the list. The insert method accepts two parameters position and object.
It will insert the object in the specified index . Let us see this with an example.
As seen in the above example item 25 is added at the index position 2.
The extend method will accept the list of elements and add them at the end of the list. We can even add another list by using this method.
Let’s add three items at the end of the list.
As seen in the above example we have three integer values at once. All the values get added in the order they were passed and it gets appended at the end of the list.
Modify the items of a List
The list is a mutable sequence of iterable objects. It means we can modify the items of a list. Use the index number and assignment operator ( = ) to assign a new value to an item.
Let’s see how to perform the following two modification scenarios
- Modify the individual item.
- Modify the range of items
Use for loop to iterate and modify all items at once. Let’s see how to modify each item of a list.
Removing elements from a List
The elements from the list can be removed using the following list methods.
Description | |
---|---|
To remove the first occurrence of the item from the list. | |
Removes and returns the item at the given index from the list. | |
To remove all items from the list. The output will be an empty list. | |
Delete the entire list. |
Remove specific item
Use the remove() method to remove the first occurrence of the item from the list.
Note : It Throws a keyerror if an item not present in the original list.
Use a loop to remove all occurrence of a specific item
Use the pop() method to remove the item at the given index. The pop() method removes and returns the item present at the given index.
Note : It will remove the last time from the list if the index number is not passed.
Use del keyword along with list slicing to remove the range of items
Use the list’ clear() method to remove all items from the list. The clear() method truncates the list.
Use the index() function to find an item in a list.
The index() function will accept the value of the element as a parameter and returns the first occurrence of the element or returns ValueError if the element does not exist.
The concatenation of two lists means merging of two lists. There are two ways to do that.
- Using the + operator.
- Using the extend() method. The extend() method appends the new list’s items at the end of the calling list.
Copying a list
There are two ways by which a copy of a list can be created. Let us see each one with an example.
Using assignment operator ( = )
This is a straightforward way of creating a copy. In this method, the new list will be a deep copy. The changes that we make in the original list will be reflected in the new list.
This is called deep copying.
As seen in the above example a copy of the list has been created. The changes made to the original list are reflected in the copied list as well.
Note: When you set list1 = list2 , you are making them refer to the same list object, so when you modify one of them, all references associated with that object reflect the current state of the object. So don’t use the assignment operator to copy the dictionary instead use the copy() method.
The copy method can be used to create a copy of a list. This will create a new list and any changes made in the original list will not reflect in the new list. This is shallow copying .
As seen in the above example a copy of the list has been created. The changes made to the original list are not reflected in the copy.
List operations
We can perform some operations over the list by using certain functions like sort() , reverse() , clear() etc.
The sort function sorts the elements in the list in ascending order.
As seen in the above example the items are sorted in the ascending order.
The reverse function is used to reverse the elements in the list.
As seen in the above example the items in the list are printed in the reverse order here.

Python Built-in functions with List
In addition to the built-in methods available in the list, we can use the built-in functions as well on the list. Let us see a few of them for example.
The max function returns the maximum value in the list while the min function returns the minimum value in the list.
As seen in the above example the max function returns 6 and min function returns 1.
The sum function returns the sum of all the elements in the list.
As seen in the above example the sum function returns the sum of all the elements in the list.
In the case of all() function, the return value will be true only when all the values inside the list are true. Let us see the different item values and the return values.
Item Values in List | Return Value |
---|---|
All Values are True | True |
One or more False Values | False |
All False Values | False |
Empty List | True |
The any() method will return true if there is at least one true value. In the case of Empty List, it will return false. Let us see the same possible combination of values for any() function in a list and its return values.
Item Values in List | Return Value |
---|---|
All Values are True | True |
One or more False Values | True |
All False Values | False |
Empty List | False |
Similarly, let’s see each one of the above scenarios with a small example.
The list can contain another list (sub-list), which in turn contains another list and so on. This is termed a nested list.
In order to retrieve the elements of the inner list we need a nested For-Loop .
As we can see in the above output the indexing of the nested lists with the index value of the outer loop first followed by the inner list. We can print values of the inner lists through a nested for-loop .
List comprehension is a simpler method to create a list from an existing list. It is generally a list of iterables generated with an option to include only the items which satisfy a condition.
- expression : Optional. expression to compute the members of the output List which satisfies the optional conditions
- variable : Required. a variable that represents the members of the input List.
- inputList : Required. Represents the input set.
- condition1 , condition2 etc; : Optional. Filter conditions for the members of the output List.
As seen in the above example we have created a new list from an existing input list in a single statement. The new list now contains only the squares of the even numbers present in the input list.
We can even create a list when the input is a continuous range of numbers.
As seen in the above example we have created a list of squares of only even numbers in a range. The output is again a list so the items will be ordered.
For the following examples, we assume that l1 and l2 are lists, x , i , j , k , n are integers.
l1 = [10, 20, 30, 40, 50] and l2 = [60, 70, 80, 60]
Description | |
---|---|
Check if the list contains item . | |
Check if list does not contain item . | |
Concatenate the lists and . Creates a new list containing the items from and . | |
Repeat the list 5 times. | |
Get the item at index . Example is 30. | |
List slicing. Get the items from index up to index (excluding ) as a List. An example is | |
List slicing with step. Returns a List with the items from index up to index j taking every k-th item. An example is [10, 30]. | |
Returns a count of total items in a list. | |
Returns the number of times a particular item (60) appears in a list. The answer is 2. | |
Returns the index number of a particular item (30) in a list. The answer is 2. | |
Returns the index number of a particular item (30) in a list. But search Returns the item with maximum value from a list. The answer is 60 only from index number 2 to 5. | |
Returns the item with a minimum value from a list. The answer is 10. | |
Returns the item with maximum value from a list. The answer is 60. | |
Add item at the end of the list | |
Append the nested list at the end | |
Modify the item present at index 2 | |
Removes the first occurrence of item 40 from the list. | |
Removes and returns the item at index 2 from the list. | |
Make list empty | |
Copy into |
Did you find this page helpful? Let others know about it. Sharing helps me continue to create free Python resources.
About Vishal

I’m Vishal Hule , the Founder of PYnative.com. As a Python developer, I enjoy assisting students, developers, and learners. Follow me on Twitter .
Related Tutorial Topics:
Python exercises and quizzes.
Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more.
- 15+ Topic-specific Exercises and Quizzes
- Each Exercise contains 10 questions
- Each Quiz contains 12-15 MCQ
Loading comments... Please wait.
About PYnative
PYnative.com is for Python lovers. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills .
Explore Python
- Learn Python
- Python Basics
- Python Databases
- Python Exercises
- Python Quizzes
- Online Python Code Editor
- Python Tricks
To get New Python Tutorials, Exercises, and Quizzes
Legal Stuff
We use cookies to improve your experience. While using PYnative, you agree to have read and accepted our Terms Of Use , Cookie Policy , and Privacy Policy .
