The ternary operator in Golang
In most of the programming languages, there is an operator (?:) called ternary operator which evaluates like an if-else chain will do. But, Go does not have a ternary operator . In this post, we will dive into why it is absent in Go.

What is the ternary operator?
The ternary operator is the ?: operator used for the conditional value. Here is an example of how one would use it in code.
This operator is absent in Golang.
Why is it absent in Go?
The reason is a simple design choice. The operator although once understood, is an easy one is in fact a complicated construct for someone who is new to code. The Go programming language chose the simple approach of if-else. This is a longer version of the operator, but it is more readable .
The solution
The solution is obviously an if-else block. It represents the same code in a much cleaner way. Here is a simple if-else block representing the above expression.
The solution is verbose but it is a design choice made by the Go creators. One of the reasons being overuse and abuse of that operator to create extremely complex code that is hard to read. This decision improves over it and forces a cleaner and readable approach over that.
How to Use Conditions in Go
Deciding to take one action or another is a fundamental building block in programming. Discover how conditional statements make this possible in Go.
Conditional statements allow you to execute decisions based on logic that evaluates to true or false. Some examples of conditional statements include if statements, if…else, if…else if.. else, and switch-case statements. Go has full support for these conditionals with a familiar and straightforward syntax.
Getting Started With Golang
To follow along with this tutorial, install Go in your local environment if you haven't already. You can also test the code snippets in this article using the Go playground .
If Statement
The if statement executes a block of code only if a certain condition is met. Here is the syntax:
Here is an example that prints “Pass” if the value of the marks variable is more than 50:
You could also declare the marks variable in the if statement like this:
When combining conditions, Go allows you to use logical operators familiar from other languages like AND(&&), OR (||), and NOT(!).
The AND operator returns true only if the value on its right and left are true:
The OR operator returns true if one of the values either on the right or left is true:
The NOT operator returns true only if the value is false:
If…Else Statement
The if…else statement executes the corresponding code depending on whether the condition is met.
In the example below, the program prints ‘Pass’ if the total marks value is above 50 and ‘Fail’ if it’s below.
If…Else If…Else Statement
The if…else if…else statement allows you to combine multiple if statements.
Extending the if…else example, the program below also checks whether the marks are above 80 and prints “Passed with distinction” if so:
Switch Statement
The switch statement is a conditional statement that allows you to execute different actions based on the value of an expression. You can identify significant values as “cases” and act on them accordingly.
The switch statement in Go is slightly different from in other programming languages like C#, JavaScript, and Python. This is because it only executes the block of code under the met case. The break keyword, required by other languages, isn't necessary here:
The above code evaluates the expression after the switch keyword, then compares it to each case value. If a value matches, the following block runs. You can have as many cases as you need, but they must all be unique. The default block runs if there is no match.
The following example uses a switch statement to display a “todo” item for each day of the week.
Here, Go’s time package provides the day of the week as an integer and, depending on that value, the switch statement prints a certain task.
When to Use Conditional Statements
Conditional statements help you create a decision flow in your program. Like many other languages, Go supports several types of conditional statement. You can use them to streamline your program and ensure its logical operation is correct.
Use conditional statements when the flow of your program depends on a certain value such as user input.
Go if..else, if..else..if, nested if with Best Practices
Antony Shikubu
August 3, 2022
Introduction
In computer programming, conditional statements help you make decisions based on a given condition. The conditional statement evaluates if a condition is true or false, therefore it is worth noting that if statements work with boolean values. Just like other programming languages, Go has its own construct for conditional statements. In this tutorial , we will learn about different types of conditional statements in Go.
Below are the topics that we will cover
- If statement
- If - Else statement
- If -else if else statement
- Nested If statement
- Logical operators AND , OR and NOT
- Using multiple conditions if if else
Supported Comparison Operators in GO
Before we go ahead learning about golang if else statement, let us be familiar with supported comparison operators as you may need to use them in the if else conditions for decision making:
if statement
If a statement is used to specify a block of code that should be executed if a certain condition is true .
Explanation
In the above syntax, the keyword if is used to declare the beginning of an if statement, followed by a condition that is being tested. The condition should be a value of boolean type , the the block of code between opening and closing curly brackets will be executed only if the condition is true . In the next example, we define code that will only run if age is above 18 years.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to true because 19 is greater than 18. The code block will be executed because the condition is true .
