- Python »
- 3.12.2 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |

7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment expression like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
For expressions as assignment targets, the annotations are evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
New in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Memory Management in Python
- Ways to increment Iterator from inside the For loop in Python
- How to Break out of multiple loops in Python ?
- Scope Resolution in Python | LEGB Rule
- How to add Python to Windows PATH?
- Benefits of Double Division Operator over Single Division Operator in Python
- Specifying the increment in for-loops in Python
- How to use Variables in Python3?
- Check multiple conditions in if statement - Python
- Difference between dir() and vars() in Python
- Variables under the hood in Python
- Understanding the Execution of Python Program
- Viewing all defined variables in Python
- Convert Python String to Float datatype
- Python Main Function
- What is the difference between Python's Module, Package and Library?
- How to convert Float to Int in Python?
- Context Variables in Python
- Why does Python automatically exit a script when it’s done?
Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Please Login to comment...
Similar reads.
- python-basics
- 10 Best Free Note-Taking Apps for Android - 2024
- 10 Best VLC Media Player Alternatives in 2024 (Free)
- 10 Best Free Time Management and Productivity Apps for Android - 2024
- 10 Best Adobe Illustrator Alternatives in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
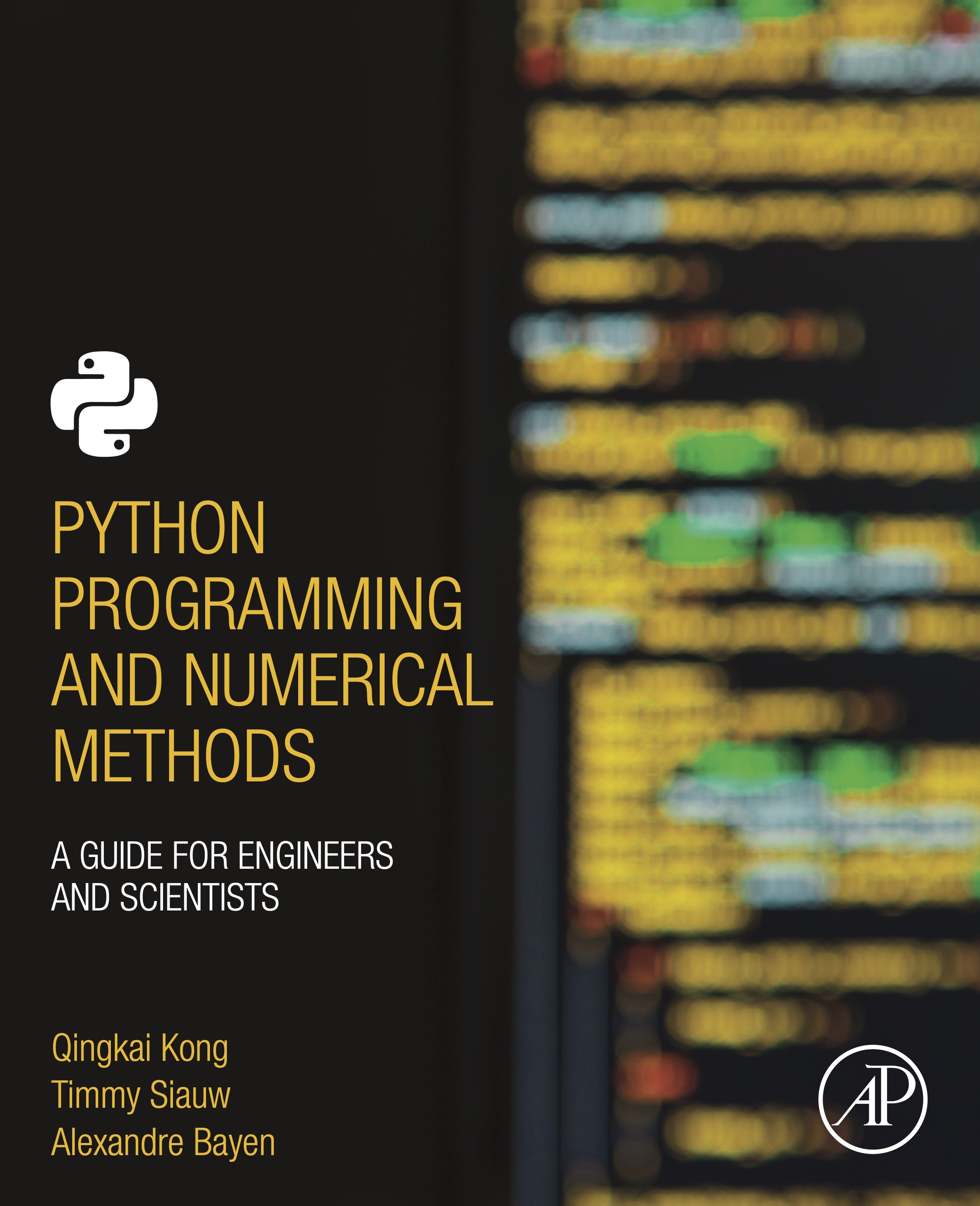
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python assignment operators.