Copyright © 2018–2024 pynative.com
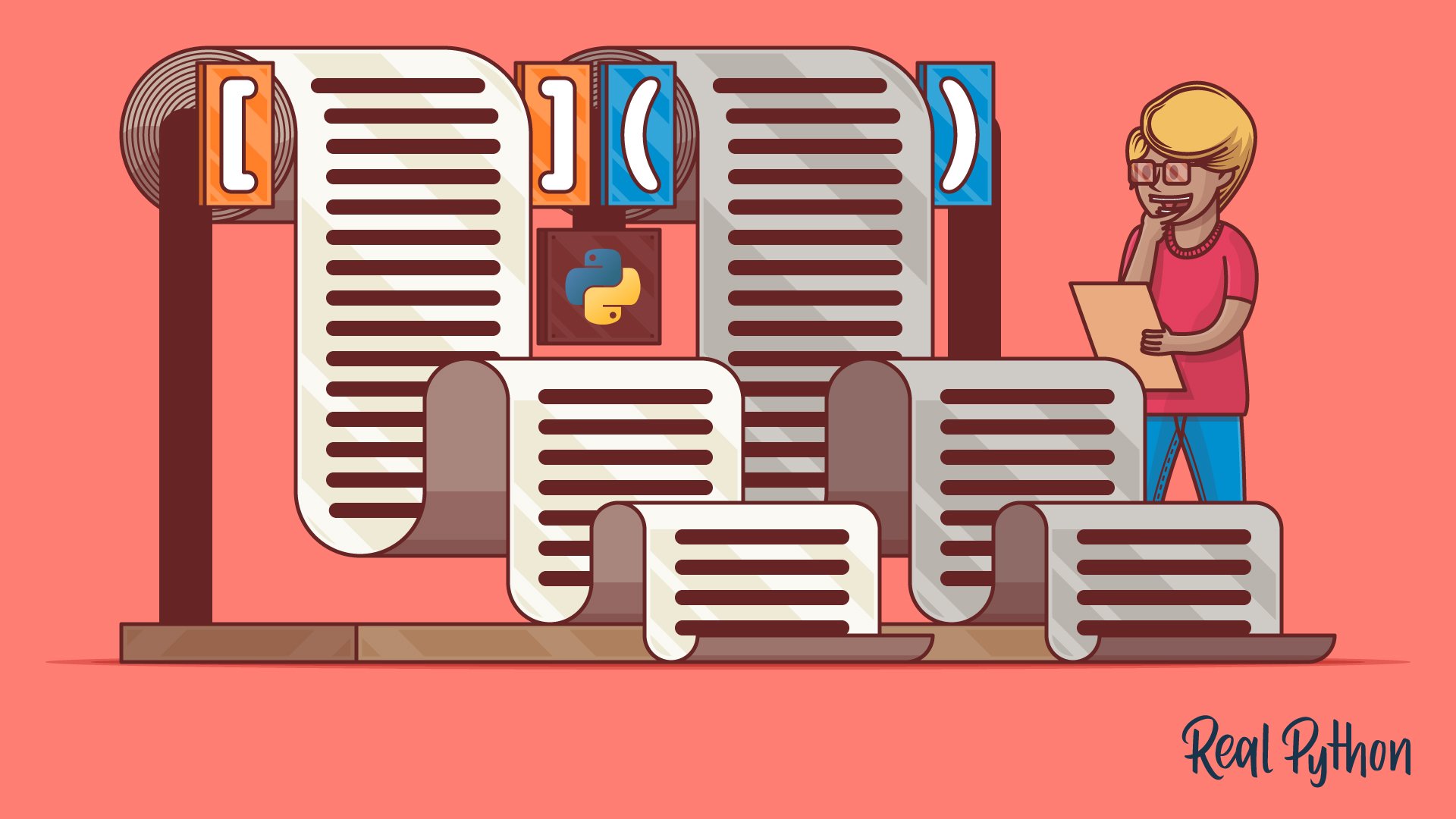
Lists vs Tuples in Python
Table of Contents
Creating Lists in Python
Creating tuples in python, lists and tuples are ordered sequences, lists and tuples can contain arbitrary objects, lists and tuples can be indexed and sliced, lists and tuples can be nested, lists are mutable, tuples are immutable, lists have mutator methods, tuples don’t, using operators and built-in functions with lists and tuples, packing and unpacking lists and tuples, using lists vs tuples in python.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: Lists and Tuples in Python
In Python, lists and tuples are versatile and useful data types that allow you to store data in a sequence. You’ll find them in virtually every nontrivial Python program. Learning about them is a core skill for you as a Python developer.
In this tutorial, you’ll:
- Get to know lists and tuples
- Explore the core characteristics of lists and tuples
- Learn how to define and manipulate lists and tuples
- Decide when to use lists or tuples in your code
To get the most out of this tutorial, you should know the basics of Python programming, including how to define variables.
Get Your Code: Click here to download the free sample code that shows you how to work with lists and tuples in Python.
Take the Quiz: Test your knowledge with our interactive “Lists vs Tuples in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Challenge yourself with this quiz to evaluate and deepen your understanding of Python lists and tuples. You'll explore key concepts, such as how to create, access, and manipulate these data types, while also learning best practices for using them efficiently in your code.
Getting Started With Python Lists and Tuples
In Python, a list is a collection of arbitrary objects, somewhat akin to an array in many other programming languages but more flexible. To define a list, you typically enclose a comma-separated sequence of objects in square brackets ( [] ), as shown below:
In this code snippet, you define a list of colors using string objects separated by commas and enclose them in square brackets.
Similarly, tuples are also collections of arbitrary objects. To define a tuple, you’ll enclose a comma-separated sequence of objects in parentheses ( () ), as shown below:
In this example, you define a tuple with data for a given person, including their name, age, job, and base country.
Up to this point, it may seem that lists and tuples are mostly the same. However, there’s an important difference:
Feature | List | Tuple |
---|---|---|
Is an ordered sequence | ✅ | ✅ |
Can contain arbitrary objects | ✅ | ✅ |
Can be indexed and sliced | ✅ | ✅ |
Can be nested | ✅ | ✅ |
Is mutable | ✅ | ❌ |
Both lists and tuples are sequence data types, which means they can contain objects arranged in order. You can access those objects using an integer index that represents their position in the sequence.
Even though both data types can contain arbitrary and heterogeneous objects, you’ll commonly use lists to store homogeneous objects and tuples to store heterogeneous objects.
Note: In this tutorial, you’ll see the terms homogeneous and heterogeneous used to express the following ideas:
- Homogeneous : Objects of the same data type or the same semantic meaning, like a series of animals, fruits, colors, and so on.
- Heterogeneous : Objects of different data types or different semantic meanings, like the attributes of a car: model, color, make, year, fuel type, and so on.
You can perform indexing and slicing operations on both lists and tuples. You can also have nested lists and nested tuples or a combination of them, like a list of tuples.
The most notable difference between lists and tuples is that lists are mutable, while tuples are immutable. This feature distinguishes them and drives their specific use cases.
Essentially, a list doesn’t have a fixed length since it’s mutable. Therefore, it’s natural to use homogeneous elements to have some structure in the list. A tuple, on the other hand, has a fixed length so the position of elements can have meaning, supporting heterogeneous data.
In many situations, you’ll define a list object using a literal . A list literal is a comma-separated sequence of objects enclosed in square brackets:
In this example, you create a list of countries represented by string objects. Because lists are ordered sequences, the values retain the insertion order.
Note: To learn more about the list data type, check out the Python’s list Data Type: A Deep Dive With Examples tutorial.
Alternatively, you can create new lists using the list() constructor :
In this example, you use the list() constructor to define a list of digits using a range object. In general, list() can convert any iterable to a list.
You can also create new list objects using list comprehensions . For example, the following comprehension builds a list of even digits:
List comprehensions are powerful tools for creating lists in Python. You’ll often find them in Python code that runs transformations over sequences of data.
Finally, to create an empty list, you can use either an empty pair of square brackets or the list() constructor without arguments:
The first approach is arguably the most efficient and most commonly used. However, the second approach can be more explicit and readable in some situations.
Similar to lists, you’ll often create new tuples using literals. Here’s a short example showing a tuple definition:
In this example, you create a tuple containing the parameters for a database connection. The data includes the server name, port, timeout, and database name.
Note: To dive deeper into the tuple data type, check out the Python’s tuple Data Type: A Deep Dive With Examples tutorial.
