Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types

JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Booleans
JavaScript Bitwise Operators
- JavaScript Object.is()
JavaScript Ternary Operator
- JavaScript typeof Operator
- What is an Operator?
In JavaScript, an operator is a special symbol used to perform operations on operands (values and variables ). For example,
Here + is an operator that performs addition, and 2 and 3 are operands.
- JavaScript Operator Types
Here is a list of different operators you will learn in this tutorial.
- Assignment Operators
- Arithmetic Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Other Operators
- JavaScript Assignment Operators
Assignment operators are used to assign values to variables. For example,
Here, the = operator is used to assign value 5 to variable x .
Here's a list of commonly used assignment operators:
Note: The commonly used assignment operator is = . You will understand other assignment operators such as += , -= , *= etc. once we learn arithmetic operators.
- JavaScript Arithmetic Operators
Arithmetic operators are used to perform arithmetic calculations . For example,
Here, the + operator is used to add two operands.
Example 1: Arithmetic operators in JavaScript
To learn about the increment and decrement operators, visit ++ and -- operator .
Note : The ** operator was introduced in ECMAScript 2016 and some browsers may not support them. To learn more, visit JavaScript exponentiation browser support .
- JavaScript Comparison Operators
Comparison operators compare two values and return a boolean value, either true or false . For example,
Here, the comparison operator > is used to compare whether a is greater than b .
Example 2: Comparison operators in JavaScript
Comparison operators are used in decision-making and loops.
Note: To learn more about comparison operators, visit JavaScript Comparison and Logical Operators
- JavaScript Logical Operators
Logical operators perform logical operations and return a boolean value, either true or false . For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the result is true .
Example 3: Logical Operators in JavaScript
Logical operators are used in decision making and loops. You will learn about the use of logical operators in detail in later tutorials.
Bitwise operators perform operations on binary representations of numbers.
Bitwise operators are rarely used in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
- JavaScript String Operators
In JavaScript, you can also use the + operator to concatenate (join) two or more strings .
Example 4: String operators in JavaScript
Note: When + is used with strings, it performs concatenation. However, when + is used with numbers, it performs addition.
- Other JavaScript Operators
Here's a list of other operators available in JavaScript. You will learn about these operators in later tutorials.
- JavaScript typeof
Table of Contents
Video: javascript operators.
Sorry about that.
Related Tutorials
JavaScript Tutorial
- Skip to main content
- Select language
- Skip to search
- Comparison operators
Non-identity / strict inequality (!==)
Less than or equal operator (<=).
JavaScript has both strict and type–converting comparisons. A strict comparison (e.g., === ) is only true if the operands are of the same type and the contents match. The more commonly-used abstract comparison (e.g. == ) converts the operands to the same type before making the comparison. For relational abstract comparisons (e.g., <= ), the operands are first converted to primitives, then to the same type, before comparison.
Strings are compared based on standard lexicographical ordering, using Unicode values.
Features of comparisons:
- Two strings are strictly equal when they have the same sequence of characters, same length, and same characters in corresponding positions.
- Two numbers are strictly equal when they are numerically equal (have the same number value). NaN is not equal to anything, including NaN. Positive and negative zeros are equal to one another.
- Two Boolean operands are strictly equal if both are true or both are false .
- Two distinct objects are never equal for either strict or abstract comparisons.
- An expression comparing Objects is only true if the operands reference the same Object.
- Null and Undefined Types are strictly equal to themselves and abstractly equal to each other.
Equality operators
Equality (==).
The equality operator converts the operands if they are not of the same type , then applies strict comparison. If both operands are objects , then JavaScript compares internal references which are equal when operands refer to the same object in memory.
Inequality (!=)
The inequality operator returns true if the operands are not equal. If the two operands are not of the same type , JavaScript attempts to convert the operands to an appropriate type for the comparison. If both operands are objects , then JavaScript compares internal references which are not equal when operands refer to different objects in memory.
Identity / strict equality (===)
The identity operator returns true if the operands are strictly equal (see above) with no type conversion .
The non-identity operator returns true if the operands are not equal and/or not of the same type .
Relational operators
Each of these operators will call the valueOf() function on each operand before a comparison is made.