One liner if statement
We can also convert our if condition to run in one line, for example here I have written the same if condition in 2 different ways, one is multi line while the other is using single line:
if else Statement
If Else statement is used to evaluate both conditions that might be true or false. In the previous example, we did not really care if a condition evaluates to false. We use If Else conditional statements to handle both true and false outcomes.
In the preceding example, we define the age and ageLimit variables. In the if statement we compare the value of age and ageLimit by checking which is greater. The statement age > ageLimit will evaluate to false because 12 is less than 18. The second code block will be executed because the condition is false .
if else if statement
If Else If statement is used to specify a new condition if the first condition is false. When using If Else If statement, you can nest as many If Else conditions as you desire.
In the above example, the first if statement evaluates to false because age (17) is not greater than ageLimit(18) . We then check again if age is equal to 17, which evaluates to true . The block of code inside this section will be executed.
Nested if Statement
Nested if Statement, is a If conditional statement that has an If statement inside another If statement. The nested If statement will always be executed only if the outer If statement evaluates to true.
In the above syntax, the second if statement with condition labeled conditionY is housed inside the outer If statement with condition labeled conditionX . As long as condition labeled conditionX is true , if conditionY will be executed.
Supported Logical Operators in GO
We also have some logical operators on golang which help us when we have to check for multiple conditions:
if else statements using logical operator
In Go we use logical operators together with If conditional statements to achieve certain goals in our code. Logical operators return/execute to boolean values, hence making more sense using them conditional statements. Below are definitions of the three logical operators in Go.
Using logical AND operator
Checks of both operands are none zero. In case both operands are none-zero, a true value will be returned, else a false value will be returned. The operator representing logical AND is && .
Using logical OR operator
Check if one operand is true. In case one operand is true code will be executed. The operator representing logical OR is || .
Using logical NOT operator
Reverses the logical state of its operands. If a condition is true, the logical operator NOT will change it to false. The operator representing logical Not is ! .
Using multiple condition in golang if else statements
We can also add multiple conditional blocks inside the if else statements to further enhance the decision making. Let us check some more examples covering if else multiple condition:
if statement with 2 logical OR Operator
Here we are checking if our num is more than 100 or less than 900:
if statement with 3 logical OR Operator
In this example we add one more OR condition to the if condition:
Using both logical AND and OR operator in single if statement
We can also use both AND and OR operator inside a single if statement, here is an example:
Using multiple conditions with if..else..if..else statement
Now we have used multiple conditions in our if condition but you can use the same inside else..if condition or combine it with if..else..if statements.
In Go, we use conditional statements to handle decisions precisely in code. They enable our code to perform different computations or actions depending on whether a condition is true or false . In this article we have learned about different if conditional statements like If, If Else, If Else If Else, Nested If statements
https://go.dev/tour/flowcontrol https://www.golangprograms.com/golang-if-else-statements.html
Related Keywords: golang if else shorthand, golang if else one line, golang if else multiple conditions, golang if else string, golang if true, golang if else best practices, golang if statement with assignment, golang if not
He is highly skilled software developer with expertise in Python, Golang, and AWS cloud services. Skilled in building scalable solutions, he specializes in Django, Flask, Pandas, and NumPy for web apps and data processing, ensuring robust and maintainable code for diverse projects. You can connect with him on Linkedin .
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
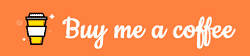
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!

Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Programming Languages
Exam certifications.