Strictly speaking, to define a tuple, you don’t need the parentheses. The comma-separated sequence will be enough:
In practice, you can define tuples without using a pair of parentheses. However, using the parentheses is a common practice because it improves the readability of your code.
Because the parentheses are optional, to define a single-item tuple, you need to use a comma:
In the first example, you create a tuple containing a single value by appending a comma after the value. In the second example, you use the parentheses without the comma. In this case, you create an integer value instead of a tuple.
You can also create new tuples using the tuple() constructor:
In this example, you create a list of digits using tuple() . This way of creating tuples can be helpful when you’re working with iterators and need to convert them into tuples.
Finally, to create empty tuples, you can use a pair of parentheses or call tuple() without arguments:
The first approach is a common way to create empty tuples. However, using tuple() can be more explicit and readable.
Exploring Core Features of Lists and Tuples
Now that you know the basics of creating lists and tuples in Python, you’re ready to explore their most relevant features and characteristics. In the following section, you’ll dive into these features and learn how they can impact the use cases of lists and tuples in your Python code.
List and tuples are ordered sequences of objects. The order in which you insert the objects when you create a list or tuple is an innate characteristic. This order remains the same for that list or tuple’s lifetime:
In these examples, you can confirm that the order of items in lists and tuples is the same order you define when creating the list or tuple.
Lists and tuples can contain any Python objects. The elements of a list or tuple can all be the same type:
In these examples, you create a list of integer numbers and then a tuple of similar objects. In both cases, the contained objects have the same data type. So, they’re homogeneous.
The elements of a list or tuple can also be of heterogeneous data types:
Here, your list and tuple contain objects of different types, including strings, integers, Booleans, and floats. So, your list and tuple are heterogeneous.
Note: Even though lists and tuples can contain heterogeneous or homogeneous objects, the common practice is to use lists for homogeneous objects and tuples for heterogeneous objects.
Lists and tuples can even contain objects like functions, classes, and modules:
In these examples, the list and the tuple contain a class, built-in function , custom function , and module objects.
Lists and tuples can contain any number of objects, from zero to as many as your computer’s memory allows. In the following code, you have a list and tuple built out of a range with a million numbers:
These two lines of code will take some time to run and populate your screen with many, many numbers.
Finally, objects in a list or tuple don’t need to be unique. A given object can appear multiple times:
Lists and tuples can contain duplicated values like "bark" in the above examples.
You can access individual elements in a list or tuple using the item’s index in square brackets. This is exactly analogous to accessing individual characters in a string. List indexing is zero-based, as it is with strings.
Consider the following list:
The indices for the elements in words are shown below:

Here’s the Python code to access individual elements of words :
The first element in the list has an index of 0 . The second element has an index of 1 , and so on. Virtually everything about indexing works the same for tuples.
You can also use a negative index, in which case the count starts from the end of the list:
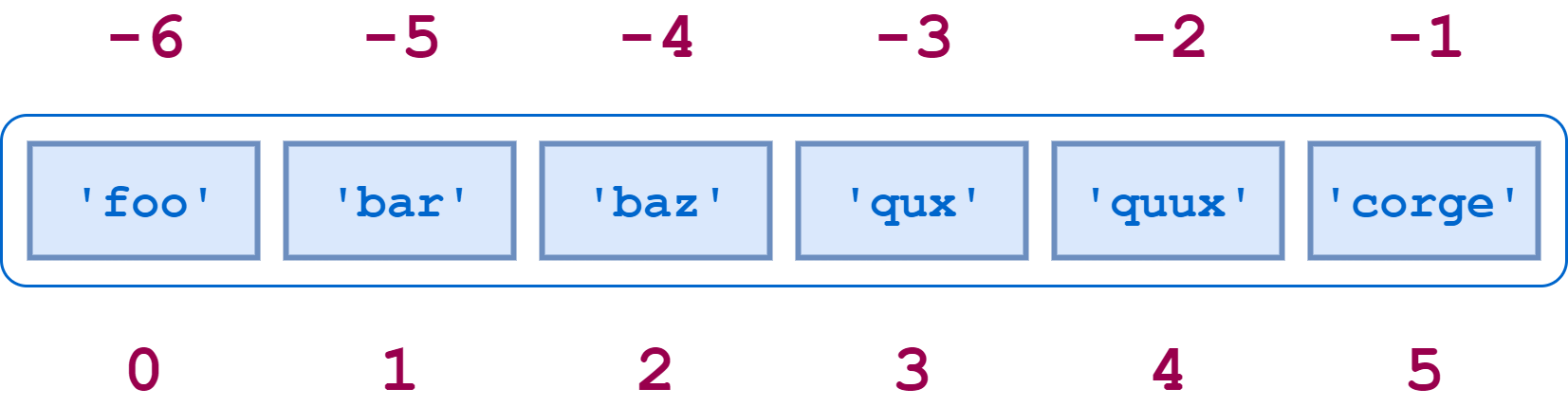
Index -1 corresponds to the last element in the list, while the first element is -len(words) , as shown below:
Slicing also works with lists and tuples. For example, the expression words[m:n] returns the portion of words from index m to, but not including, index n :
Other features of slicing work for lists as well. For example, you can use both positive and negative indices:
Omitting the first index starts the slice at the beginning of the list or tuple. Omitting the second index extends the slice to the end of the list or tuple:
You can specify a stride—either positive or negative:
The slicing operator ( [:] ) works for both lists and tuples. You can check it out by turning words into a tuple and running the same slicing operations on it.
You’ve seen that an element in a list or tuple can be of any type. This means that they can contain other lists or tuples. For example, a list can contain sublists, which can contain other sublists, and so on, to arbitrary depth.
Consider the following example:
The internal structure of this list is represented in the diagram below:
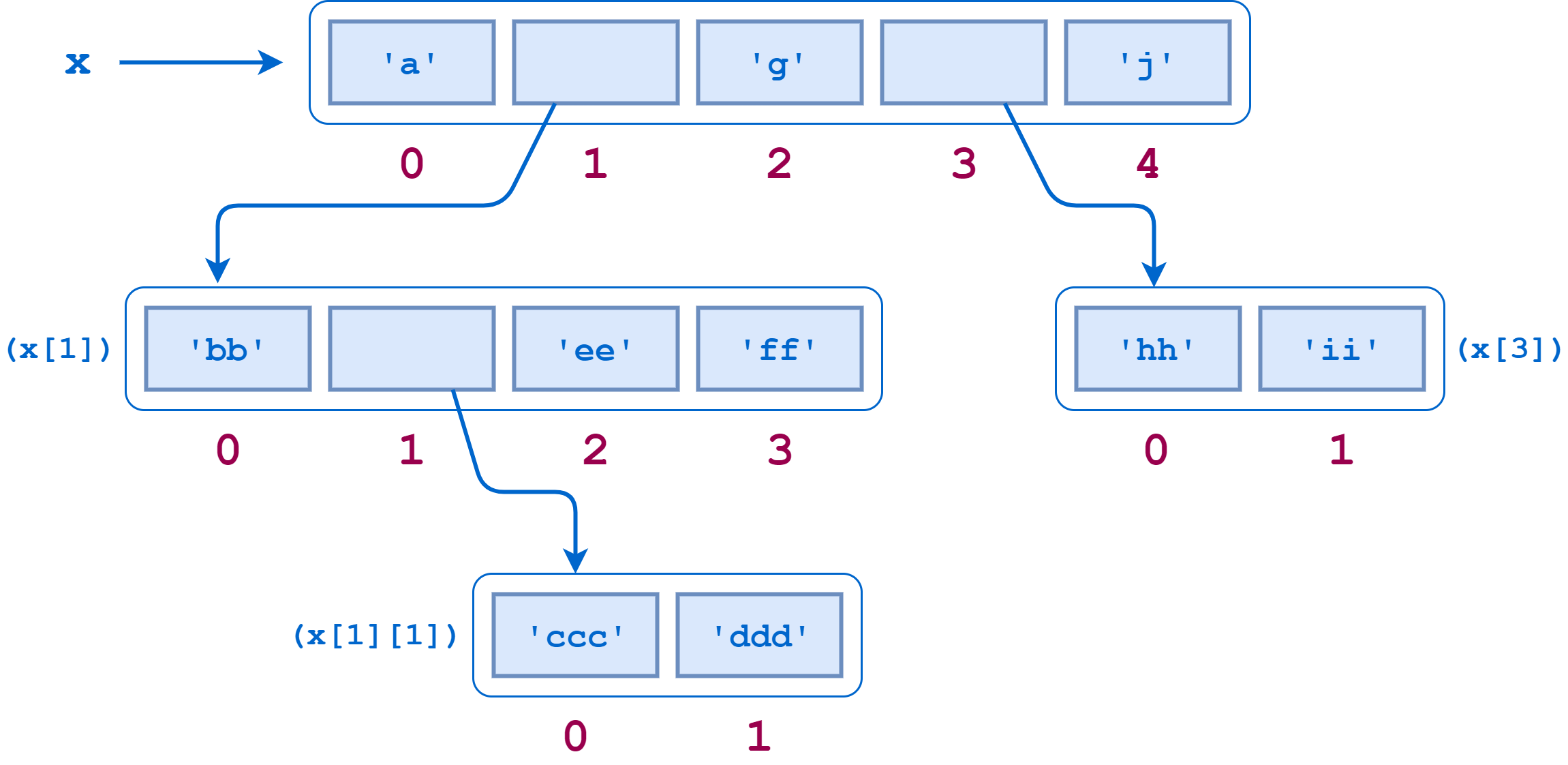
In this diagram, x[0] , x[2] , and x[4] are strings, each one character long:
However, x[1] and x[3] are sublists or nested lists:
To access the items in a sublist, append an additional index:
Here, x[1][1] is yet another sublist, so adding one more index accesses its elements:
There’s no limit to the depth you can nest lists this way. However, deeply nested lists or tuples can be hard to decipher in an indexing or slicing context.
