- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.

Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

Assignment Statement
The assignment statement is an instruction that stores a value in a variable . You use this instruction any time you want to update the value of a variable.
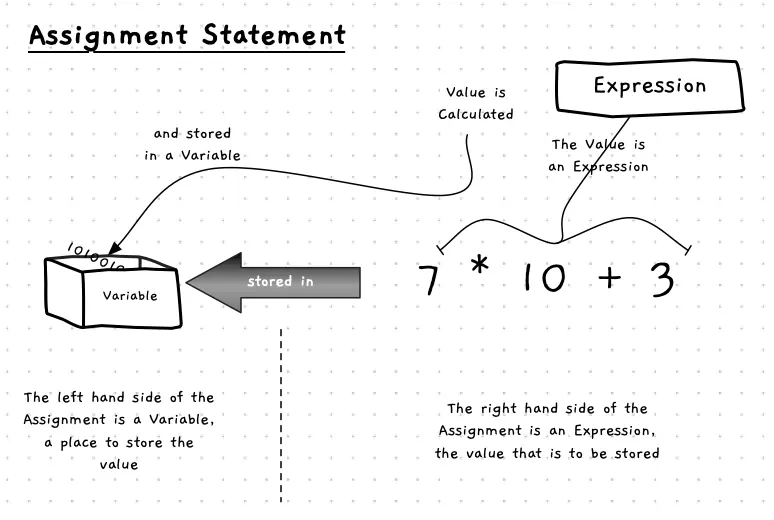
The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ). Once it has the value, it stores the value (assigns it) to the variable on the left-hand side of the assignment operator.
Assignment Statement — when, why, and how
When you create a variable, you have identified a piece of information that you want to be able to change as your program runs. Whenever you need to give a variable an initial or new value, you use an assignment statement .
The assignment statement uses the assignment operator = . Whatever is on the right-hand side of = represents the value to be assigned. This could be a literal , a method call , or any other expression . On the left-hand side you write the identifier of the variable you want to store this value in.
For example, you might decide to ask the user for their name. First, you need a variable to store the value. You might decide to call this variable name . Then, the assignment statement lets you read a response from the user and store it in that variable. In this case, the right-hand side of the assignment would be a call to ReadLine , which reads input from standard in and returns it to you. The left-hand side would be the identifier of our variable, name .
It is important to remember that every assignment statement has 2 actions :
- Calculate the value on the right-hand side
- Store it in the variable on the left-hand side.
The ordering of these actions allow you to update the value of a variable using an expression involving the variable being updated. This can be very useful. For example, you might want to update the value of a variable storing the number of steps you have taken today.
In C# the assignment operator is = . Most assignment statements are written using = , with an identifier on the left-hand side and an expression on the right-hand side. The assignment operator can optionally be modified with + , - , * , or / , which are shorthands for adding to, subtracting from, multiplying, and dividing the variable identified on the left-hand side of the statement.
Some assignment statements are written without = . These are assignment statements using increment ( ++ ) or decrement ( -- ), which allow you to add or remove one from a variable’s current value.
For example, x = x - 1 , x -= 1 , and x-- are all assignment statements which do the same thing — assign the variable x a new value that is one lower than its current value.
Basic assignment statement
In this example we use ReadLine to get input from the user and store it in a name variable.
Shorthand assignment statements
The following code shows an example of how to use some of the shorthand assignment statements.
If you ran the above code and entered 17 as the start count you should get this output:
You do not always need to store values in variables. Sometimes you can just use the value and then forget it. For example, in the above code, we read the initial count from the user. This requires us to read it as text, and then convert that text to a number. Given that we do not ever use the details in line again, we do not need to create this variable in the first place. Instead, we could pass the value to the convert function directly as shown below.
Assignment statement up close
The following sliders show how the assignment statement works in detail. These are both relatively simple programs, but notice how much is going on behind the scenes!
Assigning an int division result to an int variable
Assigning an int division result to a double variable.
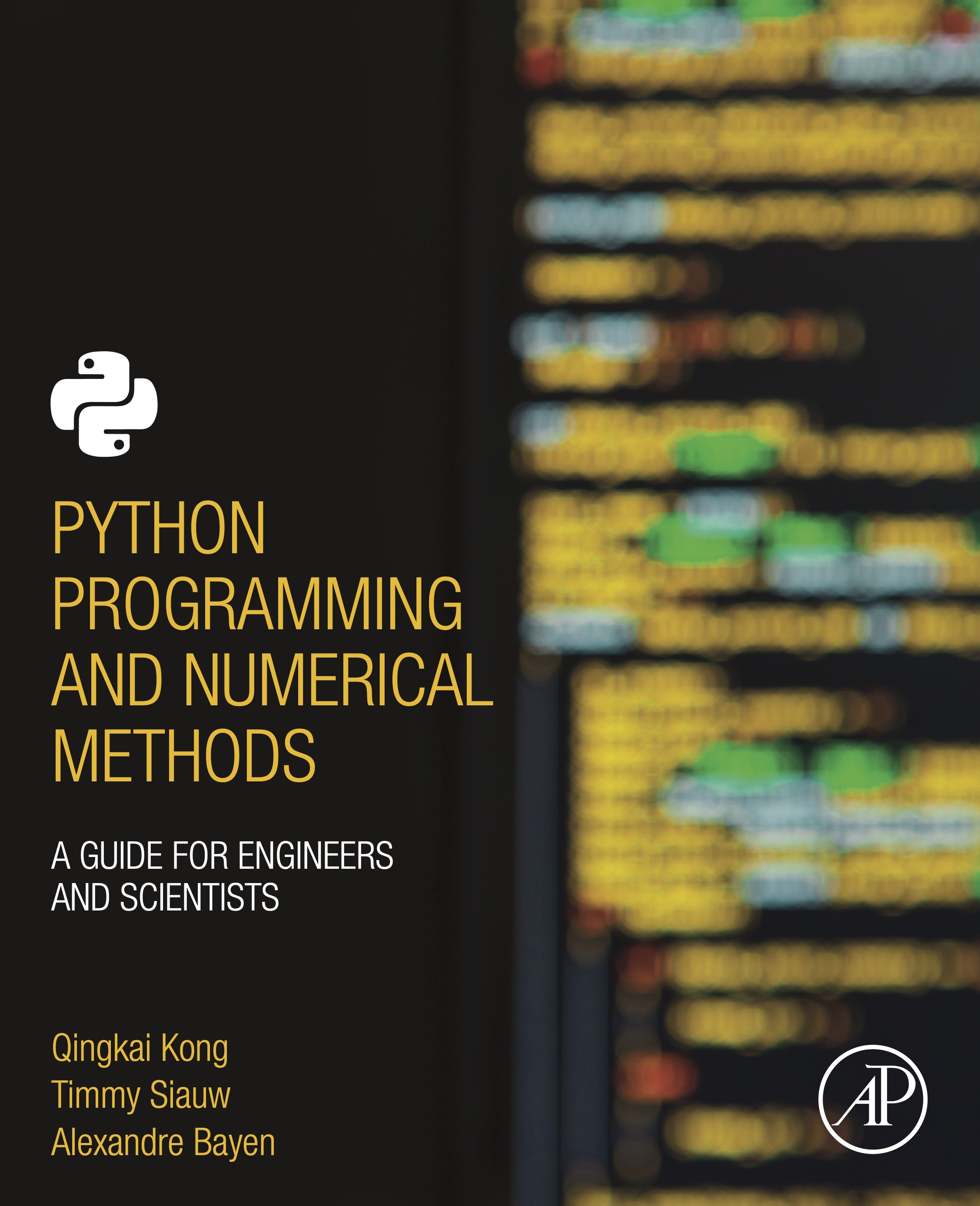
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
CS101: Introduction to Computer Science I
Variables and Assignment Statements
Read this chapter, which covers variables and arithmetic operations and order precedence in Java.
9. Assignment Statements
No. The incorrect splittings are highlighted in red:
Assignment Statement
So far, the example programs have been using the value initially put into a variable. Programs can change the value in a variable. An assignment statement changes the value that is held in a variable. The program uses an assignment statement.
The assignment statement puts the value 123 into the variable. In other words, while the program is executing there will be a 64 bit section of memory that holds the value 123.
Remember that the word "execute" is often used to mean "run". You speak of "executing a program" or "executing" a line of the program.
Question 10:
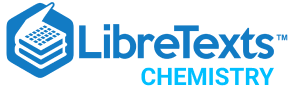
- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
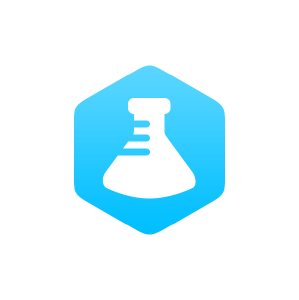
2.3: Arithmetic Operations and Assignment Statements
- Last updated
- Save as PDF
- Page ID 206261
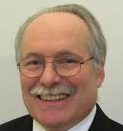
- Robert Belford
- University of Arkansas at Little Rock
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
hypothes.is tag: s20iostpy03ualr Download Assignment: S2020py03
Learning Objectives
Students will be able to:
- Explain each Python arithmetic operator
- Explain the meaning and use of an assignment statement
- Explain the use of "+" and "*" with strings and numbers
- Use the int() and float() functions to convert string input to numbers for computation
- Incorporate numeric formatting into print statements
- Recognize the four main operations of a computer within a simple Python program
- Create input statements in Python
- Create Python code that performs mathematical and string operations
- Create Python code that uses assignment statements
- Create Python code that formats numeric output
Prior Knowledge
- Understanding of Python print and input statements
- Understanding of mathematical operations
- Understanding of flowchart input symbols
Further Reading
- https://en.wikibooks.org/wiki/Non-Programmer%27s_Tutorial_for_Python_3/Hello,_World
- https://en.wikibooks.org/wiki/Non-Programmer%27s_Tutorial_for_Python_3/Who_Goes_There%3F
Model 1: Arithmetic Operators in Python
Python includes several arithmetic operators: addition, subtraction, multiplication, two types of division, exponentiation and mod .