Certified Kubernetes Application Developer (CKAD)
CompTIA PenTest+ (PT0-002)
Red Hat EX407 (Ansible)
Hacker Rank Python
Privacy Policy
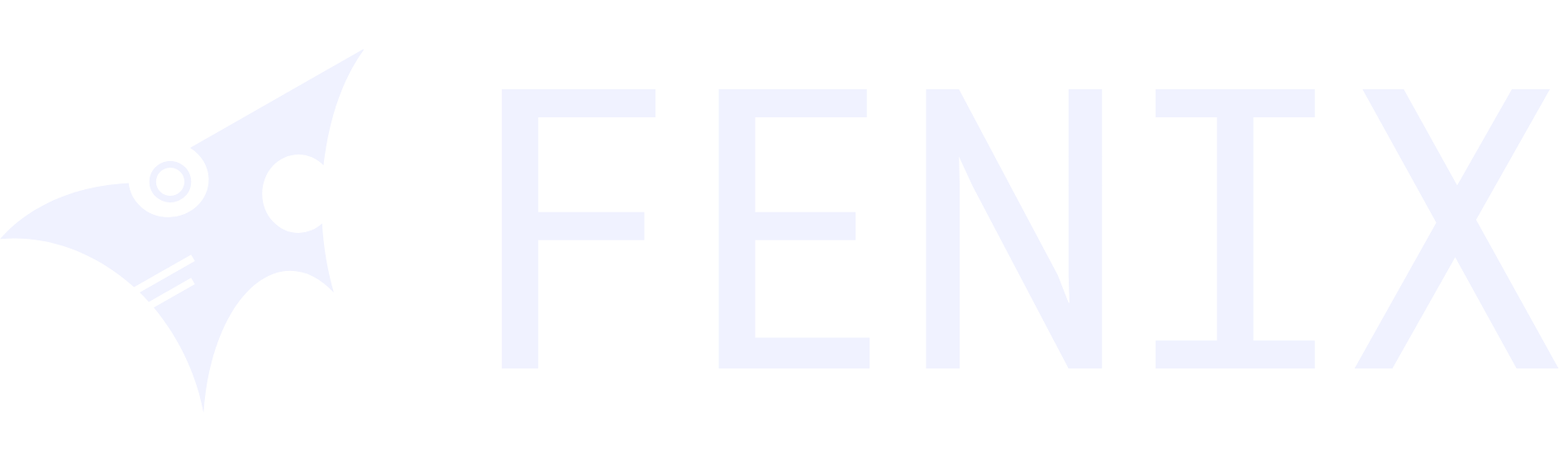
Golang Tutorial 4: Conditional Statements
Conditional statements are fundamental control flow constructs in programming languages, allowing developers to make decisions based on certain conditions. In GoLang, conditional statements provide a structured approach to executing code paths based on the evaluation of Boolean expressions. By effectively utilizing conditional statements, developers can create robust and flexible applications that respond to different scenarios and user inputs.
The if Statement: The Basic Conditional Structure
The if statement is the most basic conditional structure in GoLang. It allows developers to execute a block of code if a given condition is true. The condition is typically a Boolean expression that evaluates to either true or false .
The if-else Statement: Handling Alternative Scenarios
The if-else statement extends the if statement by providing an alternative block of code to execute if the initial condition is false. This allows developers to handle different scenarios based on the evaluation of a single condition.
Multiple Conditions with if-else Chains
Conditional statements can be nested to handle multiple conditions and execute different code paths based on the evaluation of a series of Boolean expressions. This allows developers to create more complex decision-making logic.
The switch Statement: Handling Multiple Options
The switch statement provides a more concise and efficient way to handle multiple conditions and select different code paths based on the value of an expression. It is particularly useful for handling cases where the expression can take on multiple distinct values.
Conditional Expressions: Combining Conditions
Conditional expressions allow developers to combine multiple conditions into a single expression, reducing the need for nested if-else statements. This can make code more concise and easier to read.
Conditional statements are essential tools for controlling the flow of execution in GoLang applications. They enable developers to make decisions based on user inputs, evaluate conditions, and execute different code paths based on various scenarios. By mastering the use of conditional statements, GoLang developers can create robust and flexible applications that adapt to different situations and user interactions.
You might also like...
Unveiling the power of grpc in golang: building robust, healthy microservices with an edge over http, unleashing the power of graphql with golang, naming conventions in golang: a comprehensive guide, writing idiomatic golang code, indentation and formatting in golang.
Programming-Idioms
- C++
- Or search :
Idiom #252 Conditional assignment
Assign to the variable x the string value "a" if calling the function condition returns true , or the value "b" otherwise.
- View revisions
- Successive conditions
- for else loop
- Report a bug
Please choose a nickname before doing this
No security, no password. Other people might choose the same nickname.

Golang: Conditionals and Loops
Techstructive Blog @MeetGor21
Introduction
Moving to the fourth part, we will be doing conditional statements and loops in golang. We will be seeing the basics of conditional statements like if-else and switch along with loops like for, while, and range-based loops. We won't be covering iterating over arrays in a loop as this requires an understanding of arrays.