The built-in list class provides a mutable data type. Being mutable means that once you create a list object, you can add, delete, shift, and move elements around at will. Python provides many ways to modify lists, as you’ll learn in a moment. Unlike lists, tuples are immutable , meaning that you can’t change a tuple once it has been created.
Note: To learn more about mutability and immutability in Python, check out the Python’s Mutable vs Immutable Types: What’s the Difference? tutorial.
You can replace or update a value in a list by indexing it on the left side of an assignment statement:
In this example, you create a list of letters where some letters are in uppercase while others are in lowercase. You use an assignment to turn the lowercase letters into uppercase letters.
Now, because tuples are immutable, you can’t do with a tuple what you did in the above example with a list:
If you try to update the value of a tuple element, you get a TypeError exception because tuples are immutable, and this type of operation isn’t allowed for them.
You can also use the del statement to delete individual items from a list. However, that operation won’t work on tuples:
You can remove individual elements from lists using the del statement because lists are mutable, but this won’t work with tuples because they’re immutable.
Note: To learn more about the del statement, check out the Python’s del : Remove References From Scopes and Containers tutorial.
What if you want to change several elements in a list at once? Python allows this operation with a slice assignment , which has the following syntax:
Think of an iterable as a container of multiple values like a list or tuple. This assignment replaces the specified slice of a_list with the content of <iterable> :
In this example, you replace the 0 values with the corresponding consecutive numbers using a slice assignment.
It’s important to note that the number of elements to insert doesn’t need to be equal to the number of elements in the slice. Python grows or shrinks the list as needed. For example, you can insert multiple elements in place of a single element:
In this example, you replace the 7 with a list of values from 4 to 7 . Note how Python automatically grows the list for you.
You can also insert elements into a list without removing anything. To do this, you can specify a slice of the form [n:n] at the desired index:
In this example, you insert the desired values at index 3 . Because you’re using an empty slice, Python doesn’t replace any of the existing values. Instead, it makes space for the new values as needed.
You can’t do slice assignment on tuple objects:
Because tuples are immutable, they don’t support slice assignment. If you try to do it, then you get a TypeError exception.
Python lists have several methods that you can use to modify the underlying list. These methods aren’t available for tuples because tuples are immutable, so you can’t change them in place.
In this section, you’ll explore the mutator methods available in Python list objects. These methods are handy in many situations, so they’re great tools for you as a Python developer.
.append(obj)
The .append(obj) method appends an object to the end of a list as a single item:
In this example, you append the letter "c" at the end of a using the .append() method, which modifies the list in place.
Note: List mutator methods modify the target list in place . They don’t return a new list:
In this code, you grab the return value of .append() in x . Using the print() function, you can uncover that the value is None instead of a new list object. While this behavior is deliberate to make it clear that the method mutates the object in place, it can be a common source of confusion when you’re starting to learn Python.
If you use an iterable as an argument to .append() , then that iterable is added as a single object:
This call to .append() adds the input list of letters as it is instead of appending three individual letters at the end of a . Therefore, the final list has three elements—the two initial strings and one list object. This may not be what you intended if you wanted to grow the list with the contents of the iterable.
.extend(iterable)
The .extend() method also adds items to the end of a list. However, the argument is expected to be an iterable like another list. The items in the input iterable are added as individual values:
The .extend() method behaves like the concatenation operator ( + ). More precisely, since it modifies the list in place, it behaves like the augmented concatenation operator ( += ). Here’s an example:
The augmented concatenation operator produces the same result as .extend() , adding individual items at the end of the target list.
.insert(index, obj)
The .insert() method inserts the input object into the target list at the position specified by index . Following the method call, a[<index>] is <obj> , and the remaining list elements are pushed to the right:
In this example, you insert the letter "b" between "a" and "c" using .insert() . Note that just like .append() , the .insert() method inserts the input object as a single element in the target list.
.remove(obj)
The .remove() method removes the input object from a list. If obj isn’t in the target list, then you get a ValueError exception:
With .remove() , you can delete specific objects from a given list. Note that this method removes only one instance of the input object. If the object is duplicated, then only its first instance will be deleted.
.pop([index=-1])
The .pop() method also allows you to remove items from a list. It differs from .remove() in two aspects:
- It takes the index of the object to remove rather than the object itself.
- It returns the value of the removed object.
Calling .pop() without arguments removes and returns the last item in the list:
If you specify the optional index argument, then the item at that index is removed and returned. Note that index can be negative too:
The index argument defaults to -1 , so a.pop(-1) is equivalent to a.pop() .
Several Python operators and built-in functions also work with lists and tuples. For example, the in and not in operators allow you to run membership tests on lists:
The in operator returns True if the target object is in the list and False otherwise. The not in operator produces the opposite result.
The concatenation ( + ) and repetition ( * ) operators also work with lists and tuples:
You can also use the built-in len() , min() , max() , and sum() functions with lists and tuples:
In this example, the len() function returns the number of values in the list. The min() and max() functions return the minimum and maximum values in the list, respectively. The sum() function returns the sum of the values in the input list.
Finally, it’s important to note that all these functions work the same with tuples. So, instead of using them with list objects, you can also use tuple objects.