Critical Thinking Questions:
1. Draw a line between each flowchart symbol and its corresponding line of Python code. Make note of any problems.
2. Execute the print statements in the previous Python program
a. Next to each print statement above, write the output. b. What is the value of the following line of code?
c. Predict the values of 17%3 and 18%3 without using your computer.
3. Explain the purpose of each arithmetic operation:
a. + ____________________________
b. - ____________________________
c. * ____________________________
d. ** ____________________________
e. / ____________________________
f. // ____________________________
g. % ____________________________
An assignment statement is a line of code that uses a "=" sign. The statement stores the result of an operation performed on the right-hand side of the sign into the variable memory location on the left-hand side.
4. Enter and execute the following lines of Python code in the editor window of your IDE (e.g. Thonny):
a. What are the variables in the above python program? b. What does the assignment statement : MethaneMolMs = 16 do? c. What happens if you replace the comma (,) in the print statements with a plus sign (+) and execute the code again? Why does this happen?
5. What is stored in memory after each assignment statement is executed?
Note: Concatenating Strings in python
The "+" concatenates the two strings stored in the variables into one string. "+" can only be used when both operators are strings.
6. Run the following program in the editor window of your IDE (e.g. Thonny) to see what happens if you try to use the "+" with strings instead of numbers?
a. The third line of code contains an assignment statement. What is stored in fullName when the line is executed? b. What is the difference between the two output lines? c. How could you alter your assignment statements so that print(fullName) gives the same output as print(firstName,lastName) d. Only one of the following programs will work. Which one will work, and why doesn’t the other work? Try doing this without running the programs!
e. Run the programs above and see if you were correct. f. The program that worked above results in no space between the number and the street name. How can you alter the code so that it prints properly while using a concatenation operator?
7. Before entering the following code into the Python interpreter (Thonny IDE editor window), predict the output of this program.
Now execute it. What is the actual output? Is this what you thought it would do? Explain.
8. Let’s take a look at a python program that prompts the user for two numbers and subtracts them.
Execute the following code by entering it in the editor window of Thonny.
a. What output do you expect? b. What is the actual output c. Revise the program in the following manner:
- Between lines two and three add the following lines of code: num1 = int(firstNumber) num2 = int(secondNumber)
- Next, replace the statement: difference = firstNumber – secondNumber with the statement: difference = num1 – num2
- Execute the program again. What output did you get?
d. Explain the purpose of the function int(). e. Explain how the changes in the program produced the desired output.
Model 3: Formatting Output in Python
There are multiple ways to format output in python. The old way is to use the string modulo %, and the new way is with a format method function.
9. Look closely at the output for python program 7.
a. How do you indicate the number of decimals to display using
the string modulo (%) ______________________________________________________
the format function ________________________________________________________
b. What happens to the number if you tell it to display less decimals than are in the number, regardless of formatting method used?
c. What type of code allows you to right justify your numbers?
10. Execute the following code by entering it in the editor window of Thonny.
a. Does the output look like standard output for something that has dollars and cents associated with it?
b. Replace the last line of code with the following:
print("Total cost of laptops: $%.2f" % price)
print("Total cost of laptops:" ,format(price, '.2f.))
Discuss the change in the output.
c. Replace the last line of code with the following:
print("Total cost of laptops: $", format(price,'.2f') print("Total cost of laptops: $" ,format(price, '.2f.))
Discuss the change in the output.
d. Experiment with the number ".2" in the ‘0.2f’ of the print above statement by substituting the following numbers and explain the results.
.4 ___________________________________________________
.0 ___________________________________________________
.1 ___________________________________________________
.8 ___________________________________________________
e. Now try the following numbers in the same print statement. These numbers contain a whole number and a decimal. Explain the output for each number.
02.5 ___________________________________________________
08.2 ___________________________________________________
03.1 ___________________________________________________
f. Explain what each part of the format function: format(variable, "%n.nf") does in a print statement where n.n represents a number.
variable ____________________________ First n _________________________
Second n_______________________ f _________________________
g. Revise the print statement by changing the "f" to "d" and laptopCost = 600 . Execute the statements and explain the output format.
print("Total cost of laptops: %2d" % price) print("Total cost of laptops: %10d" % price)
h. Explain how the function format(var,'10d') formats numeric data. var represents a whole number.
11. Use the following program and output to answer the questions below.
a. From the code and comments in the previous program, explain how the four main operations are implemented in this program. b. There is one new function in this sample program. What is it? From the corresponding output, determine what it does.
Application Questions: Use the Python Interpreter to check your work
- 8 to the 4 th power
- The sum of 5 and 6 multiplied by the quotient of 34 and 7 using floating point arithmetic
- Write an assignment statement that stores the remainder obtained from dividing 87 and 8 in the variable leftover
- Assume:
courseLabel = "CHEM" courseNumber = "3350"
Write a line of Python code that concatenates the label with the number and stores the result in the variable courseName . Be sure that there is a space between the course label and the course number when they are concatenated.
- Write one line of Python code that will print the word "Happy!" one hundred times.
- Write one line of code that calculates the cost of 15 items and stores the result in the variable totalCost
- Write one line of code that prints the total cost with a label, a dollar sign, and exactly two decimal places. Sample output: Total cost: $22.5
- Assume:
height1 = 67850 height2 = 456
Use Python formatting to write two print statements that will produce the following output exactly at it appears below:

Homework Assignment: s2020py03
Download the assignment from the website, fill out the word document, and upload to your Google Drive folder the completed assignment along with the two python files.
1. (5 pts) Write a Python program that prompts the user for two numbers, and then gives the sum and product of those two numbers. Your sample output should look like this:
Enter your first number:10 Enter your second number:2 The sum of these numbers is: 12 The product of these two numbers is: 20
- Your program must contain documentation lines that include your name, the date, a line that states "Py03 Homework question 1" and a description line that indicates what the program is supposed to do.
- Paste the code this word document and upload to your Google drive when the assignment is completed, with file name [your last name]_py03_HWQ1
- Save the program as a python file (ends with .py), with file name [your last name]_py03Q1_program and upload that to the Google Drive.
2. (10 pts) Write a program that calculates the molarity of a solution. Molarity is defined as numbers of moles per liter solvent. Your program will calculate molarity and must ask for the substance name, its molecular weight, how many grams of substance you are putting in solution, and the total volume of the solution. Report your calculated value of molarity to 3 decimal places. Your output should also be separated from the input with a line containing 80 asterixis.
Assuming you are using sodium chloride, your input and output should look like:
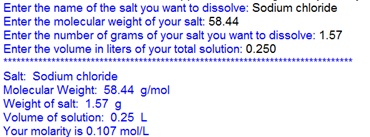
- Your program must contain documentation lines that include your name, the date, a line that states "Py03 Homework question 2" and a description line that indicates what the program is supposed to do.
- Paste the code to question two below
- Save the program as a python file (ends with .py), with file name [your last name]_py03Q2_program and upload that to the Google Drive.
3. (4 pts) Make two hypothes.is annotations dealing with external open access resources on formatting with the format function method of formatting. These need the tag of s20iostpy03ualr .
Copyright Statement

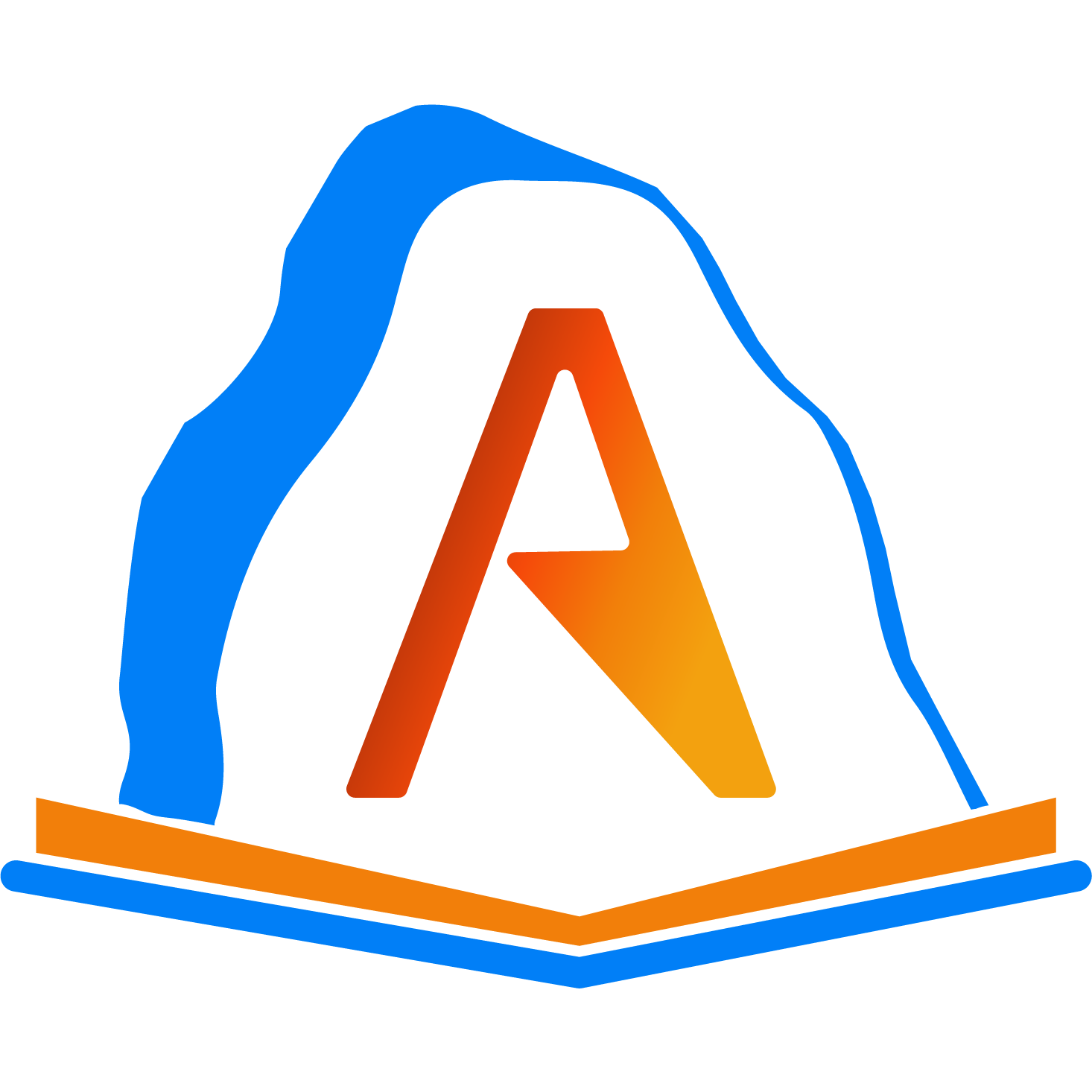
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Preface
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Variables
- 1.7 Java Development Environments (optional)
- 1.8 Unit 1 Summary
- 1.9 Unit 1 Mixed Up Code Practice
- 1.10 Unit 1 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.12 Lesson Workspace
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
Before you keep reading...