Conditional statements
Conditional statements are quite a fundamental aspect of learning a programming language. In golang, we have if-else conditional statements as well as switch cases. We will be exploring both of them in this section. Firstly, we will dive into if-else statements which are quite easy to understand.
An if statement is used for checking the validity of a condition. If the condition is true(condition is met), a particular sets of statements are executed else (condition is not satisfied) different statements gets executed. We can use a basic if-else statement in go with the following syntax:
We can also use else if for evaluating more than one condition rather than using nested if and else.
Using else if we can evaluate multiple conditions. This style is much better than using nested if else statements as it becomes harder to read for complex cases.
We also have switch statements in golang which allow us to write cases for a given state of a variable. We can simply add cases for a given variable, the case should be a valid value that the variable can take. If a case is matched it breaks out of the switch statement without executing any statements below the matched case.
The basic structure of the switch statements in golang is as follows:
This gives a good understanding of switch-case statements. We can give a variable to the switch statement and pick its value in the respective case statements to evaluate the result accordingly. The default statement is evaluated when there is no match among the given cases.
This code will by default pick javascript and developer as the values for language and devs respectively if there is no match for the provided language or the language is left empty.
We also have fallthrough in the golang switch which allows evaluating more than one case if one of them is matched. This will allow the switch and check for all the cases sequentially and evaluate all the matched and satisfied cases.
So, here we can see that the fallthrough hits multiple cases. This is unlike the base case which exits the switch statement once a case has been satisfied. This can be helpful for situations where you really want to evaluate multiple conditions for a given variable.
We can now move on to loops in golang. We only have a for loop so to speak but this can be used as any other looping statement like the while loop or range-based loop. We will first see the most fundamental loop statement in golang which is a three-component loop.
We can have a simple for loop in golang by using the three statements namely initialize , condition , and increment . The structure of the loop is quite similar to the other programming languages.
Range-based loop
We can even iterate over a string, using the range keyword in golang. We need to have two variables for using a range-based for loop in golang one is the index or the 0 based position of the element and the copy of the element in the array or string. Using the range keyword, we can iterate over the string one by one.
So, here we can see we have iterated over the string by each character. Using the range keyword in golang, The i, s is the index and the copy of the element at that index as discussed earlier. Using the index we get the value which we don't have to index the array for accessing it, that is already copied in the second variable while using the range loop.
while loop (Go's while is for)
There are no while loops as such in golang, but the for loop can also work similarly to the while loop. We can use a condition just after the for a keyword to make it act like a while loop.
We can see here that the condition is evaluated and the statements in the loop body are executed, if the condition evaluates to false, the flow is moved out of the loop and we exit the loop.
Infinite loop
We can run an infinite loop again using a keyword. We do not have any other keywords for loops in golang.
This might be used with a base condition to exit the loop otherwise there should be a memory overflow and the program will exit with errors.
If we want to exit out of a loop unconditionally, we can use the break keyword. This will break the loop and help us to exit out of an infinite or a condition-bound-based loop too.
As, we can see inside an infinite loop, we were able to break out of it by using a conditional statement and break keyword. This also applies to switch cases it basically is the opposite of fallthrough in switch-case statements. But by default(without using fallthrough), the case statement breaks the switch after a match has been found or the default case has been encountered.
We also have the opposite of break i.e. continue which halts the execution of the loop and directs back to the post statement increment(in case of for loops) or evaluation(in case of while loop). We basically are starting to iterate over the loop again after we encounter the continue but by preserving the counter/iterator state values.
For following up with the code for this and all parts of the series, head over to the 100 days of Golang GitHub repository.
So, from this section, we were able to understand the basics of conditional statements and loops in golang. We covered the things which are more important for understanding the core of the language than some specific things. There are certain parts that need to be explored further like iterating over arrays and slices which we'll cover after we have understood arrays and slices. Hopefully, you have understood the basics of the conditional statements and loops in golang. Thank you for reading, if you have any questions, or feedback please let me know in the comments or social handles. Until next time, Happy Coding :)
Django + Auth0 Quick Setup
Django Basics: Setup and Installation
If else statement
Welcome to tutorial number 8 of our Golang tutorial series .
if is a statement that has a boolean condition and it executes a block of code if that condition evaluates to true . It executes an alternate else block if the condition evaluates to false . In this tutorial, we will look at the various syntaxes and ways of using if statement.