A tuple literal can contain several items that you typically assign to a single variable or name:
When this occurs, it’s as though the items in the tuple have been packed into the object, as shown in the diagram below:
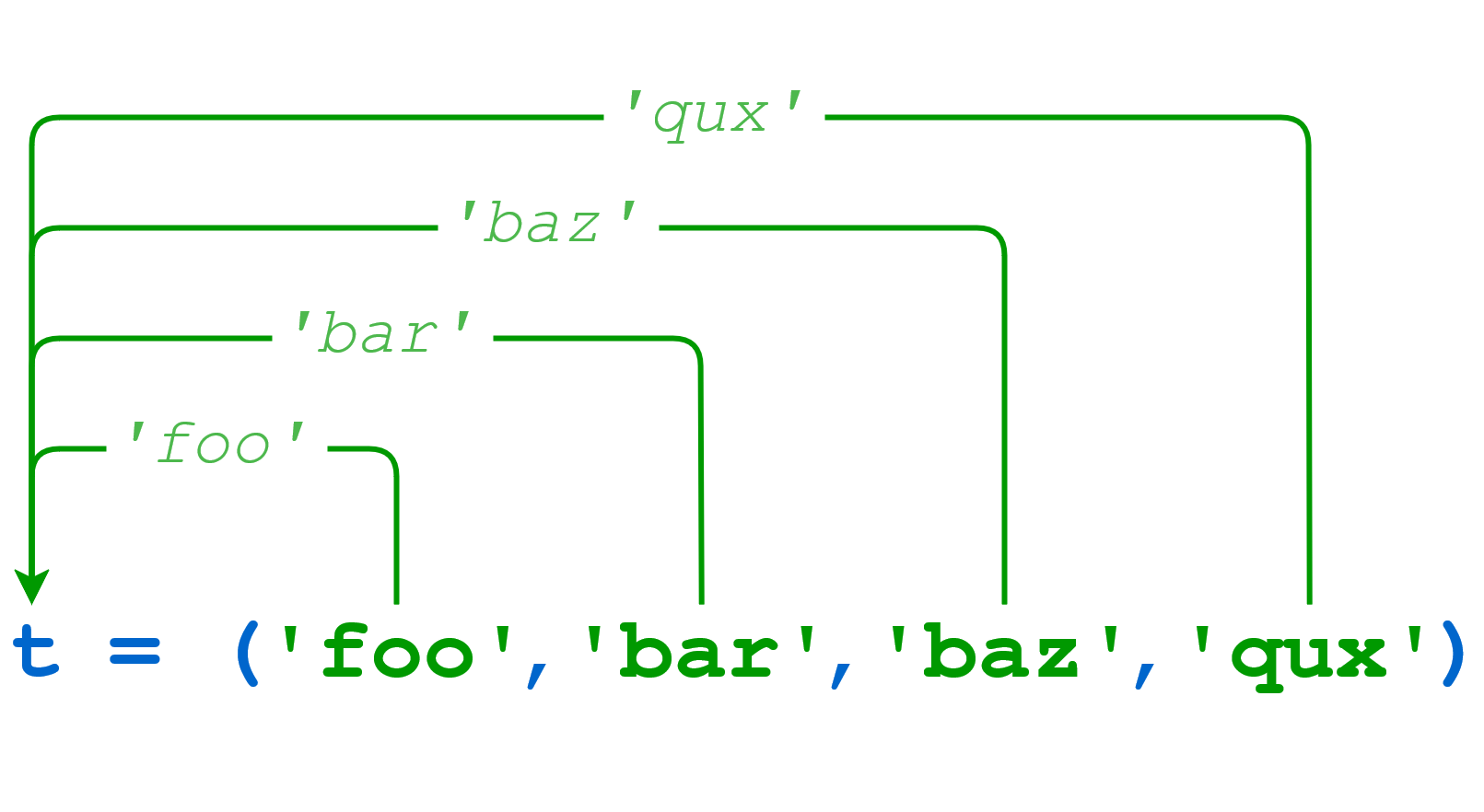
If the packed objects are assigned to a tuple of names, then the individual objects are unpacked as shown in the diagram below, where you use a tuple of s* variables:

Here’s how this unpacking works in Python code:
Note how each variable receives a single value from the unpacked tuple. When you’re unpacking a tuple, the number of variables on the left must match the number of values in the tuple. Otherwise, you get a ValueError exception:
In the first example, the number of variables is less than the items in the tuple, and the error message says that there are too many values to unpack. In the second example, the number of variables exceeds the number of items in the tuple. This time, the error message says that there aren’t enough values to unpack.
You can combine packing and unpacking in one statement to run a parallel assignment:
Again, the number of elements in the tuple on the left of the assignment must equal the number on the right. Otherwise, you get an error.
Tuple assignment allows for a curious bit of idiomatic Python. Sometimes, when programming, you have two variables whose values you need to swap. In most programming languages, it’s necessary to store one of the values in a temporary variable while the swap occurs.
Consider the following example that compares swapping with a temporary variable and unpacking:
Using a temporary variable to swap values can be annoying, so it’s great that you can do it with a single unpacking operation in Python. This feature also improves your code’s readability, making it more explicit.
Everything you’ve learned so far about lists and tuples can help you decide when to use a list or a tuple in your code. Here’s a summary of when it would be appropriate to use a list instead of a tuple:
- Mutable collections : When you need to add, remove, or change elements in the collection.
- Dynamic size : When the collection’s size might change during the code’s execution.
- Homogeneous data : When you need to store data of a homogeneous type or when the data represents a homogeneous concept.
Similarly, it’s appropriate to use a tuple rather than a list in the following situations:
- Immutable collections : When you have a fixed collection of items that shouldn’t change, such as coordinates (x, y, z), RGB color values, or other groupings of related values.
- Fixed size : When the collection’s size won’t change during the code’s execution.
- Heterogeneous data : When you need to store data of a heterogeneous type or when the data represents a heterogeneous concept.
- Function’s return values : When a function returns multiple values, you’ll typically use a tuple to pack these values together.
Finally, tuples can be more memory-efficient than lists, especially for large collections where immutability is acceptable or preferred. Similarly, if the integrity of the data is important and should be preserved throughout the program, tuples ensure and communicate that the data must remain unchanged.
Now you know the basic features of Python lists and tuples and understand how to manipulate them in your code. You’ll use these two data types extensively in your Python programming journey.
In this tutorial, you’ve:
- Learned about the built-in lists and tuples data types in Python
- Explored the core features of lists and tuples
- Discovered how to define and manipulate lists and tuples
- Learned when to use lists or tuples in your code
With this knowledge, you can now decide when it’s appropriate to use a list or tuple in your Python code. You also have the essential skills to create and manipulate lists and tuples in Python.