)"> Greater than operator (>)
The greater than operator returns true if the left operand is greater than the right operand.
)"> Greater than or equal operator (>=)
The greater than or equal operator returns true if the left operand is greater than or equal to the right operand.
Less than operator (<)
The less than operator returns true if the left operand is less than the right operand.
The less than or equal operator returns true if the left operand is less than or equal to the right operand.
Using the Equality Operators
The standard equality operators ( == and != ) use the Abstract Equality Comparison Algorithm to compare two operands. If the operands are of different types, it will attempt to convert them to the same type before making the comparison, e.g., in the expression 5 == '5' , the string on the right is converted to Number before the comparison is made.
The strict equality operators ( === and !== ) use the Strict Equality Comparison Algorithm and are intended for performing equality comparisons on operands of the same type. If the operands are of different types, the result is always false so 5 !== '5' .
Use strict equality operators if the operands must be of a specific type as well as value or if the exact type of the operands is important. Otherwise, use the standard equality operators, which allow you to compare the identity of two operands even if they are not of the same type.
When type conversion is involved in the comparison (i.e., non–strict comparison), JavaScript converts the types String , Number , Boolean , or Object operands as follows:
- When comparing a number and a string, the string is converted to a number value. JavaScript attempts to convert the string numeric literal to a Number type value. First, a mathematical value is derived from the string numeric literal. Next, this value is rounded to nearest Number type value.
- If one of the operands is Boolean , the Boolean operand is converted to 1 if it is true and +0 if it is false .
- If an object is compared with a number or string, JavaScript attempts to return the default value for the object. Operators attempt to convert the object to a primitive value, a String or Number value, using the valueOf and toString methods of the objects. If this attempt to convert the object fails, a runtime error is generated.
- Note that an object is converted into a primitive if, and only if, its comparand is a primitive. If both operands are objects, they're compared as objects, and the equality test is true only if both refer the same object.
Specifications
Browser compatibility.
- Object.is()
- Math.sign()
- Equality comparisons and sameness
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Arithmetic operators
- Array comprehensions
- Assignment operators
- Bitwise operators
- Comma operator
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
JavaScript Comparison Operators – How to Compare Objects for Equality in JS
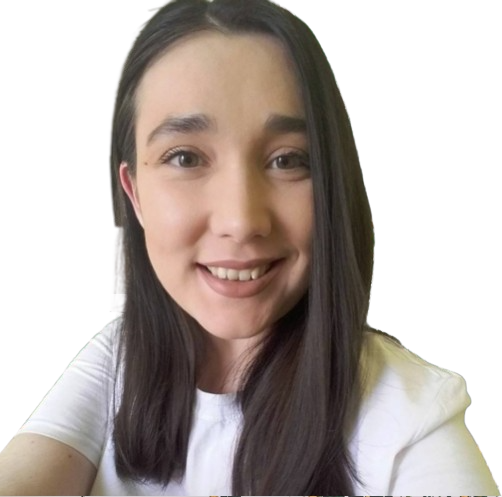
While coding in JavaScript, there may be times when you need to compare objects for equality. The thing is, comparing objects in JavaScript is not that straightforward.
In this article, you learn three ways to compare objects for equality in JavaScript.
Let's get into it!
What's the Difference Between Comparing Primitive Data Types VS Non-Primitive Data Types in JavaScript?
Data types in JavaScript fall into one of two categories:
- Primitive (such as Number, String, Boolean, Undefined, Null, Symbol)
- Non-primitive (such as Object)
Primitive data types refer to a single value, and comparing primitive values is relatively straightforward – you only need to use any of the comparison operators.
In the following example, I use the strict equality operator, === , which checks if the two operands are equal and returns a Boolean as a result:
You can also assign the value of the variable a to another variable, a1 , and compare them:
In the example above, both variables point to the same value, so the result is true .
When it comes to objects, however, comparing them isn't that straightforward.
Even though both objects have the same key and value pairs, the result of the comparison is false . Why is that?
Is it because I used the strict equality operator, === ? What happens if I use the loose quality operator, == ?
I get the same result!