Runestone Academy can only continue if we get support from individuals like you. As a student you are well aware of the high cost of textbooks. Our mission is to provide great books to you for free, but we ask that you consider a $10 donation, more if you can or less if $10 is a burden.
Making great stuff takes time and $$. If you appreciate the book you are reading now and want to keep quality materials free for other students please consider a donation to Runestone Academy. We ask that you consider a $10 donation, but if you can give more thats great, if $10 is too much for your budget we would be happy with whatever you can afford as a show of support.
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side of the = sign. The value of the expression on the right hand side of the = sign (which can contain math operators and other variables) is copied into the memory location of the variable on the left hand side.
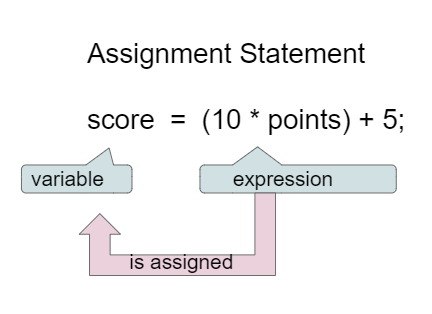
Figure 1: Assignment Statement (variable = expression) ¶
Instead of saying equals for the = operator in an assignment statement, say “gets” or “is assigned” to remember that the variable on the left hand side gets or is assigned the value on the right. In the figure above, score is assigned the value of 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable to a copy of the value of another variable like y = x;. This won’t change the value of the variable that you are copying from.

Click on the Show CodeLens button to step through the code and see how the values of the variables change.
The program is supposed to figure out the total money value given the number of dimes, quarters and nickels. There is an error in the calculation of the total. Fix the error to compute the correct amount.
Calculate and print the total pay given the weekly salary and the number of weeks worked. Use string concatenation with the totalPay variable to produce the output Total Pay = $3000 . Don’t hardcode the number 3000 in your print statement.

Assume you have a package with a given height 3 inches and width 5 inches. If the package is rotated 90 degrees, you should swap the values for the height and width. The code below makes an attempt to swap the values stored in two variables h and w, which represent height and width. Variable h should end up with w’s initial value of 5 and w should get h’s initial value of 3. Unfortunately this code has an error and does not work. Use the CodeLens to step through the code to understand why it fails to swap the values in h and w.
1-4-7: Explain in your own words why the ErrorSwap program code does not swap the values stored in h and w.
Swapping two variables requires a third variable. Before assigning h = w , you need to store the original value of h in the temporary variable. In the mixed up programs below, drag the blocks to the right to put them in the right order.
The following has the correct code that uses a third variable named “temp” to swap the values in h and w.
The code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right, then check your solution. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks.
After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
Fix the code below to perform a correct swap of h and w. You need to add a new variable named temp to use for the swap.
1.4.2. Incrementing the value of a variable ¶
If you use a variable to keep score you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one (score = score + 1) as shown below. The formula looks a little crazy in math class, but it makes sense in coding because the variable on the left is set to the value of the arithmetic expression on the right. So, the score variable is set to the previous value of score + 1.
Click on the Show CodeLens button to step through the code and see how the score value changes.
1-4-11: What is the value of b after the following code executes?
- It sets the value for the variable on the left to the value from evaluating the right side. What is 5 * 2?
- Correct. 5 * 2 is 10.
1-4-12: What are the values of x, y, and z after the following code executes?
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 1, y = 0, z = 3
1.4.3. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Arithmetic expressions can be of type int or double. An arithmetic operation that uses two int values will evaluate to an int value. An arithmetic operation that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to put text together in the input program above – more on this when we talk about strings.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == ! They mean different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Use == and != only with int values and not doubles because double values are an approximation and 3.3333 will not equal 3.3334 even though they are very close.
Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3 ? Isn’t surprising that it prints 0 ? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer, essentially rounding down the answer to a whole number. If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, so that *, /, and % are done before + and -. However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence .
1-4-15: Consider the following code segment. Be careful about integer division.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int result where it is rounded down to the nearest int.
1-4-16: Consider the following code segment.
What is the value of the expression?
- Dividing an integer by an integer results in an integer
- Correct. Dividing an integer by an integer results in an integer
- The value 5.5 will be rounded down to 5
1-4-17: Consider the following code segment.
- Correct. Dividing a double by an integer results in a double
- Dividing a double by an integer results in a double
1-4-18: Consider the following code segment.
- Correct. Dividing an integer by an double results in a double
- Dividing an integer by an double results in a double
1.4.4. The Modulo Operator ¶
The percent sign operator ( % ) is the mod (modulo) or remainder operator. The mod operator ( x % y ) returns the remainder after you divide x (first number) by y (second number) so 5 % 2 will return 1 since 2 goes into 5 two times with a remainder of 1. Remember long division when you had to specify how many times one number went into another evenly and the remainder? That remainder is what is returned by the modulo operator.
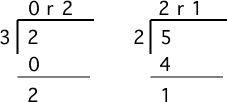
Figure 2: Long division showing the whole number result and the remainder ¶
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x . The value y can’t go into x at all (goes in 0 times), since x is smaller than y , so the result is just x . So if you see 2 % 3 the result is 2 .
1-4-21: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. modulo gives you the remainder.
- modulo gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-22: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.5. FlowCharting ¶
Assume you have 16 pieces of pizza and 5 people. If everyone gets the same number of slices, how many slices does each person get? Are there any leftover pieces?
In industry, a flowchart is used to describe a process through symbols and text. A flowchart usually does not show variable declarations, but it can show assignment statements (drawn as rectangle) and output statements (drawn as rhomboid).
The flowchart in figure 3 shows a process to compute the fair distribution of pizza slices among a number of people. The process relies on integer division to determine slices per person, and the mod operator to determine remaining slices.
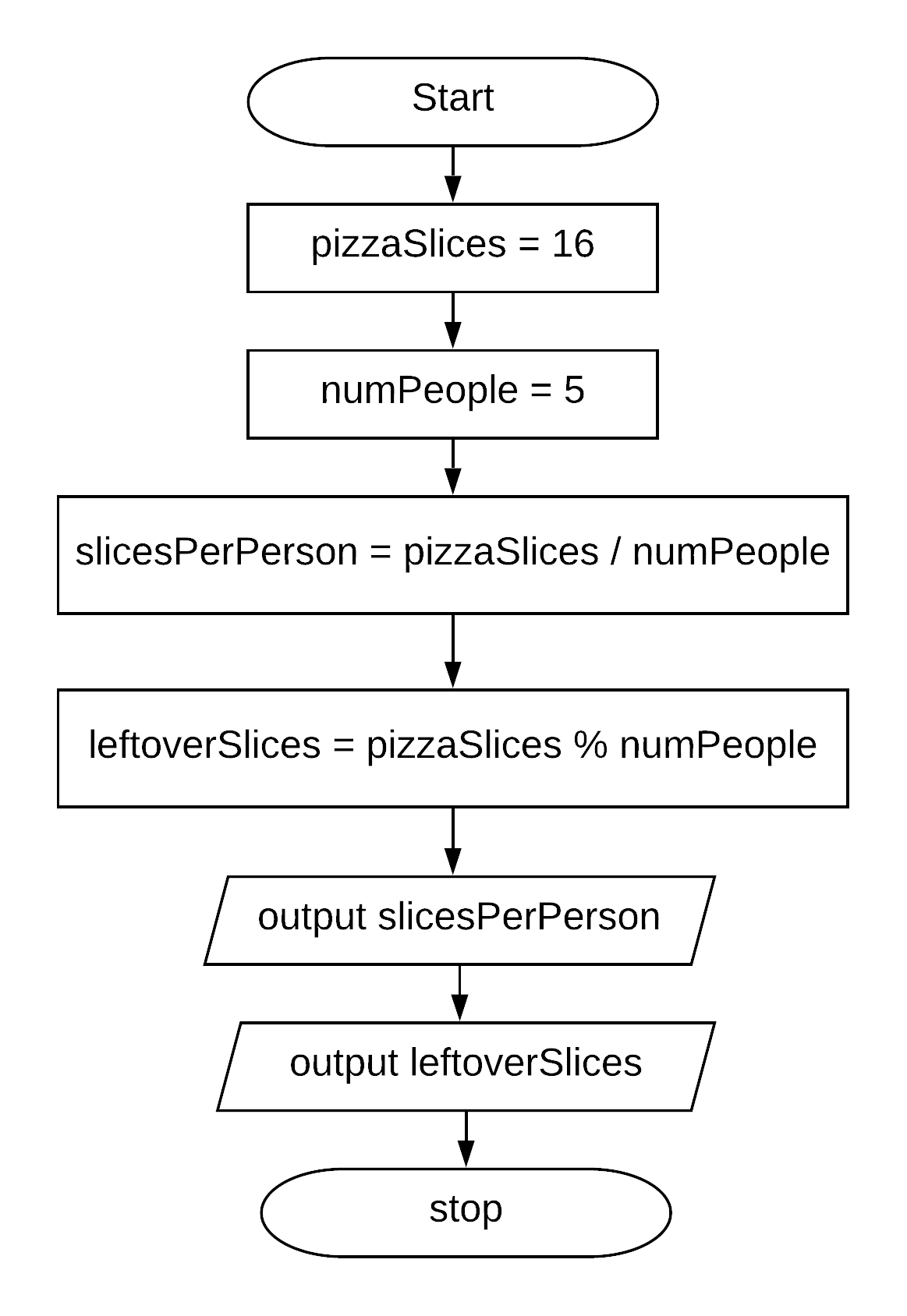
Figure 3: Example Flow Chart ¶
A flowchart shows pseudo-code, which is like Java but not exactly the same. Syntactic details like semi-colons are omitted, and input and output is described in abstract terms.