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
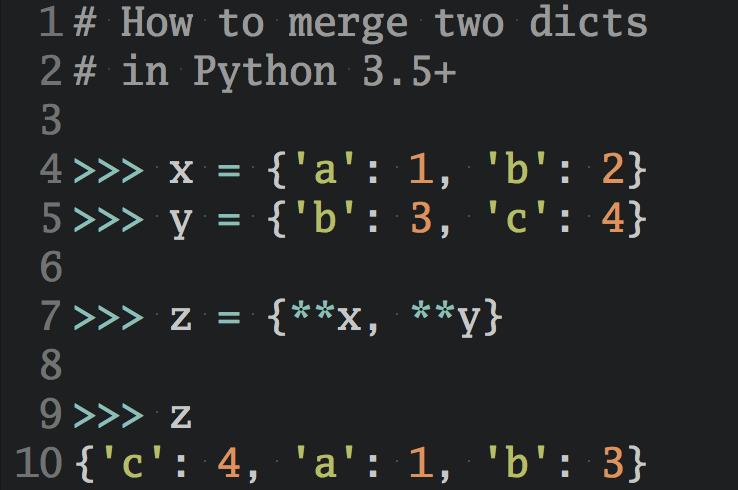
About Leodanis Pozo Ramos
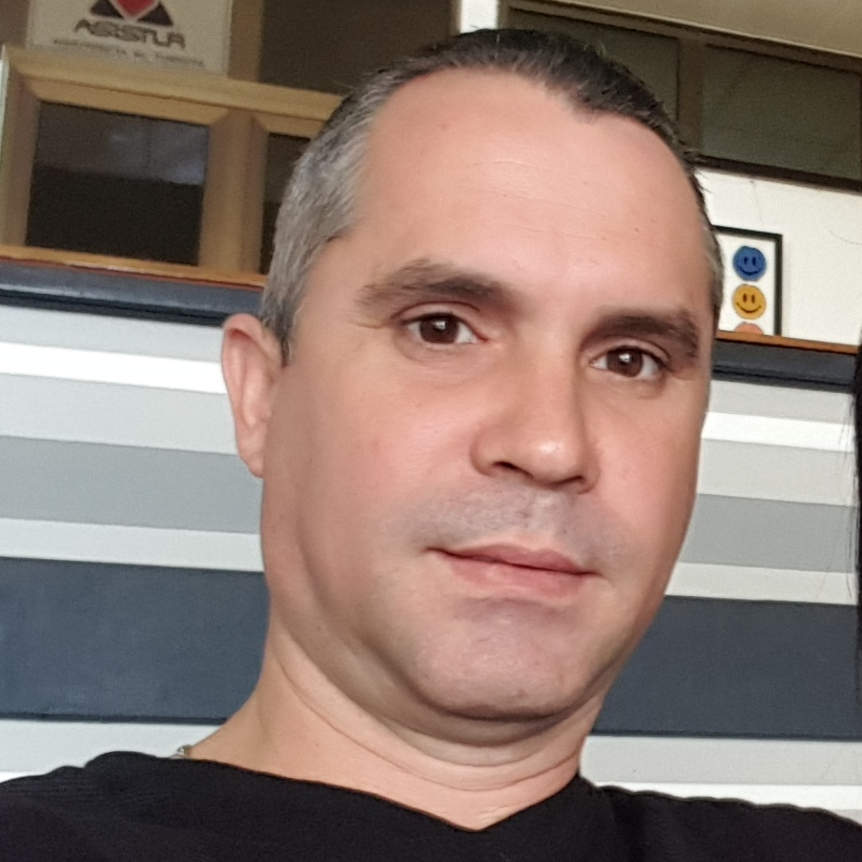
Leodanis is an industrial engineer who loves Python and software development. He's a self-taught Python developer with 6+ years of experience. He's an avid technical writer with a growing number of articles published on Real Python and other sites.
Each tutorial at Real Python is created by a team of developers so that it meets our high quality standards. The team members who worked on this tutorial are:
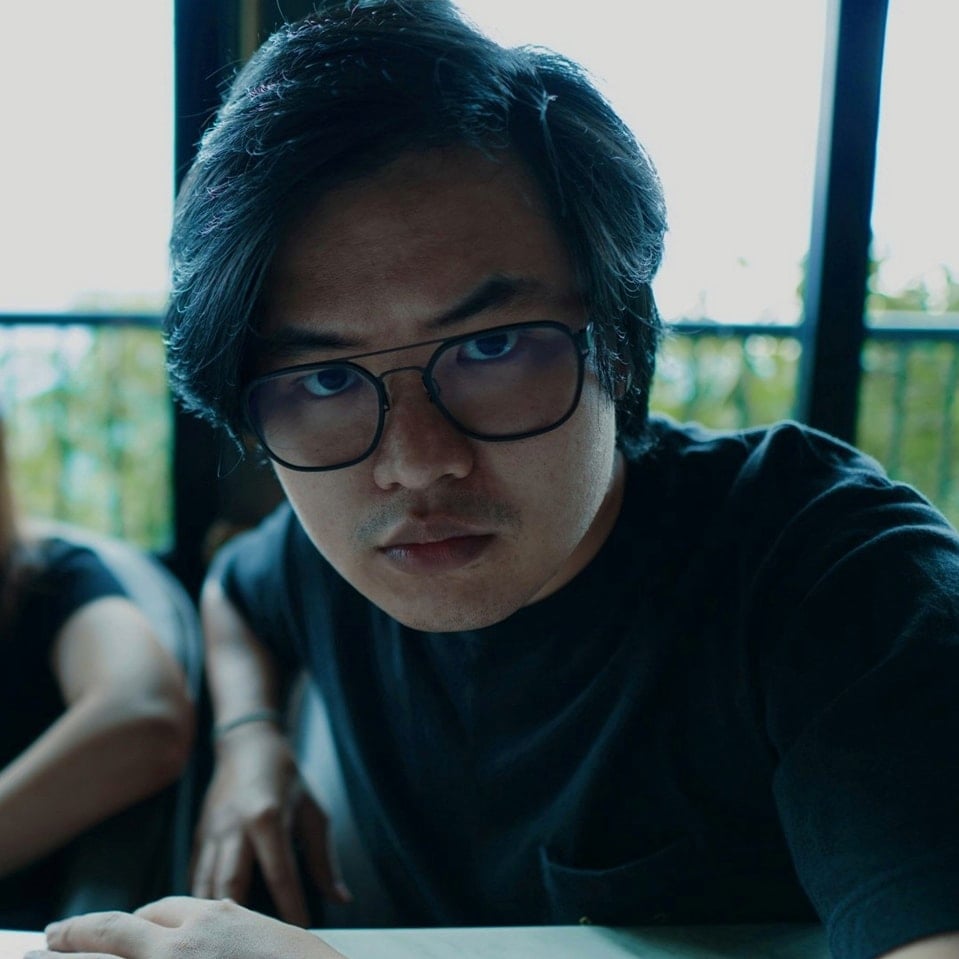
Master Real-World Python Skills With Unlimited Access to Real Python
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
Join us and get access to thousands of tutorials, hands-on video courses, and a community of expert Pythonistas:
What Do You Think?
What’s your #1 takeaway or favorite thing you learned? How are you going to put your newfound skills to use? Leave a comment below and let us know.
Commenting Tips: The most useful comments are those written with the goal of learning from or helping out other students. Get tips for asking good questions and get answers to common questions in our support portal . Looking for a real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session . Happy Pythoning!
Keep Learning
Related Topics: basics python
Recommended Video Course: Lists and Tuples in Python
Keep reading Real Python by creating a free account or signing in:
Already have an account? Sign-In
Almost there! Complete this form and click the button below to gain instant access:
Lists vs Tuples in Python (Sample Code)
🔒 No spam. We take your privacy seriously.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Operator | Example | Same As | Try it |
---|---|---|---|
= | x = 5 | x = 5 | |
+= | x += 3 | x = x + 3 | |
-= | x -= 3 | x = x - 3 | |
*= | x *= 3 | x = x * 3 | |
/= | x /= 3 | x = x / 3 | |
%= | x %= 3 | x = x % 3 | |
//= | x //= 3 | x = x // 3 | |
**= | x **= 3 | x = x ** 3 | |
&= | x &= 3 | x = x & 3 | |
|= | x |= 3 | x = x | 3 | |
^= | x ^= 3 | x = x ^ 3 | |
>>= | x >>= 3 | x = x >> 3 | |
<<= | x <<= 3 | x = x << 3 |
Related Pages

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Python Course
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Assignment Operators in Python
The Python Operators are used to perform operations on values and variables. These are the special symbols that carry out arithmetic, logical, and bitwise computations. The value the operator operates on is known as the Operand. Here, we will cover Different Assignment operators in Python .