Both a and b are seemingly the same, yet the objects are not equal when I use === or == .
You would think that two objects with the same properties and properties with the same values would be considered equal.
The reason for this result has to do with how JavaScript approaches testing for equality when it comes to comparing primitive and non-primitive data types.
The difference between primitive and non-primitive data types is that:
- primitive data types are compared by value .
- non-primitive data types are compared by reference .
In the following sections, you will see some ways to compare objects for equality.
How to Compare Objects by Reference in JavaScript
As you saw from the example in the section above, using == and === returns false when you try to compare objects by value:
Both objects have identical keys and values, but the result was false because they are different instances.
To compare objects by reference, you have to test whether both point to the same location in memory.
When referring to an object, you refer to an address in memory.
Let's see an example:
In the example above, thanks to the line let b = a; , both variables have the same reference and point to the same object instance, so the result is true .
When I assign the variable a to the b , the address of a gets copied to b . This results in both having the same address – not the same value.
With that said, most of the time, you will want to compare objects by value and not by instance.
And as you saw, you can't just use == or === to compare objects by value – it requires a bit more work.

How to Compare Objects Using The JSON.stringify() Function in JavaScript
One way you can compare two objects by value is by using the JSON.stringify function.
The JSON.stringify() function converts objects into equivalent JSON strings. You can then use any of the comparison operators to compare the strings.
The JSON.stringify() function converted both objects into JSON strings, and since both a and b have the same properties and values, the result is true .
But JSON.stringify() isn't always the best solution for comparing objects by value since it has limitations.
First of all, when using JSON.stringify() , the order of the keys matters.
Look at what happens when the keys are in a different order:
In this example, you would expect the result to be true since the values are the same – only the order of the keys got reversed.
JSON.stringify() stringifies the object as it is, so the order of the keys is important. If they are not in the same order, the result will be false .
So, JSON.stringify() is not the best choice for comparing objects since you can't always be certain of the order of the keys.
There is also an additional limitation: JSON doesn't represent all types.
Look at what happens when the value of one key is undefined:
In the example above, the result is unexpected. The result should have been false but returned as true because JSON ignores keys whose values are undefined.
How to Compare Objects Using the Lodash _.isEqual() Method in JavaScript
An elegant and sophisticated solution for comparing objects by their value is to use the well-tested JavaScript library Lodash and its _.isEqual() method.
Let's take the example from the previous section, where the keys have the same value but are in a different order, and use the _.isEqual() method:
In the previous section, the result when using JSON.stringify was false .
The Lodash library offers a variety of edge cases and performs a deep comparison between two values to check if the two objects are deeply equal.
In this article, you learned how to compare objects for equality in JavaScript.
You saw three different ways and the pros and cons of each. When in doubt, the most effective way to compare objects is by using the Lodash library.
Thank you for reading, and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Home » JavaScript Tutorial » JavaScript Comparison Operators
JavaScript Comparison Operators
Summary : in this tutorial, you will learn how to use JavaScript comparison operators to compare two values.
Introduction to JavaScript comparison operators
To compare two values, you use a comparison operator. The following table shows the comparison operators in JavaScript:
A comparison operator returns a Boolean value indicating whether the comparison is true or not. See the following example:
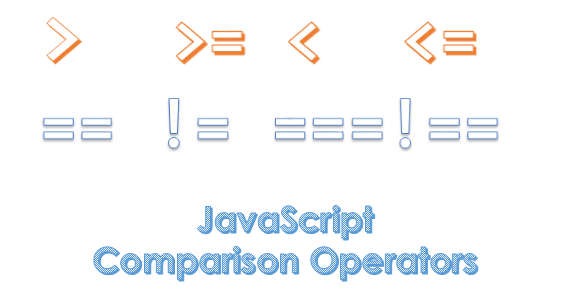
A comparison operator takes two values. If the types of values are not comparable, the comparison operator converts them into values of comparable types according to specific rules.
Compare numbers
If values are numbers, the comparison operators perform a numeric comparison. For example:
This example is straightforward. The variable a is 10 , b is 20 . The expression a >= b expression returns false and the expression a == 10 expression returns true .