Complete the program based on the process shown in the Figure 3 flowchart. Note the first line of code declares all 4 variables as type int. Add assignment statements and print statements to compute and print the slices per person and leftover slices. Use System.out.println for output.

1.4.6. Storing User Input in Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables.

Figure 4: Program input and output ¶
A Java program can ask the user to type in one or more values. The Java class Scanner is used to read from the keyboard input stream, which is referenced by System.in . Normally the keyboard input is typed into a console window, but since this is running in a browser you will type in a small textbox window displayed below the code. The code below shows an example of prompting the user to enter a name and then printing a greeting. The code String name = scan.nextLine() gets the string value you enter as program input and then stores the value in a variable.
Run the program a few times, typing in a different name. The code works for any name: behold, the power of variables!
Run this program to read in a name from the input stream. You can type a different name in the input window shown below the code.
Try stepping through the code with the CodeLens tool to see how the name variable is assigned to the value read by the scanner. You will have to click “Hide CodeLens” and then “Show in CodeLens” to enter a different name for input.
The Scanner class has several useful methods for reading user input. A token is a sequence of characters separated by white space.
Run this program to read in an integer from the input stream. You can type a different integer value in the input window shown below the code.
A rhomboid (slanted rectangle) is used in a flowchart to depict data flowing into and out of a program. The previous flowchart in Figure 3 used a rhomboid to indicate program output. A rhomboid is also used to denote reading a value from the input stream.
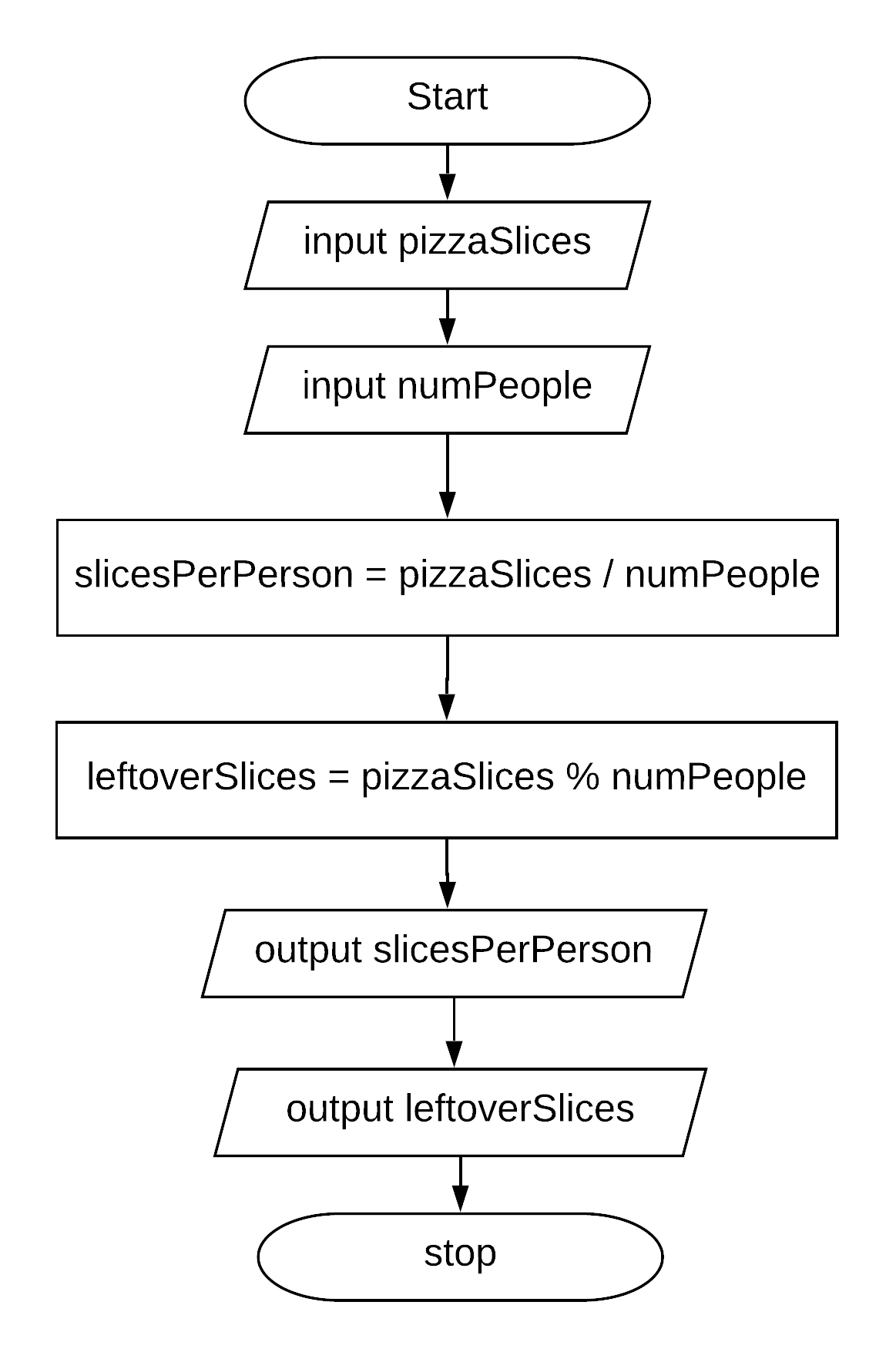
Figure 5: Flow Chart Reading User Input ¶
Figure 5 contains an updated version of the pizza calculator process. The first two steps have been altered to initialize the pizzaSlices and numPeople variables by reading two values from the input stream. In Java this will be done using a Scanner object and reading from System.in.
Complete the program based on the process shown in the Figure 5 flowchart. The program should scan two integer values to initialize pizzaSlices and numPeople. Run the program a few times to experiment with different values for input. What happens if you enter 0 for the number of people? The program will bomb due to division by zero! We will see how to prevent this in a later lesson.
The program below reads two integer values from the input stream and attempts to print the sum. Unfortunately there is a problem with the last line of code that prints the sum.
Run the program and look at the result. When the input is 5 and 7 , the output is Sum is 57 . Both of the + operators in the print statement are performing string concatenation. While the first + operator should perform string concatenation, the second + operator should perform addition. You can force the second + operator to perform addition by putting the arithmetic expression in parentheses ( num1 + num2 ) .
More information on using the Scanner class can be found here https://www.w3schools.com/java/java_user_input.asp
1.4.7. Programming Challenge : Dog Years ¶
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years. If you want an extra challenge, try reading the values using a Scanner.
1.4.8. Summary ¶
Arithmetic expressions include expressions of type int and double.
The arithmetic operators consist of +, -, * , /, and % (modulo for the remainder in division).
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away, essentially rounding down the answer to a whole number.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. (*, /, % have precedence over + and -, unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException to occur.
The assignment operator (=) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the evaluation of the expression.

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = operator. For example:
In the above assignment statement, the result of x + 1 is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1 ≠ the Java expression x = 2 * x + 1
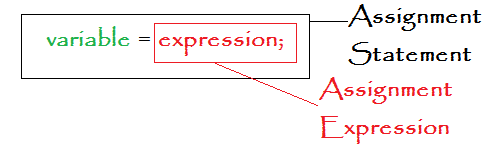
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | ◘
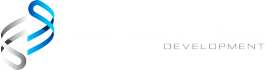
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages

Variable Assignment
To "assign" a variable means to symbolically associate a specific piece of information with a name. Any operations that are applied to this "name" (or variable) must hold true for any possible values. The assignment operator is the equals sign which SHOULD NEVER be used for equality, which is the double equals sign.