Assignment operators are used to assign values to variables:
Related Pages

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in python.
Last Updated on June 8, 2023 by Prepbytes
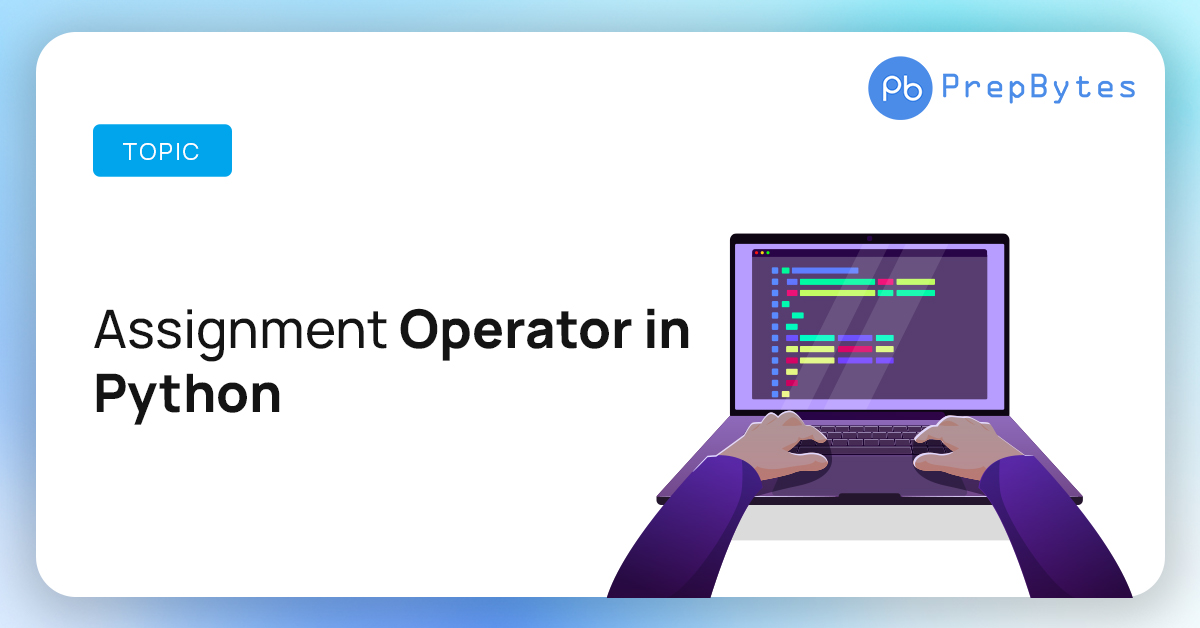
To fully comprehend the assignment operators in Python, it is important to have a basic understanding of what operators are. Operators are utilized to carry out a variety of operations, including mathematical, bitwise, and logical operations, among others, by connecting operands. Operands are the values that are acted upon by operators. In Python, the assignment operator is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is the most commonly used operator in Python. In this article, we will explore the assignment operator in Python, how it works, and its different types.

What is an Assignment Operator in Python?
The assignment operator in Python is used to assign a value to a variable. The assignment operator is represented by the equals sign (=), and it is used to assign a value to a variable. When an assignment operator is used, the value on the right-hand side is assigned to the variable on the left-hand side. This is a fundamental operation in programming, as it allows developers to store data in variables that can be used throughout their code.
For example, consider the following line of code:
Explanation: In this case, the value 10 is assigned to the variable a using the assignment operator. The variable a now holds the value 10, and this value can be used in other parts of the code. This simple example illustrates the basic usage and importance of assignment operators in Python programming.
Types of Assignment Operator in Python
There are several types of assignment operator in Python that are used to perform different operations. Let’s explore each type of assignment operator in Python in detail with the help of some code examples.