Compare strings
If the operands are strings, JavaScript compares the character codes numerically one by one in the string.
Because JavaScript compares the character codes in the strings numerically, you may receive an unexpected result, for example:
In this example, f2 is less than f1 because the letter B has the character code 66 while the letter a has the character code 97 .
To fix this, you need to:
- First, convert the strings into a common format, either lowercase or uppercase
- Second, compare the converted values
For example:
Note that the toLowerCase() is a method of the String object that converts the string to lowercase.
Compare a number with a value of another type
If one value is a number and the other is not, the comparison operator will convert the non-numeric value into a number and compare them numerically. For example:
In this example, the comparison operator converts the string '20' into the number 20 and compares with the number 10. Here is an example:
In this example, the comparison operator converts the string '10' into the number 10 and compares them numerically.
Compare an object with a non-object
If a value is an object, the valueOf() method of that object is called to return the value for comparison. If the object doesn’t have the valueOf() method, the toString() method is called instead. For example:
In this first comparison, the apple object has the valueOf() method that returns 10 . Therefore, the comparison operator uses the number 10 for comparison.
In the second comparison, JavaScript first calls the valueOf() method. However, the orange object doesn’t have the valueOf() method. So JavaScript calls the toString() method to get the returned value of 20 for comparison.
Compare a Boolean with another value
If a value is a Boolean value, JavaScript converts it to a number and compares the converted value with the other value; true is converted to 1 and false is converted to 0 . For example:
In addition to the above rules, the equal ( == ) and not equal ( != ) operators also have the following rules.
Compare null and undefined
In JavaScript, null equals undefined . It means that the following expression returns true .
Compare NaN with other values
If either value is NaN , then the equal operator( == ) returns false .
The not-equal ( != ) operator returns true when comparing the NaN with another value:
Strict equal ( === ) and not strict equal ( !== )
Besides the comparison operators above, JavaScript provides strict equal ( === ) and not strict equal ( !== ) operators.
The strict equal and not strict equal operators behave like the equal and not equal operators except that they don’t convert the operand before comparison. See the following example:
In the first comparison, since we use the equality operator, JavaScript converts the string into a number and performs the comparison.
However, in the second comparison, we use the strict equal operator ( === ), JavaScript doesn’t convert the string before comparison, therefore the result is false .
In this tutorial, you have learned how to use the JavaScript comparison operators to compare values.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- JavaScript Arithmetic Unary Negation(-) Operator
- JavaScript Pipeline Operator
- JavaScript Instanceof Operator
- JavaScript Arithmetic Unary Plus(+) Operator
- JavaScript Remainder(%) Operator
- Operator precedence in JavaScript
- What does !== undefined mean in JavaScript ?
- JavaScript in Operator
- JavaScript Arithmetic Operators
- JavaScript Ternary Operator
- JavaScript Comma Operator
- JavaScript Remainder Assignment(%=) Operator
- JavaScript Assignment Operators
- How to get negative result using modulo operator in JavaScript ?
- What is (~~) "double tilde" operator in JavaScript ?
- How to access object properties from result returned by async() function in JavaScript ?
- What does OR Operator || in a Statement in JavaScript ?
- What is JavaScript >>> Operator and how to use it ?
- Explain the purpose of the ‘in’ operator in JavaScript
JavaScript Comparison Operators
Comparison operators.
The comparison operators are used to check if a condition is true or false.
Comparison operators are used in logical expressions to determine their equality or differences in variables or values.
Comparison Operators list
There are many comparison operators as shown in the table with the description.
Equality Operator (==)
The Equality operator is used to compare the equality of two operands. If equal then the condition is true otherwise false.
Example: Below example illustrates the (==) operator in JavaScript.
Inequality Operator(!=)
The Inequality Operator is used to compare the inequality of two operands. If equal then the condition is false otherwise true.
Example: Below examples illustrate the (!=) operator in JavaScript.
Strict equality Operator(===)
The Strict equality Operator is used to compare the equality of two operands with type. If both value and type are equal then the condition is true otherwise false.
Example: Below examples illustrate the (===) operator in JavaScript.