The '=' symbol is the assignment operator. Warning, while the assignment operator looks like the traditional mathematical equals sign, this is NOT the case. The equals operator is '=='
Design Pattern
To evaluate an assignment statement:
- Evaluate the "right side" of the expression (to the right of the equal sign).
- Once everything is figured out, place the computed value into the variables bucket.
We've already seen many examples of assignment. Assignment means: "storing a value (of a particular type) under a variable name" . Think of each assignment as copying the value of the righthand side of the expression into a "bucket" associated with the left hand side name!
Read this as, the variable called "name" is "assigned" the value computed by the expression to the right of the assignment operator ('=');
Now that you have seen some variables being assigned, tell me what the following code means?
The answer to above questions: the assignment means that lkjasdlfjlskdfjlksjdflkj is a variable (a really badly named one), but a variable none-the-less. jlkajdsf and lkjsdflkjsdf must also be variables. The sum of the two numbers held in jlkajdsf and lkjsdflkjsdf is stored in the variable lkjasdlfjlskdfjlksjdflkj.
Examples of builtin Data and Variables (and Constants)
For more info, use the "help" command: (e.g., help realmin);
Examples of using Data and Variable
Pattern to memorize, assignment pattern.
The assignment pattern creates a new variable, if this is the first time we have seen the "name", or, updates the variable to a new value!
Read the following code in English as: First, compute the value of the thing to the right of the assignment operator (the =). then store the computed value under the given name, destroying anything that was there before.
Or more concisely: assign the variable "name" the value computed by "right_hand_expression"

1.4 — Variable assignment and initialization
In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we’ll explore how to actually put values into variables and use those values.
As a reminder, here’s a short snippet that first allocates a single integer variable named x , then allocates two more integer variables named y and z :
Variable assignment
After a variable has been defined, you can give it a value (in a separate statement) using the = operator . This process is called assignment , and the = operator is called the assignment operator .
By default, assignment copies the value on the right-hand side of the = operator to the variable on the left-hand side of the operator. This is called copy assignment .
Here’s an example where we use assignment twice:
This prints:
When we assign value 7 to variable width , the value 5 that was there previously is overwritten. Normal variables can only hold one value at a time.
One of the most common mistakes that new programmers make is to confuse the assignment operator ( = ) with the equality operator ( == ). Assignment ( = ) is used to assign a value to a variable. Equality ( == ) is used to test whether two operands are equal in value.
Initialization
One downside of assignment is that it requires at least two statements: one to define the variable, and another to assign the value.
These two steps can be combined. When an object is defined, you can optionally give it an initial value. The process of specifying an initial value for an object is called initialization , and the syntax used to initialize an object is called an initializer .
In the above initialization of variable width , { 5 } is the initializer, and 5 is the initial value.
Different forms of initialization
Initialization in C++ is surprisingly complex, so we’ll present a simplified view here.
There are 6 basic ways to initialize variables in C++:
You may see the above forms written with different spacing (e.g. int d{7}; ). Whether you use extra spaces for readability or not is a matter of personal preference.
Default initialization
When no initializer is provided (such as for variable a above), this is called default initialization . In most cases, default initialization performs no initialization, and leaves a variable with an indeterminate value.
We’ll discuss this case further in lesson ( 1.6 -- Uninitialized variables and undefined behavior ).
Copy initialization
When an initial value is provided after an equals sign, this is called copy initialization . This form of initialization was inherited from C.
Much like copy assignment, this copies the value on the right-hand side of the equals into the variable being created on the left-hand side. In the above snippet, variable width will be initialized with value 5 .
Copy initialization had fallen out of favor in modern C++ due to being less efficient than other forms of initialization for some complex types. However, C++17 remedied the bulk of these issues, and copy initialization is now finding new advocates. You will also find it used in older code (especially code ported from C), or by developers who simply think it looks more natural and is easier to read.
For advanced readers
Copy initialization is also used whenever values are implicitly copied or converted, such as when passing arguments to a function by value, returning from a function by value, or catching exceptions by value.
Direct initialization
When an initial value is provided inside parenthesis, this is called direct initialization .
Direct initialization was initially introduced to allow for more efficient initialization of complex objects (those with class types, which we’ll cover in a future chapter). Just like copy initialization, direct initialization had fallen out of favor in modern C++, largely due to being superseded by list initialization. However, we now know that list initialization has a few quirks of its own, and so direct initialization is once again finding use in certain cases.
Direct initialization is also used when values are explicitly cast to another type.
One of the reasons direct initialization had fallen out of favor is because it makes it hard to differentiate variables from functions. For example:
List initialization
The modern way to initialize objects in C++ is to use a form of initialization that makes use of curly braces. This is called list initialization (or uniform initialization or brace initialization ).
List initialization comes in three forms:
As an aside…
Prior to the introduction of list initialization, some types of initialization required using copy initialization, and other types of initialization required using direct initialization. List initialization was introduced to provide a more consistent initialization syntax (which is why it is sometimes called “uniform initialization”) that works in most cases.
Additionally, list initialization provides a way to initialize objects with a list of values (which is why it is called “list initialization”). We show an example of this in lesson 16.2 -- Introduction to std::vector and list constructors .
List initialization has an added benefit: “narrowing conversions” in list initialization are ill-formed. This means that if you try to brace initialize a variable using a value that the variable can not safely hold, the compiler is required to produce a diagnostic (usually an error). For example:
In the above snippet, we’re trying to assign a number (4.5) that has a fractional part (the .5 part) to an integer variable (which can only hold numbers without fractional parts).
Copy and direct initialization would simply drop the fractional part, resulting in the initialization of value 4 into variable width . Your compiler may optionally warn you about this, since losing data is rarely desired. However, with list initialization, your compiler is required to generate a diagnostic in such cases.
Conversions that can be done without potential data loss are allowed.
To summarize, list initialization is generally preferred over the other initialization forms because it works in most cases (and is therefore most consistent), it disallows narrowing conversions, and it supports initialization with lists of values (something we’ll cover in a future lesson). While you are learning, we recommend sticking with list initialization (or value initialization).
Best practice
Prefer direct list initialization (or value initialization) for initializing your variables.
Author’s note
Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) also recommend using list initialization to initialize your variables.
In modern C++, there are some cases where list initialization does not work as expected. We cover one such case in lesson 16.2 -- Introduction to std::vector and list constructors .
Because of such quirks, some experienced developers now advocate for using a mix of copy, direct, and list initialization, depending on the circumstance. Once you are familiar enough with the language to understand the nuances of each initialization type and the reasoning behind such recommendations, you can evaluate on your own whether you find these arguments persuasive.
Value initialization and zero initialization
When a variable is initialized using empty braces, value initialization takes place. In most cases, value initialization will initialize the variable to zero (or empty, if that’s more appropriate for a given type). In such cases where zeroing occurs, this is called zero initialization .
Q: When should I initialize with { 0 } vs {}?
Use an explicit initialization value if you’re actually using that value.
Use value initialization if the value is temporary and will be replaced.
Initialize your variables
Initialize your variables upon creation. You may eventually find cases where you want to ignore this advice for a specific reason (e.g. a performance critical section of code that uses a lot of variables), and that’s okay, as long as the choice is made deliberately.
Related content
For more discussion on this topic, Bjarne Stroustrup (creator of C++) and Herb Sutter (C++ expert) make this recommendation themselves here .
We explore what happens if you try to use a variable that doesn’t have a well-defined value in lesson 1.6 -- Uninitialized variables and undefined behavior .
Initialize your variables upon creation.
Initializing multiple variables
In the last section, we noted that it is possible to define multiple variables of the same type in a single statement by separating the names with a comma:
We also noted that best practice is to avoid this syntax altogether. However, since you may encounter other code that uses this style, it’s still useful to talk a little bit more about it, if for no other reason than to reinforce some of the reasons you should be avoiding it.
You can initialize multiple variables defined on the same line:
Unfortunately, there’s a common pitfall here that can occur when the programmer mistakenly tries to initialize both variables by using one initialization statement:
In the top statement, variable “a” will be left uninitialized, and the compiler may or may not complain. If it doesn’t, this is a great way to have your program intermittently crash or produce sporadic results. We’ll talk more about what happens if you use uninitialized variables shortly.
The best way to remember that this is wrong is to consider the case of direct initialization or brace initialization:
Because the parenthesis or braces are typically placed right next to the variable name, this makes it seem a little more clear that the value 5 is only being used to initialize variable b and d , not a or c .
Unused initialized variables warnings
Modern compilers will typically generate warnings if a variable is initialized but not used (since this is rarely desirable). And if “treat warnings as errors” is enabled, these warnings will be promoted to errors and cause the compilation to fail.
Consider the following innocent looking program:
When compiling this with the g++ compiler, the following error is generated:
and the program fails to compile.
There are a few easy ways to fix this.
- If the variable really is unused, then the easiest option is to remove the defintion of x (or comment it out). After all, if it’s not used, then removing it won’t affect anything.
- Another option is to simply use the variable somewhere:
But this requires some effort to write code that uses it, and has the downside of potentially changing your program’s behavior.
The [[maybe_unused]] attribute C++17
In some cases, neither of the above options are desirable. Consider the case where we have a bunch of math/physics values that we use in many different programs:
If we use these a lot, we probably have these saved somewhere and copy/paste/import them all together.
However, in any program where we don’t use all of these values, the compiler will complain about each variable that isn’t actually used. While we could go through and remove/comment out the unused ones for each program, this takes time and energy. And later if we need one that we’ve previously removed, we’ll have to go back and re-add it.
To address such cases, C++17 introduced the [[maybe_unused]] attribute, which allows us to tell the compiler that we’re okay with a variable being unused. The compiler will not generate unused variable warnings for such variables.