Operators |
| ||
---|---|---|---|
| = | Assign the value of the right side of the expression to the left side operand | c = a + b |
| += | Add right side operand with left side operand and then assign the result to left operand | a += b |
| -= | Subtract right side operand from left side operand and then assign the result to left operand | a -= b |
| *= | Multiply right operand with left operand and then assign the result to the left operand | a *= b |
| /= | Divide left operand with right operand and then assign the result to the left operand | a /= b |
| %= | Divides the left operand with the right operand and then assign the remainder to the left operand | a %= b |
| //= | Divide left operand with right operand and then assign the value(floor) to left operand | a //= b |
| **= | Calculate exponent(raise power) value using operands and then assign the result to left operand | a **= b |
| &= | Performs Bitwise AND on operands and assign the result to left operand | a &= b |
| |= | Performs Bitwise OR on operands and assign the value to left operand | a |= b |
| ^= | Performs Bitwise XOR on operands and assign the value to left operand | a ^= b |
| >>= | Performs Bitwise right shift on operands and assign the result to left operand | a >>= b |
| <<= | Performs Bitwise left shift on operands and assign the result to left operand | a <<= b |
| := | Assign a value to a variable within an expression | a := exp |
Here are the Assignment Operators in Python with examples.
Assignment Operator
Assignment Operators are used to assign values to variables. This operator is used to assign the value of the right side of the expression to the left side operand.
Addition Assignment Operator
The Addition Assignment Operator is used to add the right-hand side operand with the left-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the addition assignment operator which will first perform the addition operation and then assign the result to the variable on the left-hand side.
S ubtraction Assignment Operator
The Subtraction Assignment Operator is used to subtract the right-hand side operand from the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the subtraction assignment operator which will first perform the subtraction operation and then assign the result to the variable on the left-hand side.
M ultiplication Assignment Operator
The Multiplication Assignment Operator is used to multiply the right-hand side operand with the left-hand side operand and then assigning the result to the left-hand side operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the multiplication assignment operator which will first perform the multiplication operation and then assign the result to the variable on the left-hand side.
D ivision Assignment Operator
The Division Assignment Operator is used to divide the left-hand side operand with the right-hand side operand and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the division assignment operator which will first perform the division operation and then assign the result to the variable on the left-hand side.
M odulus Assignment Operator
The Modulus Assignment Operator is used to take the modulus, that is, it first divides the operands and then takes the remainder and assigns it to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the modulus assignment operator which will first perform the modulus operation and then assign the result to the variable on the left-hand side.
F loor Division Assignment Operator
The Floor Division Assignment Operator is used to divide the left operand with the right operand and then assigs the result(floor value) to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the floor division assignment operator which will first perform the floor division operation and then assign the result to the variable on the left-hand side.
Exponentiation Assignment Operator
The Exponentiation Assignment Operator is used to calculate the exponent(raise power) value using operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the exponentiation assignment operator which will first perform exponent operation and then assign the result to the variable on the left-hand side.
Bitwise AND Assignment Operator
The Bitwise AND Assignment Operator is used to perform Bitwise AND operation on both operands and then assigning the result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise AND assignment operator which will first perform Bitwise AND operation and then assign the result to the variable on the left-hand side.
Bitwise OR Assignment Operator
The Bitwise OR Assignment Operator is used to perform Bitwise OR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise OR assignment operator which will first perform bitwise OR operation and then assign the result to the variable on the left-hand side.
Bitwise XOR Assignment Operator
The Bitwise XOR Assignment Operator is used to perform Bitwise XOR operation on the operands and then assigning result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise XOR assignment operator which will first perform bitwise XOR operation and then assign the result to the variable on the left-hand side.
Bitwise Right Shift Assignment Operator
The Bitwise Right Shift Assignment Operator is used to perform Bitwise Right Shift Operation on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise right shift assignment operator which will first perform bitwise right shift operation and then assign the result to the variable on the left-hand side.
Bitwise Left Shift Assignment Operator
The Bitwise Left Shift Assignment Operator is used to perform Bitwise Left Shift Opertator on the operands and then assign result to the left operand.
Example: In this code we have two variables ‘a’ and ‘b’ and assigned them with some integer value. Then we have used the bitwise left shift assignment operator which will first perform bitwise left shift operation and then assign the result to the variable on the left-hand side.
Walrus Operator
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression.
Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop . The operator will solve the expression on the right-hand side and assign the value to the left-hand side operand ‘x’ and then execute the remaining code.
Assignment Operators in Python – FAQs
What are assignment operators in python.
Assignment operators in Python are used to assign values to variables. These operators can also perform additional operations during the assignment. The basic assignment operator is = , which simply assigns the value of the right-hand operand to the left-hand operand. Other common assignment operators include += , -= , *= , /= , %= , and more, which perform an operation on the variable and then assign the result back to the variable.
What is the := Operator in Python?
The := operator, introduced in Python 3.8, is known as the “walrus operator”. It is an assignment expression, which means that it assigns values to variables as part of a larger expression. Its main benefit is that it allows you to assign values to variables within expressions, including within conditions of loops and if statements, thereby reducing the need for additional lines of code. Here’s an example: # Example of using the walrus operator in a while loop while (n := int(input("Enter a number (0 to stop): "))) != 0: print(f"You entered: {n}") This loop continues to prompt the user for input and immediately uses that input in both the condition check and the loop body.
What is the Assignment Operator in Structure?
In programming languages that use structures (like C or C++), the assignment operator = is used to copy values from one structure variable to another. Each member of the structure is copied from the source structure to the destination structure. Python, however, does not have a built-in concept of ‘structures’ as in C or C++; instead, similar functionality is achieved through classes or dictionaries.
What is the Assignment Operator in Python Dictionary?
In Python dictionaries, the assignment operator = is used to assign a new key-value pair to the dictionary or update the value of an existing key. Here’s how you might use it: my_dict = {} # Create an empty dictionary my_dict['key1'] = 'value1' # Assign a new key-value pair my_dict['key1'] = 'updated value' # Update the value of an existing key print(my_dict) # Output: {'key1': 'updated value'}
What is += and -= in Python?
The += and -= operators in Python are compound assignment operators. += adds the right-hand operand to the left-hand operand and assigns the result to the left-hand operand. Conversely, -= subtracts the right-hand operand from the left-hand operand and assigns the result to the left-hand operand. Here are examples of both: # Example of using += a = 5 a += 3 # Equivalent to a = a + 3 print(a) # Output: 8 # Example of using -= b = 10 b -= 4 # Equivalent to b = b - 4 print(b) # Output: 6 These operators make code more concise and are commonly used in loops and iterative data processing.
Please Login to comment...
Similar reads.
- Python-Operators
- Top 10 Fun ESL Games and Activities for Teaching Kids English Abroad in 2024
- Top Free Voice Changers for Multiplayer Games and Chat in 2024
- Best Monitors for MacBook Pro and MacBook Air in 2024
- 10 Best Laptop Brands in 2024
- 15 Most Important Aptitude Topics For Placements [2024]
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Python »
- 3.12.5 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |
7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment statement like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
The assignment target is considered “simple” if it consists of a single name that is not enclosed in parentheses. For simple assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
If the assignment target is not simple (an attribute, subscript node, or parenthesized name), the annotation is evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
Added in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
What does colon at assignment for list[:] = [...] do in Python [duplicate]
I came accross the following code:
What does nums[:] = do that nums = does not? For that matter, what does nums[:] do that nums does not?

- Asked and answered I believe. What does [:] in Python mean – CollinD Commented Sep 8, 2015 at 2:38
- 1 @CollinD Didn't see that question, thanks. But still doesn't answer the assignment question – sinθ Commented Sep 8, 2015 at 2:39
- I had voted to reopen because I didn't think the linked duplicate explained slice assignment. I must have opened the wrong link, because it definitely does explain slice assignment. – Josh Smeaton Commented Sep 8, 2015 at 3:30
2 Answers 2
This syntax is a slice assignment. A slice of [:] means the entire list. The difference between nums[:] = and nums = is that the latter doesn't replace elements in the original list. This is observable when there are two references to the list
To see the difference just remove the [:] from the assignment above.