Strict inequality Operator (!==)
The Strict inequality Operator is used to compare the inequality of two operands with type. If both value and type are not equal then the condition is true otherwise false.
Example: Below examples illustrate the (!==) operator in JavaScript.
Greater than Operator(>)
The Greater than Operator is used to check whether the left-side value is greater than the right-side value. If the value is greater then the condition is true otherwise false.
Example: Below examples illustrate the (>) operator in JavaScript.
Greater than or equal Operator(>=)
The Greater than or equal Operator is used to check whether the left side operand is greater than or equal to the right side operand. If the value is greater than or equal then the condition is true otherwise false.
Example: Below examples illustrate the (>=) operator in JavaScript.
Less than Operator(<)
The Less than Operator is used to check whether the left-side value is less than the right-side value. If yes then the condition is true otherwise false.
Example: Below examples illustrate the (<) operator in JavaScript.
Less than or equal Operator(<=)
The Less than or equal Operator is used to check whether the left side operand value is less than or equal to the right side operand value. If yes then the condition is true otherwise false.
Example: Below examples illustrate the (<=) operator in JavaScript.
Supported Browsers: The browsers supported by all JavaScript Comparison operators are listed below:
- Google Chrome
- Microsoft Edge
- Internet Explorer
We have a complete list of Javascript operators, to check those please go through this Javascript Operators Complete reference article.
We have a Cheat Sheet on Javascript where we covered all the important topics of Javascript to check those please go through Javascript Cheat Sheet-A Basic guide to JavaScript .
Please Login to comment...
- javascript-operators
- Web Technologies
- 10 Best HuggingChat Alternatives and Competitors
- Best Free Android Apps for Podcast Listening
- Google AI Model: Predicts Floods 7 Days in Advance
- Who is Devika AI? India's 'AI coder', an alternative to Devin AI
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Is this AI? See if you can spot the technology in your everyday life.
Tell us what is ai and see how your answers compare to the experts.

Artificial intelligence is suddenly everywhere. Fueled by huge technological advances in recent years and gobs of venture capitalist money , AI has become one of the hottest corporate buzzwords.
Roughly 1 in 7 public companies mentioned “artificial intelligence” in their annual filings last year, according to a Washington Post analysis . But the term is fuzzy.
“AI is purposefully ill-defined from a marketing perspective,” said Alex Hanna, director of research at Distributed AI Research Institute. It “has been composed of wishful thinking and hype from the beginning.”
So what is AI, really? To cut through the hype, we asked 16 experts to judge 10 everyday technologies. Try to spot the AI for yourself and see how you compare to readers and the experts.
Is this AI?
Chatbots like ChatGPT
Could be AI
Definitely AI
Experts said
Readers said
We’ll start with an easy one. The viral chatbot responds to a user’s prompt by systematically churning out words, producing a surprisingly coherent but not always accurate reply.
Most experts said that the tool is definitely AI, Things are about to get even murkier.
Auto-correct on mobile phones
Most experts said auto-correct could be considered artificial intelligence, and many said it definitely is AI.
Earlier versions of auto-correct guessed what you meant to type by comparing the locations of the keys you pressed with a dictionary of popular words. But the latest versions also consider the context surrounding a word to better predict what you meant, using the same technique at the core of chatbots. Hint: You’ll see this technology again.
Tap-to-pay credit cards
Not quite. No expert considered tap-to-pay credit cards to be AI. The system uses radio waves to transmit payment information. It sure seems fancy, but it’s not AI.
Google Translate
Eleven of 16 experts surveyed said Google Translate is definitely AI.
Modern translation services don’t simply look up words in a dictionary and return the foreign language match. Rather, the system uses terabytes of multilingual data to build a model of connections between words and their context.
Sound familiar? This technique, called a transformer model , also underlies chatbots and recent auto-correct systems. It provides a more natural, though not always accurate, translation.
Personalized ads
A majority of experts felt it could be AI.
The defining feature of artificial intelligence is that “behavior is learned from data rather than explicitly programmed,” said Matthew Carrigan, a machine-learning engineer at Hugging Face.