The following program should generate no warnings/errors:
Additionally, the compiler will likely optimize these variables out of the program, so they have no performance impact.
In future lessons, we’ll often define variables we don’t use again, in order to demonstrate certain concepts. Making use of [[maybe_unused]] allows us to do so without compilation warnings/errors.
Question #1
What is the difference between initialization and assignment?
Show Solution
Initialization gives a variable an initial value at the point when it is created. Assignment gives a variable a value at some point after the variable is created.
Question #2
What form of initialization should you prefer when you want to initialize a variable with a specific value?
Direct list initialization (aka. direct brace initialization).
Question #3
What are default initialization and value initialization? What is the behavior of each? Which should you prefer?
Default initialization is when a variable initialization has no initializer (e.g. int x; ). In most cases, the variable is left with an indeterminate value.
Value initialization is when a variable initialization has an empty brace (e.g. int x{}; ). In most cases this will perform zero-initialization.
You should prefer value initialization to default initialization.
Now that we’ve learned how to use the print(expression) statement, let’s focus on the next major concept in Python, as well as any other programming language: variables .
The word variable is traditionally defined as a value that can change. We’ve seen variables like $ x $ used in Algebraic equations like $ x + 4 = 7 $ to represent unknown values that we can try to work out. In programming a variable is defined as a way to store a value in a computer’s memory so we can retrieve it later. One common way to think of variables is like a box in the real world. We can put something in the box, representing our value. Likewise, we can write a name on the side of the box, corresponding to our variable’s name. When we want to use the variable, we can get the value that it currently stores, and even change it to a different value. It’s a pretty handy mental metaphor to keep in mind!
In a later lab, we’ll learn how to use operators to manipulate the values stored in variables, but for right now we’re just going to focus on storing and retrieving data using variables.
Creating Variables
To use a variable, we must first create one. In Python, we create a variable in a special type of statement called an assignment statement . The basic structure for an assignment statement is a = expression . When our Python interpreter runs this statement, it will first evaluate expression into a single value. Then, it will store that value in the variable named a .
For example, let’s consider the Python statement:
In that statement, we are storing the string value "Hello World" in the variable named x . Pretty handy!
Now, let’s cover some important rules related to assignment statements:
- Assignment statements are always written with the variable on the left, and an expression on the right. We cannot reverse the statement and say expression = x in programming like we can in math. In mathematical terms, this means an assignment statement is not commutative.
- The left side of an assignment statement must be a location where a value can be stored. For now, we’re just going to work with single variables, so we don’t have to worry about this yet. In a later lab, we’ll introduce lists as another way to store data, and we’ll revisit this rule.
- The right side of an assignment statement must be an expression that evaluates to a value that can be stored in the location given on the left side. Right now we’re only working with string values, so we don’t have to worry about this rule yet. We’ll come back to it in a future lab.
Using Variables
Once we’ve created a variable, we can use it in any expression to recall the current value stored in the variable. So, we can extend our previous example to store a value in a variable, and then use the print(expression) statement to display it’s value. Here’s what that would look like in Python:
Notice that we don’t put quotation marks " around the variable x in the print(expression) statement. This is because we want to display the value stored in the variable x , not the string value "x" . So, when we run this code, we should get this output:
To confirm, feel free to try it yourself! Copy the code above into a Python file, then use the python3 command in the terminal to run the file and see what it does. Running these examples is a great way to learn how a computer actually executes code, and it helps to confirm that your “mental model” of a computer matches how a real computer operates.
Updating Variable Values
Python also allows us to change the value stored in a variable using another assignment statement . For example, we can write some Python code that uses the same variable to print multiple outputs:
When we run this code, we’ll see the following output:
So, just like we learned above, when we evaluate a variable in code, it will result in the value currently stored in that variable at the time it is evaluated. So, even though we are printing the same variable twice, each time it is storing a different value. Recall that this is why we call items like a a variable - their value can change!
Variable Names
Finally, Python has a few simple rules that determine what names can be used for variables in our code. Let’s quickly review those rules, as well as some conventions that most Python developers follow when naming variables.
First, the rules that must be followed:
- Variable names must begin with either a letter or an underscore _ .
- Variable names may only contain letters, numbers, and underscores _ .
- Variable names are case sensitive.
Next, here are the conventions that most Python developers follow for variable names, which we will also follow in this course:
- Variable names beginning with an underscore _ have a special meaning. So, we won’t create any variables beginning with an underscore right now, but later we’ll learn about what they mean and start using them.
- Variables should have a descriptive name, like total or average, that makes it clear what the variable is used for.
- Variables should be named using Snake Case . This means that spaces are represented by underscores _ , as in number_of_inputs
- tmp or temp are temporary variables.
- i , j , and k are iterator variables (we’ll learn about those later).
- x , y , and z are coordinates in a coordinate plane.
- r , g , b , a are colors in an RGB color system.
- For example, Python has a print statement, so we should not name a variable print in our language.
- In general, longer variable names are more useful than short ones, even if they are more difficult to type.
These conventions are not strict requirements enforced by the Python language itself, but they are general rules to help us write code that is meaningful and easy to read.
Finally, don’t forget that some of the code examples in this course will NOT follow these conventions, mainly because long, descriptive variable names might give away the purpose of the code itself. We’ll still follow the rules that are required, but in many cases we’ll use simple variable names so that the focus is learning to read the structure of the code, not inferring what it does based solely on the names of the variables.
Last modified by: Russell Feldhausen May 30, 2023
If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
AP®︎/College Computer Science Principles
Course: ap®︎/college computer science principles > unit 3, storing data in variables, assigning variables, displaying variables, re-assigning variables, pseudocode for variables.
- (Choice A) x ← 200 A x ← 200
- (Choice B) var x = 200 B var x = 200
- (Choice C) x = 200 C x = 200
- (Choice D) var x ← 200 D var x ← 200
Want to join the conversation?
- Upvote Button navigates to signup page
- Downvote Button navigates to signup page
- Flag Button navigates to signup page

- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Different Forms of Assignment Statements in Python
- Statement, Indentation and Comment in Python
- Conditional Statements in Python
- Assignment Operators in Python
- Loops and Control Statements (continue, break and pass) in Python
- Different Ways of Using Inline if in Python
- Difference between "__eq__" VS "is" VS "==" in Python
- Augmented Assignment Operators in Python
- Nested-if statement in Python
- How to write memory efficient classes in Python?
- Difference Between List and Tuple in Python
- A += B Assignment Riddle in Python
- Difference between List VS Set VS Tuple in Python
- Assign Function to a Variable in Python
- Python pass Statement
- Python If Else Statements - Conditional Statements
- Data Classes in Python | Set 5 (post-init)
- Assigning multiple variables in one line in Python
- Assignment Operators in Programming
- What is the difference between = (Assignment) and == (Equal to) operators
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Please Login to comment...
Similar reads.
- python-basics
- Python Programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Lesson 4: Assignment Statements and Numeric Functions
Once you've read your data into a SAS data set, surely you want to do something with it. A common thing to do is to change the original data in some way in an attempt to answer a research question of interest to you. You can change the data in one of two ways:
- You can use a basic assignment statement in which you add some information to all of the observations in the data set. Some assignment statements may take advantage of the numerous SAS functions that are available to make programming certain calculations easier ( e.g. , taking an average).
- Alternatively, you can use an if-then-else statement to add some information to some but not all of the observations. In this lesson, we will learn how to use assignment statements and numeric SAS functions to change your data. In the next lesson, we will learn how to use if-then-else statements to change a subset of your data.
Modifying your data may involve not only changing the values of a particular variable but also the type of the variable. That is, you might need to change a character variable to a numeric variable. For that reason, we'll investigate how to use the INPUT function to convert character data values to numeric values. (We'll learn how to use the PUT function to convert numeric values to character values in Stat 481 when we study character functions in depth.)
Upon completing this lesson, you should be able to do the following:
- write a basic assignment statement involving a numeric variable
- write an assignment statement that involves an arithmetic calculation
- write an assignment statement that utilizes one of the many numeric SAS functions that are available
- describe how SAS handles missing values for various arithmetic calculations and functions
- write an assignment statement involving nested functions
- write a basic assignment statement involving a character variable
- convert character data values to numeric values using the INPUT function
4.1 - Assignment Statement Basics
The fundamental method of modifying the data in a data set is by way of a basic assignment statement. Such a statement always takes the form:
variable = expression;
where the variable is any valid SAS name and the expression is the calculation that is necessary to give the variable its values. The variable must always appear to the left of the equal sign and the expression must always appear to the right of the equal sign. As always, the statement must end with a semicolon (;).
Because assignment statements involve changing the values of variables, in the process of learning about assignment statements we'll get practice with working with both numeric and character variables. We'll also learn how using numeric SAS functions can help to simplify some of our calculations.
Example 4.1
Throughout this lesson, we'll work on modifying various aspects of the temporary data set grades that are created in the following DATA step:
The data set contains student names ( name ), each of their four exam grades ( e1 , e2 , e3 , e4 ), their project grade ( p1 ), and their final exam grade ( f1 ).
A couple of comments. For the sake of the examples that follow, we'll use the DATALINES statement to read in the data. We could have just as easily used the INFILE statement. Additionally, for the sake of ease, we'll create temporary data sets rather than permanent ones. Finally, after each SAS DATA step, we'll use the PRINT procedure to print all or part of the resulting SAS data set for your perusal.
Example 4.2
The following SAS program illustrates a very simple assignment statement in which SAS adds up the four exam scores of each student and stores the result in a new numeric variable called examtotal .
Note that, as previously described, the new variable name examtotal appears to the left of the equal sign, while the expression that adds up the four exam scores ( e1 + e2 + e3 + e4 ) appears to the right of the equal sign.