1. Simple Assignment Operator (=)
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is:
Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example
Explanation: In this case, the value 25 is assigned to the variable a using the simple assignment operator. The variable a now holds the value 25.
2. Addition Assignment Operator (+=)
The addition assignment operator is used to add a value to a variable and store the result in the same variable. The syntax for the addition assignment operator is:
Here, the value on the right-hand side is added to the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is incremented by 5 using the addition assignment operator. The result, 15, is then printed to the console.
3. Subtraction Assignment Operator (-=)
The subtraction assignment operator is used to subtract a value from a variable and store the result in the same variable. The syntax for the subtraction assignment operator is
Here, the value on the right-hand side is subtracted from the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is decremented by 5 using the subtraction assignment operator. The result, 5, is then printed to the console.
4. Multiplication Assignment Operator (*=)
The multiplication assignment operator is used to multiply a variable by a value and store the result in the same variable. The syntax for the multiplication assignment operator is:
Here, the value on the right-hand side is multiplied by the variable on the left-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is multiplied by 5 using the multiplication assignment operator. The result, 50, is then printed to the console.
5. Division Assignment Operator (/=)
The division assignment operator is used to divide a variable by a value and store the result in the same variable. The syntax for the division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 5 using the division assignment operator. The result, 2.0, is then printed to the console.
6. Modulus Assignment Operator (%=)
The modulus assignment operator is used to find the remainder of the division of a variable by a value and store the result in the same variable. The syntax for the modulus assignment operator is
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the remainder is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the modulus assignment operator. The remainder, 1, is then printed to the console.
7. Floor Division Assignment Operator (//=)
The floor division assignment operator is used to divide a variable by a value and round the result down to the nearest integer, and store the result in the same variable. The syntax for the floor division assignment operator is:
Here, the variable on the left-hand side is divided by the value on the right-hand side, and the result is rounded down to the nearest integer. The rounded result is then stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is divided by 3 using the floor division assignment operator. The result, 3, is then printed to the console.
8. Exponentiation Assignment Operator (**=)
The exponentiation assignment operator is used to raise a variable to the power of a value and store the result in the same variable. The syntax for the exponentiation assignment operator is:
Here, the variable on the left-hand side is raised to the power of the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example
Explanation: In this case, the value of a is raised to the power of 3 using the exponentiation assignment operator. The result, 8, is then printed to the console.
9. Bitwise AND Assignment Operator (&=)
The bitwise AND assignment operator is used to perform a bitwise AND operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise AND assignment operator is:
Here, the variable on the left-hand side is ANDed with the value on the right-hand side using the bitwise AND operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ANDed with 3 using the bitwise AND assignment operator. The result, 2, is then printed to the console.
10. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator is used to perform a bitwise OR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise OR assignment operator is:
Here, the variable on the left-hand side is ORed with the value on the right-hand side using the bitwise OR operator, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is ORed with 3 using the bitwise OR assignment operator. The result, 7, is then printed to the console.
11. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator is used to perform a bitwise XOR operation on the binary representation of a variable and a value, and store the result in the same variable. The syntax for the bitwise XOR assignment operator is:
Here, the variable on the left-hand side is XORed with the value on the right-hand side using the bitwise XOR operator, and the result are stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is XORed with 3 using the bitwise XOR assignment operator. The result, 5, is then printed to the console.
12. Bitwise Right Shift Assignment Operator (>>=)
The bitwise right shift assignment operator is used to shift the bits of a variable to the right by a specified number of positions, and store the result in the same variable. The syntax for the bitwise right shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the right by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Explanation: In this case, the value of a is shifted 2 positions to the right using the bitwise right shift assignment operator. The result, 2, is then printed to the console.
13. Bitwise Left Shift Assignment Operator (<<=)
The bitwise left shift assignment operator is used to shift the bits of a variable to the left by a specified number of positions, and store the result in the same variable. The syntax for the bitwise left shift assignment operator is:
Here, the variable on the left-hand side has its bits shifted to the left by the number of positions specified by the value on the right-hand side, and the result is stored back in the variable on the left-hand side. For example,
Conclusion Assignment operator in Python is used to assign values to variables, and it comes in different types. The simple assignment operator (=) assigns a value to a variable. The augmented assignment operators (+=, -=, *=, /=, %=, &=, |=, ^=, >>=, <<=) perform a specified operation and assign the result to the same variable in one step. The modulus assignment operator (%) calculates the remainder of a division operation and assigns the result to the same variable. The bitwise assignment operators (&=, |=, ^=, >>=, <<=) perform bitwise operations and assign the result to the same variable. The bitwise right shift assignment operator (>>=) shifts the bits of a variable to the right by a specified number of positions and stores the result in the same variable. The bitwise left shift assignment operator (<<=) shifts the bits of a variable to the left by a specified number of positions and stores the result in the same variable. These operators are useful in simplifying and shortening code that involves assigning and manipulating values in a single step.