For a non-AI approach, a programmer might write specific rules, like, “If a user is a 30-year-old male, show them sports ads.” But AI uses machine learning to create its own rules by analyzing large amounts of data. This enables incredibly specific connections, but it can be difficult to understand how a program drew its conclusions.
Computer opponents in video games
You’re not alone. Few experts today definitely consider video game opponents to be AI.
Chess has long been a proving ground for AI research. AI researchers often test programs through games because they have well-defined rules with clear victors and losers.
In 1980, a Carnegie Mellon professor offered a $100,000 prize for the first computer program to defeat a world chess champion. Seventeen years later, IBM’s Deep Blue beat Garry Kasparov, cementing its place in the history of artificial intelligence.
Beating a world chess champion is no longer impressive. Today’s chess engines dominate all human players.
GPS directions
Turn-by-turn navigation uses a defined set of instructions to search though a database of road networks to quickly find the best route.
How much that route-finding technique just carries out preset rules left experts divided on whether GPS could be considered AI. You said
Facial recognition software, like Apple Face ID
The majority of experts consider facial recognition software to definitely be AI, but you said
The technology maps the precise geometry of people’s facial features. Facial recognition has prompted concern from privacy experts as its use is adopted by governments, especially because the technology is less accurate at identifying people with darker skin.
Apple’s Face ID feature, which lets users unlock their devices by looking at them, projects infrared dots on a face and uses a neural network — a mathematical system that acts like a computerized brain — to determine whether the face matches.
Microsoft's Clippy
You’re in the minority on this one. Most experts said Clippy is not AI, although 1 in 4 said it could at least be considered AI.
The animated paper clip assistant, launched with Microsoft Office 97, offered tips based on what the user was doing, like offering to help format a letter after “Dear Kevin,” was typed. But the widely panned feature was a far cry from intelligence. Microsoft turned it off by default in 2001 and launched a self-aware ad campaign that Clippy was out of work.
Virtual voice assistants, like Alexa or Siri
Just over half of experts consider a virtual voice assistant to definitely be AI.
It can process words as they’re said and handle requests using machine learning and neural networks, which many experts say have become synonymous with artificial intelligence.
Even among experts, what counts as artificial intelligence is fuzzy.
“The term ‘AI’ has become so broadly used in practice that … it’s almost always better to use a more specific term,” said Nicholas Vincent, an assistant professor at Simon Fraser University.
Nothing was unanimously deemed AI by experts, and few products were definitely declared not AI. Most landed somewhere in the middle.
What readers and experts consider to be AI
Some experts don’t think anything we use today is AI. Current technology is “capable of specific tasks they are trained for but dysfunctional at unforeseen events,” said Pruthuvi Maheshakya Wijewardena, a data and applied scientist at Microsoft, who identified no product as definitely AI.
The “capabilities of an AI is a spectrum, and we are still at the lower end,” said Maheshakya Wijewardena.
For Emily M. Bender, a professor of linguistics at the University of Washington, calling anything AI is “a way to dodge accountability” for its creators.
What artificial intelligence generates, whether it’s auto-correct, chatbots or photos, is trained from large amounts of data, often pulled off the internet . When that data is flawed, inaccurate or offensive, the results can reflect — and even amplify — those flaws.
The term AI makes “the machines sound like autonomous thinking entities rather than tools that are created and used by people and companies,” said Bender.
About this story
Emma Kumer contributed to this story.
The experts surveyed were Emily M. Bender, professor, University of Washington; Matthew Carrigan, machine-learning engineer, Hugging Face; Yali Du, lecturer, King’s College London; Hany Farid, professor, UC Berkeley; Florent Gbelidji, machine-learning engineer, Hugging Face; Alex Hanna, director of research, Distributed AI Research Institute; Nathan Lambert, research scientist, Allen Institute for AI; Pablo Montalvo, machine-learning engineer, Hugging Face; Alvaro Moran, machine-learning engineer, Hugging Face; Chinasa T. Okolo, fellow, Center for Technology Innovation at the Brookings Institution; Giada Pistilli, principal ethicist, Hugging Face; Daniela Rus, director, MIT Computer Science & Artificial Intelligence Laboratory; Mahesh Sathiamoorthy, formerly of Google DeepMind; Luca Soldaini, senior applied research scientist, Allen Institute for AI; Nicholas Vincent, assistant professor, Simon Fraser University; and Pruthuvi Maheshakya Wijewardena, data and applied scientist, Microsoft.