Launch and run the SAS program. Review the output from the PRINT procedure to convince yourself that the new numeric variable examtotal is indeed the sum of the four exam scores for each student appearing in the data set. Also, note what SAS does when it is asked to calculate something when some of the data are missing. Rather than add up the three exam scores that do exist for John Simon, SAS instead assigns a missing value to his examtotal . If you think about it, that's a good thing! Otherwise, you'd have no way of knowing that his examtotal differed in some fundamental way from that of the other students. The important lesson here is to always be aware of how SAS is going to handle the missing values in your data set when you perform various calculations!
Example 4.3
In the previous example, the assignment statement created a new variable in the data set by simply using a variable name that didn't already exist in the data set. You need not always use a new variable name. Instead, you could modify the values of a variable that already exists. The following SAS program illustrates how the instructor would modify the variable e2 , say for example, if she wanted to modify the grades of the second exam by adding 8 points to each student's grade:
Note again that the name of the variable being modified ( e2 ) appears to the left of the equal sign, while the arithmetic expression that tells SAS to add 8 to the second exam score ( e2 +8) appears to the right of the equal sign. In general, when a variable name appears on both sides of the equal sign, the original value on the right side is used to evaluate the expression. The result of the expression is then assigned to the variable on the left side of the equal sign.
Launch and run the SAS program. Review the output from the print procedure to convince yourself that the values of the numeric variable e2 are indeed eight points higher than the values in the original data set.
4.2 - Arithmetic Calculations Using Arithmetic Operators
All we've done so far is add variables together. Of course, we could also subtract, multiply, divide, or exponentiate variables. We just have to make sure that we use the symbols that SAS recognizes. They are:
As is the case in other programming languages, you can perform more than one operation in an assignment statement. The operations are performed as they are for any mathematical expression, namely:
- exponentiation is performed first, then multiplication and division, and finally addition and subtraction
- if multiple instances of addition, multiple instances of subtraction, or addition and subtraction appear together in the same expression, the operations are performed from left to right
- if multiple instances of multiplication, multiple instances of division, or multiplication and division appear together in the same expression, the operations are performed from left to right
- if multiple instances of exponentiation occur in the same expression, the operations are performed right to left
- operations in parentheses are performed first
It's that last bullet that I think is the most helpful to know. If you use parentheses to specifically tell SAS what you want to be calculated first, then you needn't worry as much about the other rules. Let's take a look at two examples.
Example 4.4
The following example contains a calculation that illustrates the standard order of operations. Suppose a statistics instructor calculates the final grade by weighting the average exam score by 0.6, the project score by 0.2, and the final exam by 0.2. The following SAS program illustrates how the instructor (incorrectly) calculates the students' final grades:
Well, okay, so the instructor should stick to statistics and not mathematics. As you can see in the assignment statement, the instructor is attempting to tell SAS to average the four exam scores by adding them up and dividing by 4, and then multiplying the result by 0.6. Let's see what SAS does instead. Launch and run the SAS program, and review the output to see if you can figure out what SAS did, say, for the first student Alexander Smith. If you're still not sure, review the rules for the order of the operations again. The rules tell us that SAS first:
- takes Alexander's first exam score of 78 and multiples it by 0.6 to get 46.8
- takes Alexander's fourth exam score of 69 and divides it by 4 to get 17.25
- takes Alexander's project score of 97 and multiplies it by 0.2 to get 19.4
- takes Alexander's final exam score of 80 and multiplies it by 0.2 to get 16.0
Then, SAS performs all of the addition:
to get his final score of 267.45. Now, maybe that's the final score that Alexander wants, but it is still fundamentally wrong. Let's see if we can help set the statistics instructor straight by taking advantage of that last rule that says operations in parentheses are performed first.
Example 4.5
The following example contains a calculation that illustrates the standard order of operations. Suppose a statistics instructor calculates the final grade by weighting the average exam score by 0.6, the project score by 0.2, and the final exam by 0.2. The following SAS program illustrates how the instructor (correctly) calculates the students' final grades:
Let's dissect the calculation of Alexander's final score again. The assignment statement for final tells SAS:
- to first add Alexander's four exam scores (78, 82, 86, 69) to get 315
- and then divide that total 315 by 4 to get an average exam score of 78.75
- and then multiply the average exam score of 78.75 by 0.6 to get 47.25
- and then take Alexander's project score of 97 and multiply it by 0.2 to get 19.4
- and then take Alexander's final exam score of 80 and multiply it by 0.2 to get 16.0
Then, SAS performs the addition of the last three items:
to get his final score of 82.65. There, that sounds much better. Sorry, Alexander.
Launch and run the SAS program to see how we did. Review the output from the print procedure to convince yourself that the final grades have been calculated as the instructor wishes. By the way, note again that SAS assigns a missing value to the final grade for John Simon.
In this last example, we calculated the students' average exam scores by adding up their four exam grades and dividing them by 4. We could have instead taken advantage of one of the many numeric functions that are available in SAS, namely that of the MEAN function.
4.3 - Numeric Functions
Just as is the case for other programming languages, such as C++ or S-Plus, a SAS function is a pre-programmed routine that returns a value computed from one or more arguments. The standard form of any SAS function is:
For example, if we want to add three variables, a , b, and c , using the SAS function SUM and assign the resulting value to a variable named d , the correct form of our assignment statement is:
In this case, sum is the name of the function, d is the target variable to which the result of the SUM function is assigned, and a , b , and c are the function's arguments. Some functions require a specific number of arguments, whereas other functions, such as SUM, can contain any number of arguments. Some functions require no arguments. As you'll see in the examples that follow, the arguments can be variable names, constants, or even expressions.
SAS offers arithmetic, financial, statistical, and probability functions. There are far too many of these functions to explore them all in detail, but let's take a look at some examples.
Example 4.6
In the previous example, we calculated students' average exam scores by adding up their four exam grades and dividing by 4. Alternatively, we could use the MEAN function. The following SAS program illustrates the calculation of the average exam scores in two ways — by definition and by using the MEAN function:
Launch and run the SAS program. Review the output from the PRINT procedure to convince yourself that the two methods of calculating the average exam scores do indeed yield the same results:
Oooops! What happened? SAS reports that the average exam score for John Simon is 82 when the average is calculated using the MEAN function, but reports a missing value when the average is calculated using the definition. If you study the results, you'll soon figure out that when calculating an average using the MEAN function, SAS ignores the missing values and goes ahead and calculates the average based on the available values.
We can't really make some all-conclusive statement about which method is more appropriate, as it really depends on the situation and the intent of the programmer. Instead, the (very) important lesson here is to know how missing values are handled for the various methods that are available in SAS! We can't possibly address all of the possible calculations and functions in this course. So ... you would be wise to always check your calculations out on a few representative observations to make sure that your SAS programming is doing exactly as you intended. This is another one of those good programming practices to jot down.
Although you can refer to SAS Help and Documentation (under " functions , by category ") for a full accounting of the built-in numeric functions that are available in SAS, here is a list of just some of the numeric functions that can be helpful when performing statistical analyses:
I have used the INT function a number of times when dealing with numbers whose first few digits contain some additional information that I need. For example, the area code in this part of Pennsylvania is 814. If I have phone numbers that are stored as numbers, say, as 8142341230, then I can use the INT function to extract the area code from the number. Let's take a look at an example of this use of the INT function.
Example 4.7
The following SAS program uses the INT function to extract the area codes from a set of ten-digit telephone numbers:
In short, the INT function returns the integer part of the expression contained within parentheses. So, if the phone number is 8145562314, then int ( phone /10000000) becomes int (814.5562314) which becomes, as claimed, the area code 814. Now, launch and run the SAS program, and review the output from the PRINT procedure to convince yourself that the area codes are calculated as claimed.
Example 4.8
One really cool thing is that you can nest functions in SAS (as you can in most programming languages). That is, you can compute a function within another function. When you nest functions, SAS works from the inside out. That is, SAS performs the action in the innermost function first. It uses the result of that function as the argument of the next function, and so on. You can nest any function as long as the function that is used as the argument meets the requirements for the argument.The following SAS program illustrates nested functions when it rounds the students' exam average to the nearest unit:
For example, the average of Alexander's four exams is 78.75 (the sum of 78, 82, 86, and 69 all divided by 4). Thus, in calculating the avg for Alexander, 78.75 becomes the argument for the ROUND function. That is, 78.75 is rounded to the nearest one unit to get 79. Launch and run the SAS program, and review the output from the PRINT procedure to convince yourself that the exam averages avg are rounded as claimed.
4.4 - Assigning Character Variables
So far, all of our examples have pertained to numeric variables. Now, let's take a look at adding a new character variable to your data set or modifying an existing characteristic variable in your data set. In the previous lessons, we learned how to read the values of a character variable by putting a dollar sign ($) after the variable's name in the INPUT statement. Now, you can update a character variable (or create a new character variable!) by specifying the variable's values in an assignment statement.
Example 4.9
When creating a new character variable in a data set, most often you will want to assign the values based on certain conditions. For example, suppose an instructor wants to create a character variable called status which indicates whether a student "passed" or "failed" based on their overall final grade. A grade below 65, say, might be considered a failing grade, while a grade of 65 or higher might be considered a passing grade. In this case, we would need to make use of an if-then-else statement . We'll learn more about this kind of statement in the next lesson, but you'll get the basic idea here. The following SAS program illustrates the creation of a new character variable called status using an assignment statement in conjunction with an if-then-else statement:
Launch and run the SAS program. Review the output from the PRINT procedure to convince yourself that the values of the character variable status have been assigned correctly. As you can see, to specify a character variable's value using an assignment statement, you enclose the value in quotes. Some comments:
- You can use either single quotes or double quotes. Change the single quotes in the above program to double quotes, and re-run the SAS program to convince yourself that the character values are similarly assigned.