Here are some Frequently Asked Questions on Assignment Operator in Python:
Q1 – Can I use the assignment operator to assign multiple values to multiple variables at once? Ans – Yes, you can use the assignment operator to assign multiple values to multiple variables at once, separated by commas. For example, "x, y, z = 1, 2, 3" would assign the value 1 to x, 2 to y, and 3 to z.
Q2 – Is it possible to chain assignment operators in Python? Ans – Yes, you can chain assignment operators in Python to perform multiple operations in one line of code. For example, "x = y = z = 1" would assign the value 1 to all three variables.
Q3 – How do I perform a conditional assignment in Python? Ans – To perform a conditional assignment in Python, you can use the ternary operator. For example, "x = a (if a > b) else b" would assign the value of a to x if a is greater than b, otherwise it would assign the value of b to x.
Q4 – What happens if I use an undefined variable in an assignment operation in Python? Ans – If you use an undefined variable in an assignment operation in Python, you will get a NameError. Make sure you have defined the variable before trying to assign a value to it.
Q5 – Can I use assignment operators with non-numeric data types in Python? Ans – Yes, you can use assignment operators with non-numeric data types in Python, such as strings or lists. For example, "my_list += [4, 5, 6]" would append the values 4, 5, and 6 to the end of the list named my_list.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Python list functions & python list methods, python interview questions, namespaces and scope in python, what is the difference between append and extend in python, python program to check for the perfect square, python program to find the sum of first n natural numbers.
What is Assignment Operator in Python?

Before deep-diving into the assignment operators in Python, first, understand what operators are. So operators are used in between the operands to perform various operations, like mathematical operations , bitwise operations , logical operations , and so on. Here, operands are the values on which operators perform the actions. So, assignment operators are used to assigning values to the operands on the left-hand side. Assignment operators assign the right-hand side values to the operand that is present on the left-hand side. The assignment operator in Python is used as the "=" symbol.
Let’s see a very basic example of the assignment operator.
Table Of Assignment Operators
Here we will see different assignment operators in Python with their names, descriptions, and syntax. Let's take them one by one.
Assignment Operator :
This is an assignment operator in Python which assigns the right-hand side expression value to the operand present on the left-hand side.
Sample Code :
Addition Assignment Operator :
This type of assignment operator in Python adds left and right operands, and after that, it assigns the calculated value to the left-hand operand.
Subtraction Assignment Operator :
This operator subtracts the right operand from the left operand and assigns the result to the left operand.
Multiplication Assignment Operator:
This operator will multiply the left-hand side operand by the right-hand side operand and, after that, assign the result to the left-hand operand.
Division Assignment Operator :
This type of assignment operator in Python will divide the left-hand side operand from the right-hand side operand and, after that, assign the result to the left-hand operand.
Modulus Assignment Operator :
This operator will divide the left-hand side operand to the right-hand side operand and, after that, assign the reminder to the left-hand operand.
Click here, to learn more about modulus in python .
Exponentiation Assignment Operator :
This operator raises the left-side operand to the power of the right-side operand and assigns the result to the left-side value.
Floor Division Assignment Operator :
This operator will divide the left operand by the right operand and assign the quotient value (which would be in the form of an integer) to the left operand.
Bitwise AND Assignment Operator :
This operator will perform the bitwise operation on both the left and right operands and, after that, it will assign the resultant value to the left operand.
Bitwise OR Assignment Operator:
This operator will perform the bitwise or operation on both the left and right operands and, after that, it will assign the resultant value to the left operand.
Bitwise XOR Assignment Operator:
This operator will perform the bitwise XOR operation on the left and right operands and, after that, it will assign the resultant value to the left operand.
Bitwise Right Shift Assignment Operator :
This operator will right shift the left operand by the specified position, i.e., b , and after that, it will assign the resultant value to the left operand.
Bitwise Left Shift Assignment Operator:
This operator will left shift the left operand by the specified position, i.e., b , and after that, it will assign the resultant value to the left operand.