Note: vincent thorpe's comments below are either incorrect or irrelevant to the question. This is not a matter of value vs reference semantics, nor whether you apply the operator to an lvalue or rvalue.

- Simply say, (list[:] = ...) is value type but (list = ...) is reference type – Brady Huang Commented Feb 6, 2018 at 10:01
- 2 @Brady Huang that doesn't describe this accurately – Ryan Haining Commented Feb 6, 2018 at 17:11
- 1 you are saying that slice notation list[:] means the reference, while the list means the copy? then why in for in list: if you pass the list itself and you insert some element inside the loop, this loop will never end because since its incrementing its length it will be forever. it means if yo'u pass list without[:] you are passing the reference. that's contradictory huh – vincent thorpe Commented Jul 18, 2019 at 8:29
- 1 the [:] operator if you put Lvalue or Rvalue it means different – vincent thorpe Commented Jul 18, 2019 at 10:33
- 4 @vincentthorpe lst[:] not followed by an = calls __getitem__ , while lst[:] = calls __setitem__ , so [] and []= are best thought of as two different operators – Ryan Haining Commented Jul 18, 2019 at 16:50
nums = foo rebinds the name nums to refer to the same object that foo refers to.
nums[:] = foo invokes slice assignment on the object that nums refers to, thus making the contents of the original object a copy of the contents of foo .
- Better answer in my opinion – YoarkYANG Commented Oct 15, 2021 at 2:28
- " ...rebinds... " indeed, +1 – Glenn Slayden Commented Dec 27, 2021 at 11:37
- Best answer indeed. The example is a little weird though, since you never print "c". – Manu Torres Commented Oct 24, 2022 at 14:38
Not the answer you're looking for? Browse other questions tagged python python-3.x or ask your own question .
- The Overflow Blog
- Best practices for cost-efficient Kafka clusters
- The hidden cost of speed
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- What's the difference? lie down vs lie
- Does the USA plan to establish a military facility on Saint Martin's Island in the Bay of Bengal?
- What is the translation of a code monkey in French?
- Is it a good idea to perform I2C Communication in the ISR?
- Short story about humanoid creatures living on ice, which can swim under the ice and eat the moss/plants that grow on the underside of the ice
- In roulette, is the frequency of getting long sequences of reds lower than that of shorter sequences?
- Applying for different jobs finding out it is for the same project between different companies
- How do I learn more about rocketry?
- Can Christian Saudi Nationals visit Mecca?
- Short story - disease that causes deformities and telepathy. Bar joke
- Why is there so much salt in cheese?
- Is missing SAN in certificate a security issue?
- You find yourself locked in a room
- An instructor is being added to co-teach a course for questionable reasons, against the course author's wishes—what can be done?
- Are all citizens of Saudi Arabia "considered Muslims by the state"?
- Was the term " these little ones" used as a code word for believers?
- Largest number possible with +, -, ÷
- What is an overview of utilitarian arguments in support of exclusive relationships?
- Stained passport am I screwed
- How best to cut (slightly) varying size notches in long piece of trim
- Current in a circuit is 50% lower than predicted by Kirchhoff's law
- Microsoft SQL In-Memory OLTP in SQL Express 2019/2022
- How to change upward facing track lights 26 feet above living room?
- How to load a function from a Vim9 script and call it?

IMAGES
VIDEO
COMMENTS
The Python language has distinct concepts for expressions and statements. Assignment is a statement even if the syntax sometimes tricks you into thinking it's an expression (e.g. a=b=99 works but is a special syntax case and doesn't mean that the b=99 is an expression like it is for example in C). List comprehensions are instead expressions because they return a value, in a sense the loop they ...
Python's list is a flexible, versatile, powerful, and popular built-in data type. It allows you to create variable-length and mutable sequences of objects. In a list, you can store objects of any type. You can also mix objects of different types within the same list, although list elements often share the same type.
List. Lists are used to store multiple items in a single variable. Lists are one of 4 built-in data types in Python used to store collections of data, the other 3 are Tuple, Set, and Dictionary, all with different qualities and usage. Lists are created using square brackets:
2. list[:] specifies a range within the list, in this case it defines the complete range of the list, i.e. the whole list and changes them. list=range(100), on the other hand, kind of wipes out the original contents of list and sets the new contents. But try the following:
Exercise 1: Reverse a list in Python. Exercise 2: Concatenate two lists index-wise. Exercise 3: Turn every item of a list into its square. Exercise 4: Concatenate two lists in the following order. Exercise 5: Iterate both lists simultaneously. Exercise 6: Remove empty strings from the list of strings.
Implicit Assignments in Python. Python implicitly runs assignments in many different contexts. In most cases, these implicit assignments are part of the language syntax. In other cases, they support specific behaviors. Whenever you complete an action in the following list, Python runs an implicit assignment for you: Define or call a function
Using the list() function. Python lists, like all Python data types, are objects. The class of a list is called 'list', with a lower case L. If you want to convert another Python object to a list, you can use the list() function, which is actually the constructor of the list class itself. This function takes one argument: an object that is ...
Python has a great built-in list type named "list". List literals are written within square brackets [ ]. Lists work similarly to strings -- use the len() function and square brackets [ ] to access data, with the first element at index 0. ... Assignment with an = on lists does not make a copy. Instead, assignment makes the two variables point ...
Python lists store multiple data together in a single variable. In this tutorial, we will learn about Python lists (creating lists, changing list items, removing items, and other list operations) with the help of examples.
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
Creating a Python list. The list can be created using either the list constructor or using square brackets [].. Using list() constructor: In general, the constructor of a class has its class name.Similarly, Create a list by passing the comma-separated values inside the list().; Using square bracket ([]): In this method, we can create a list simply by enclosing the items inside the square brackets.
Python Lists are just like dynamically sized arrays, declared in other languages (vector in C++ and ArrayList in Java). In simple language, a list is a collection of things, enclosed in [ ] and separated by commas. The list is a sequence data type which is used to store the collection of data.
The list data type has some more methods. Here are all of the methods of list objects: list. append (x) Add an item to the end of the list. Equivalent to a[len(a):] = [x]. list. extend (iterable) Extend the list by appending all the items from the iterable. Equivalent to a[len(a):] = iterable. list. insert (i, x) Insert an item at a given position.
The built-in list class provides a mutable data type. Being mutable means that once you create a list object, you can add, delete, shift, and move elements around at will. Python provides many ways to modify lists, as you'll learn in a moment. Unlike lists, tuples are immutable, meaning that you can't change a tuple once it has been created.
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
The Walrus Operator in Python is a new assignment operator which is introduced in Python version 3.8 and higher. This operator is used to assign a value to a variable within an expression. Syntax: a := expression. Example: In this code, we have a Python list of integers. We have used Python Walrus assignment operator within the Python while loop.
As you have written: list1=[1,2,3,4] list2=list1. The above code snippet will map list1 to list2. To check whether two variables refer to the same object, you can use is operator. >>> list1 is list2. # will return "True". In your example, Python created one list, reference by list1 & list2. So there are two references to the same object.
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right. Assignment is defined recursively depending on the form of the target (list).
Nested python list assignment. 50. Python: list of lists. 2. Assigning a list to another list in a list of lists in Python. 2. Python, List of Lists. 1. Python list assignation. 0. Assigning lists to eachother in Python. 2. List assignment in python. Hot Network Questions How to remove a file which name seems to be "." on an SMB share?
74. This syntax is a slice assignment. A slice of [:] means the entire list. The difference between nums[:] = and nums = is that the latter doesn't replace elements in the original list. This is observable when there are two references to the list. # original and other refer to. To see the difference just remove the [:] from the assignment above.