- If you forget to specify the closing quote, it is typically a show-stopper as SAS continues to scan the program looking for the closing quote. Delete the closing quote in the above program, and re-run the SAS program to convince yourself that the program fails to accomplish what is intended. Check your log window to see what kind of a warning statement is generated.
4.5 - Converting Data
Suppose you are asked to calculate sales income using the price and the number of units sold. Pretty straightforward, eh? As long as price and units are stored in your data set as numeric variables, then you could just use the assignment statement:
It may be the case, however, that price and units are instead stored as character variables. Then, you can imagine it being a little odd trying to multiply price by units . In that case, the character variables price and units first need to be converted to numeric variables price and units . How SAS helps us do that is the subject of this section. To be specific, we'll learn how the INPUT function converts character values to numeric values.
The reality though is that SAS is a pretty smart application. If you try to do something to a character variable that should only be done to a numeric variable, SAS automatically tries first to convert the character variable to a numeric variable for you. The problem with taking this lazy person's approach is that it doesn't always work the way you'd hoped. That's why, by the end of our discussion, you'll appreciate that the moral of the story is that it is always best for you to perform the conversions yourself using the INPUT function.
Example 4.11
The following SAS program illustrates how SAS tries to perform an automatic character-to-numeric conversion of standtest and e1 , e2 , e3 , and e4 so that arithmetic operations can be performed on them:
Okay, first note that for some crazy reason, all of the data in the data set have been read in as character data. That is, even the exam scores ( e1 , e2 , e3 , e4 ) and the standardized test scores ( standtest ) are stored as character variables. Then, when SAS goes to calculate the average exam score ( avg ), SAS first attempts to convert e1 , e2 , e3 , and e4 to numeric variables. Likewise, when SAS calculates a new standardized test score ( std ), SAS first attempts to convert standtest to a numeric variable. Let's see how it does. Launch and run the SAS program, and before looking at the output window, take a look at the log window. You should see something that looks like this:
The first NOTE that you see is a standard message that SAS prints in the log to warn you that it performed an automatic character-to-numeric conversion on your behalf. Then, you see three NOTES about invalid numeric data concerning the standtest values 1,210, 1,010, and 1,180. In case you haven't figured it out yourself, it's the commas in those numbers that is throwing SAS for a loop. In general, the automatic conversion produces a numeric missing value from any character value that does not conform to standard numeric values (containing only digits 0, 1, ..., 9, a decimal point, and plus or minus signs). That's why that fifth NOTE is there about missing values being generated. The output itself:
shows the end result of the attempted automatic conversion. The calculation of avg went off without a hitch because e1 , e2 , e3 , and e4 contain standard numeric values, whereas the calculation of std did not because standtest contains nonstandard numeric values. Let's take this character-to-numeric conversion into our own hands.
Example 4.12
The following SAS program illustrates the use of the INPUT function to convert the character variable standtest to a numeric variable explicitly so that an arithmetic operation can be performed on it:
The only difference between the calculation of std here and that in the previous example is that the standtest variable has been inserted here into the INPUT function. The general form of the INPUT function is:
- source is the character variable, constant or expression to be converted to a numeric variable
- informat is a numeric informat that allows you to read the values stored in source
In our case, standtest is the character variable we are trying to convert to a numeric variable. The values in standtest conform to the comma5 . informat, and hence its specification in the INPUT function.
Let's see how we did. Launch and run the SAS program, and again before looking at the output window, take a look at the log window. You should see something that now looks like this:
Ahhaa! No warnings about SAS taking over our program and performing automatic conversions. That's because we are in control this time! Now, looking at the output:
we see that we successfully calculated std this time around. That's much better!
A couple of closing comments. First, I might use our discussion here to add another item to your growing list of good programming practices. Whenever possible, make sure that you are the one who is in control of your program. That is, know what your program is doing at all times, and if it's not doing what you'd expect it to do for all situations , then rewrite it in such a way to make sure that it does.
Second, you might be wondering "geez, we just spent all this time talking about character-to-numeric conversions, but what happens if I have to do a numeric-to-character conversion instead?" Don't worry ... SAS doesn't let you down. If you try to do something to a character variable that should only be done to a numeric variable, SAS automatically tries first to convert the character variable to a numeric variable. If that doesn't work, then you'll want to use the PUT function to convert your numeric values to character values explicitly. We'll address the PUT function in Stat 481 when we learn about character functions in depth.
4.6 - Summary
In this lesson, we learned how to write basic assignment statements, as well as use numeric SAS functions, in order to change the contents of our SAS data set. One thing you might have noticed is that almost all of our examples involved assignment statements that changed every observation in our data set. There may be situations, however, when you don't want to change every observation, but rather want to change just a subset of observations, those that meet a certain condition. To do so, you have to use if-then-else statements, which we'll learn about in the next lesson. In doing so, we'll also learn a few more good programming practices.
The homework for this lesson will give you practice with assignment statements and numeric functions so that you become even more familiar with how they work and can use them in your own SAS programming.

COMMENTS
The assignment statement is used to store a value in a variable. As in most programming languages these days, the assignment statement has the form: <variable>= <expression>; For example, once we have an int variable j, we can assign it the value of expression 4 + 6: int j; j= 4+6; As a convention, we always place a blank after the = sign but ...
An Assignment statement is a statement that is used to set a value to the variable name in a program. Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted.
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
The assignment statement is an instruction that stores a value in a variable. You use this instruction any time you want to update the value of a variable. The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ).
In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location(s) denoted by a variable name; in other words, it copies a value into the variable.In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.. Today, the most commonly used notation for this operation is x = expr (originally Superplan ...
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
The meaning of the first assignment is computing the sum of the value in Counter and 1, and saves it back to Counter. Since Counter 's current value is zero, Counter + 1 is 1+0 = 1 and hence 1 is saved into Counter. Therefore, the new value of Counter becomes 1 and its original value 0 disappears. The second assignment statement computes the ...
The Assignment Statement A variable can be used in an expression like 3.14*r**2. The expression is evaluated and then stored. >>> r = 10 ... Store the result in the variable named on the left hand side. < variable name > = < expression > Naming Variables Rule 1. Name must be comprised of digits, upper
The assignment operator = is used to associate a variable name with a given value. For example, type the command: a=3.45. in the command line window. This command assigns the value 3.45 to the variable named a. Next, type the command: a. in the command window and hit the enter key. You should see the value contained in the variable a echoed to ...
Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You" Assigning a value is known as binding in Python. In the example above, we have assigned the value of 2 to mySecondVariable. Note how I assigned an integer value of 1 and then a string ...
Programs can change the value in a variable. An assignment statement changes the value that is held in a variable. The program uses an assignment statement. The assignment statement puts the value 123 into the variable. In other words, while the program is executing there will be a 64 bit section of memory that holds the value 123. ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
An assignment statement is a line of code that uses a "=" sign. The statement stores the result of an operation performed on the right-hand side of the sign into the variable memory location on the left-hand side. 4. Enter and execute the following lines of Python code in the editor window of your IDE (e.g. Thonny):
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables. 1.4.1. Assignment Statements ... gets the string value you enter as program input and then stores the value in a variable. Run the program a few times, typing in a different name. The code works for any name: behold, the power ...
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java. After a variable is declared, you can assign a value to it by using an assignment statement. In Java, the equal sign = is used as the assignment operator. The syntax for assignment statements is as follows: variable ...
To evaluate an assignment statement: Evaluate the "right side" of the expression (to the right of the equal sign). Once everything is figured out, place the computed value into the variables bucket. More info . We've already seen many examples of assignment. Assignment means: "storing a value (of a particular type) under a variable name".
1.4 — Variable assignment and initialization. Alex April 25, 2024. In the previous lesson ( 1.3 -- Introduction to objects and variables ), we covered how to define a variable that we can use to store values. In this lesson, we'll explore how to actually put values into variables and use those values. As a reminder, here's a short snippet ...
Creating Variables. To use a variable, we must first create one. In Python, we create a variable in a special type of statement called an assignment statement. The basic structure for an assignment statement is a = expression. When our Python interpreter runs this statement, it will first evaluate expression into a single value.
Assignment Statements. You can create your own variables, and give them values, with an assignment statement. The assignment operator is the equals sign ( = ), used like so: >> x = 6 * 7. x = 42. This example creates a new variable named x and assigns it the value of the expression 6 * 7.
Assigning variables. Here's how we create a variable named score in JavaScript: var score = 0; That line of code is called a statement. All programs are made up of statements, and each statement is an instruction to the computer about something we need it to do. Let's add the lives variable: var score = 0; var lives = 3;
Multiple- target assignment: x = y = 75. print(x, y) In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left. OUTPUT. 75 75. 7. Augmented assignment : The augmented assignment is a shorthand assignment that combines an expression and an assignment.
The following SAS program illustrates a very simple assignment statement in which SAS adds up the four exam scores of each student and stores the result in a new numeric variable called examtotal.. DATA grades; input name $ 1-15 e1 e2 e3 e4 p1 f1; * add up each students four exam scores and store it in examtotal; examtotal = e1 + e2 + e3 + e4; DATALINES; Alexander Smith 78 82 86 69 97 80 John ...
When a value such as 6 or 0.355 occurs in a Python program, it is called a number literal. Variable and Types. A variable in Python can store a value of any type. The data type is associated with the value, not the variable. Once a variable is initialized with a value of a particular type, it should always store values of that same type.