- Assignment operators in Python assign the right-hand side values to the operand that is present on the left-hand side.
- The assignment operators in Python is used as the "=" symbol.
- We have different types of assignment operators like +=, -=, \*=, %=, /=, //=, \*\*=, &=, |=, ^=, >>=, <<= .
- All the operators have the same precedence, and hence we prioritise operations based on their associativiy . The associativity is from right to left.
Learn More:
- XOR in Python .
Python Lab Assignments
You can not learn a programming language by only reading the language construct. It also requires programming - writing your own code and studying those of others. Solve these assignments, then study the solutions presented here.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
Python Examples
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution.
All the programs on this page are tested and should work on all platforms.
Want to learn Python by writing code yourself? Enroll in our Interactive Python Course for FREE.
- Python Program to Check Prime Number
- Python Program to Add Two Numbers
- Python Program to Find the Factorial of a Number
- Python Program to Make a Simple Calculator
- Python Program to Print Hello world!
- Python Program to Find the Square Root
- Python Program to Calculate the Area of a Triangle
- Python Program to Solve Quadratic Equation
- Python Program to Swap Two Variables
- Python Program to Generate a Random Number
- Python Program to Convert Kilometers to Miles
- Python Program to Convert Celsius To Fahrenheit
- Python Program to Check if a Number is Positive, Negative or 0
- Python Program to Check if a Number is Odd or Even
- Python Program to Check Leap Year
- Python Program to Find the Largest Among Three Numbers
- Python Program to Print all Prime Numbers in an Interval
- Python Program to Display the multiplication Table
- Python Program to Print the Fibonacci sequence
- Python Program to Check Armstrong Number
- Python Program to Find Armstrong Number in an Interval
- Python Program to Find the Sum of Natural Numbers
- Python Program to Display Powers of 2 Using Anonymous Function
- Python Program to Find Numbers Divisible by Another Number
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal
- Python Program to Find ASCII Value of Character
- Python Program to Find HCF or GCD
- Python Program to Find LCM
- Python Program to Find the Factors of a Number
- Python Program to Shuffle Deck of Cards
- Python Program to Display Calendar
- Python Program to Display Fibonacci Sequence Using Recursion
- Python Program to Find Sum of Natural Numbers Using Recursion
- Python Program to Find Factorial of Number Using Recursion
- Python Program to Convert Decimal to Binary Using Recursion
- Python Program to Add Two Matrices
- Python Program to Transpose a Matrix
- Python Program to Multiply Two Matrices
- Python Program to Check Whether a String is Palindrome or Not
- Python Program to Remove Punctuations From a String
- Python Program to Sort Words in Alphabetic Order
- Python Program to Illustrate Different Set Operations
- Python Program to Count the Number of Each Vowel
- Python Program to Merge Mails
- Python Program to Find the Size (Resolution) of an Image
- Python Program to Find Hash of File
- Python Program to Create Pyramid Patterns
- Python Program to Merge Two Dictionaries
- Python Program to Safely Create a Nested Directory
- Python Program to Access Index of a List Using for Loop
- Python Program to Flatten a Nested List
- Python Program to Slice Lists
- Python Program to Iterate Over Dictionaries Using for Loop
- Python Program to Sort a Dictionary by Value
- Python Program to Check If a List is Empty
- Python Program to Catch Multiple Exceptions in One Line
- Python Program to Copy a File
- Python Program to Concatenate Two Lists
- Python Program to Check if a Key is Already Present in a Dictionary
- Python Program to Split a List Into Evenly Sized Chunks
- Python Program to Parse a String to a Float or Int
- Python Program to Print Colored Text to the Terminal
- Python Program to Convert String to Datetime
- Python Program to Get the Last Element of the List
- Python Program to Get a Substring of a String
- Python Program to Print Output Without a Newline
- Python Program Read a File Line by Line Into a List
- Python Program to Randomly Select an Element From the List
- Python Program to Check If a String Is a Number (Float)
- Python Program to Count the Occurrence of an Item in a List
- Python Program to Append to a File
- Python Program to Delete an Element From a Dictionary
- Python Program to Create a Long Multiline String
- Python Program to Extract Extension From the File Name
- Python Program to Measure the Elapsed Time in Python
- Python Program to Get the Class Name of an Instance
- Python Program to Convert Two Lists Into a Dictionary
- Python Program to Differentiate Between type() and isinstance()
- Python Program to Trim Whitespace From a String
- Python Program to Get the File Name From the File Path
- Python Program to Represent enum
- Python Program to Return Multiple Values From a Function
- Python Program to Get Line Count of a File
- Python Program to Find All File with .txt Extension Present Inside a Directory
- Python Program to Get File Creation and Modification Date
- Python Program to Get the Full Path of the Current Working Directory
- Python Program to Iterate Through Two Lists in Parallel
- Python Program to Check the File Size
- Python Program to Reverse a Number
- Python Program to Compute the Power of a Number
- Python Program to Count the Number of Digits Present In a Number
- Python Program to Check If Two Strings are Anagram
- Python Program to Capitalize the First Character of a String
- Python Program to Compute all the Permutation of the String
- Python Program to Create a Countdown Timer
- Python Program to Count the Number of Occurrence of a Character in String
- Python Program to Remove Duplicate Element From a List
- Python Program to Convert Bytes to a String

IMAGES
VIDEO
COMMENTS
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right. Assignment is defined recursively depending on the form of the target (list).