IMAGES
VIDEO
COMMENTS
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
When comparing a string with a number, JavaScript will convert the string to a number when doing the comparison. An empty string converts to 0. A non-numeric string converts to NaN which is always false. When comparing two strings, "2" will be greater than "12", because (alphabetically) 1 is less than 2.
Comparisons. We know many comparison operators from maths. In JavaScript they are written like this: Greater/less than: a > b, a < b. Greater/less than or equals: a >= b, a <= b. Equals: a == b, please note the double equality sign == means the equality test, while a single one a = b means an assignment.
Expressions and operators. This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. A complete and detailed list of operators and expressions is also available in the reference.
Note: In JavaScript, == is a comparison operator, whereas = is an assignment operator. If you mistakenly use = instead of == , you might get unwanted result. Example 2: Not Equal to Operator
JavaScript Assignment Operators. Assignment operators are used to assign values to variables. For example, const x = 5; Here, ... JavaScript Comparison Operators. Comparison operators compare two values and return a boolean value, either true or false. For example, const a = 3, b = 2; console.log(a > b); // true ...
Conclusion. In this tutorial, you've learned the 7 types of JavaScript operators: Arithmetic, assignment, comparison, logical, ternary, typeof, and bitwise operators. These operators can be used to manipulate values and variables to achieve a desired outcome. Congratulations on finishing this guide!
JavaScript has both strict and type-converting comparisons. A strict comparison (e.g., ===) is only true if the operands are of the same type and the contents match.The more commonly-used abstract comparison (e.g. ==) converts the operands to the same type before making the comparison.For relational abstract comparisons (e.g., <=), the operands are first converted to primitives, then to the ...
One way you can compare two objects by value is by using the JSON.stringify function. The JSON.stringify() function converts objects into equivalent JSON strings. You can then use any of the comparison operators to compare the strings. let a = { name: 'Dionysia', age: 29}; let b = { name: 'Dionysia', age: 29};
Javascript operators are used to perform different types of mathematical and logical computations. Examples: The Assignment Operator = assigns values. The Addition Operator + adds values. The Multiplication Operator * multiplies values. The Comparison Operator > compares values
= is the assignment operator. It sets a variable (the left-hand side) to a value (the right-hand side). The result is the value on the right-hand side. == is the comparison operator. It will only return true if both values are equivalent after coercing their types to the same type. === is a more strict comparison operator often called the ...
A comparison operator returns a Boolean value indicating whether the comparison is true or not. See the following example: let r1 = 20 > 10; // true let r2 = 20 < 10; // false let r3 = 10 == 10; // true Code language: JavaScript (javascript) A comparison operator takes two values. If the types of values are not comparable, the comparison ...
Welcome to my video on "Assignment and Comparison Operators in JavaScript"! In this tutorial, we will be exploring two fundamental concepts in JavaScript: as...
Comparison Operators list. There are many comparison operators as shown in the table with the description. OPERATOR NAME. USAGE. OPERATION. Equality Operator. a==b. Compares the equality of two operators. Inequality Operator.
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.
React is stewarded by Facebook (Meta) and it was created way back in 2013. React is very popular, as you can see by its weekly downloads shown in Table 1. This popularity makes it the default ...
Fueled by huge technological advances in recent years and gobs of venture capitalist money, AI has become one of the hottest corporate buzzwords. Roughly 1 in 7 public companies mentioned ...
Javascript objects comparison and assignment. Ask Question Asked 6 years, 1 month ago. Modified 6 years, 1 month ago. ... b = {} Now, I want to be able to compare and assign a and b (like a=b) in such a way, that whichever property b holds it updates in a, and if it doesn't hold any property, a remains the same. eg.
Is it possible in JavaScript to assign values to multiple variables while comparing inline (the ? operator) ? Here is a dumbed down version of a non working code I wished would work. var toto = true; var test0, test1; toto ? test0 = 'test0', test1 = 'test1' : null;