Here, we will cover Assignment Operators in Python. So, Assignment Operators are used to assigning values to variables. Operator. Description. Syntax = Assign value of right side of expression to left side operand: x = y + z +=
Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms :-. 1. Basic form: Unmute.
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
Python assignment operators facilitate the storage of a value in a variable by assigning the value on the right side of the operator to the variable on the left. This process is vital in programming because it enables developers to save data in variables for later use within their programs. Operator Operation
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
The simple assignment operator is the most commonly used operator in Python. It is used to assign a value to a variable. The syntax for the simple assignment operator is: variable = value. Here, the value on the right-hand side of the equals sign is assigned to the variable on the left-hand side. For example.
So, assignment operators are used to assigning values to the operands on the left-hand side. Assignment operators assign the right-hand side values to the operand that is present on the left-hand side. The assignment operator in Python is used as the "=" symbol. Let's see a very basic example of the assignment operator.
If one line code is definitely going to happen for you, Python 3.8 introduces assignment expressions affectionately known as "the walrus operator". someBoolValue and (num := 20) The 20 will be assigned to num if the first boolean expression is True .
Also, try to solve Python list Exercise. Exercise 7: Return the count of a given substring from a string. Write a program to find how many times substring "Emma" appears in the given string. Given: str_x = "Emma is good developer. Emma is a writer" Code language: Python (python) Expected Output: Emma appeared 2 times
Python Exercises, Practice, Solution: Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
PEP 572 seeks to add assignment expressions (or "inline assignments") to the language, but it has seen a prolonged discussion over multiple huge threads on the python-dev mailing list—even after multiple rounds on python-ideas. Those threads were often contentious and were clearly voluminous to the point where many probably just tuned them out.
It contains Python function assignments, programs, questions, and challenges. Total 10 questions. The solution is provided for all questions and tested on Python 3. This function exercise covers questions on concepts such as function creation, function calls, function arguments, inner functions, and built-in functions.
python programs with output for class 12 and 11 students. Simple Assignements are for beginners starting from basics hello world program to game development using class and object concepts. A list of assignment solutions with source code are provided to learn concept by examples in an easy way.
All Examples. Files. Python Program to Print Hello world! Python Program to Add Two Numbers. Python Program to Find the Square Root. Python Program to Calculate the Area of a Triangle. Python Program to Solve Quadratic Equation. Python Program to Swap Two Variables. Python Program to Generate a Random Number.
In simpler form, this is also commonly used to designate a default... In fact, they produce concise one-line expressions that help to eliminate line noise from the code. This behavior is the basis for a form of the if/else ternary operator: A = Y if X else Z. edited Jan 5, 2012 at 19:40. answered Jan 5, 2012 at 19:12. joaquin. 84.3k 31 140 153.
Subtracts a value from the variable and assigns the result to that variable.
So always use the == operator to check if any two Python objects have the same value. 4. Tuple Assignment and Mutable Objects . If you're familiar with built-in data structures in Python, you know that tuples are immutable. So you cannot modify them in place. Data structures like lists and dictionaries, on the other hand, are mutable.
sums.append(int(match.group(1))) Python for Everybody assignment 11.1 The actual goal is to read a file, look for integers using the re.findall() looking for a regular expression of [0-9]+, then converting the extracted strings to integers and finally summing up the integers. Pycharm is returning: TypeError: int () argument must be a string, a ...