If statement syntax
The syntax of the if statement is provided below
If the condition is true, the lines of code between the braces { and } is executed.
Unlike in other languages like C, the braces { } are mandatory even if there is only one line of code between the braces { } .
Let’s write a simple program to find out whether a number is even or odd.
Run in Playground
In the above program, the condition num%2 in line no. 9 finds whether the remainder of dividing num by 2 is zero or not. Since it is 0 in this case, the text The number 10 is even is printed and the program returns.
The if statement has an optional else construct which will be executed if the condition in the if statement evaluates to false .
In the above snippet, if condition evaluates to false , then the lines of code between else { and } will be executed.
Let’s rewrite the program to find whether the number is odd or even using if else statement.
Run in playground
In the above code, instead of returning if the condition is true as we did in the previous section , we create an else statement that will be executed if the condition is false . In this case, since 11 is odd, the if condition is false and the lines of code within the else statement is executed. The above program will print.
If … else if … else statement
The if statement also has optional else if and else components. The syntax for the same is provided below
The condition is evaluated for the truth from the top to bottom.
In the above statement if condition1 is true, then the lines of code within if condition1 { and the closing brace } are executed.
If condition1 is false and condition2 is true, then the lines of code within else if condition2 { and the next closing brace } is executed.
If both condition1 and condition2 are false, then the lines of code in the else statement between else { and } are executed.
There can be any number of else if statements.
In general, whichever if or else if ’s condition evaluates to true , it’s corresponding code block is executed. If none of the conditions are true then else block is executed.
Let’s write a program that uses else if .
In the above program, the condition else if num >= 51 && num <= 100 in line no. 11 is true and hence the program will print
If with assignment
There is one more variant of if which includes an optional shorthand assignment statement that is executed before the condition is evaluated. Its syntax is
In the above snippet, assignment-statement is first executed before the condition is evaluated.
Let’s rewrite the program which finds whether the number is even or odd using the above syntax.
In the above program num is initialized in the if statement in line no. 8. One thing to be noted is that num is available only for access from inside the if and else . i.e. the scope of num is limited to the if else blocks. If we try to access num from outside the if or else , the compiler will complain. This syntax often comes in handy when we declare a variable just for the purpose of if else construct. Using this syntax in such cases ensures that the scope of the variable is only within the if else statement.
The else statement should start in the same line after the closing curly brace } of the if statement. If not the compiler will complain.
Let’s understand this by means of a program.
In the program above, the else statement does not start in the same line after the closing } of the if statement in line no. 11. Instead, it starts in the next line. This is not allowed in Go. If you run this program, the compiler will output the error,
The reason is because of the way Go inserts semicolons automatically. You can read about the semicolon insertion rule here https://golang.org/ref/spec#Semicolons .
In the rules, it’s specified that a semicolon will be inserted after closing brace } , if that is the final token of the line. So a semicolon is automatically inserted after the if statement’s closing braces } in line no. 11 by the Go compiler.
So our program actually becomes
after semicolon insertion. The compiler would have inserted a semicolon in line no. 4 of the above snippet.
Since if{...} else {...} is one single statement, a semicolon should not be present in the middle of it. Hence this program fails to compile. Therefore it is a syntactical requirement to place the else in the same line after the if statement’s closing brace } .
I have rewritten the program by moving the else after the closing } of the if statement to prevent the automatic semicolon insertion.
Now the compiler will be happy and so are we 😃.
Idiomatic Go
We have seen various if-else constructs and we have in fact seen multiple ways to write the same program. For example, we have seen multiple ways to write a program that checks whether the number is even or odd using different if else constructs. Which one is the idiomatic way of coding in Go? In Go’s philosophy, it is better to avoid unnecessary branches and indentation of code. It is also considered better to return as early as possible. I have provided the program from the previous section below,
The idiomatic way of writing the above program in Go’s philosophy is to avoid the else and return from the if if the condition is true.
In the above program, as soon as we find out the number is even, we return immediately. This avoids the unnecessary else code branch. This is the way things are done in Go 😃. Please keep this in mind whenever writing Go programs.
I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter or LinkedIn . Have a good day.
If you would like to advertise on this website, hire me, or if you have any other software development needs please email me at naveen[at]golangbot[dot]com .
Next tutorial - Loops
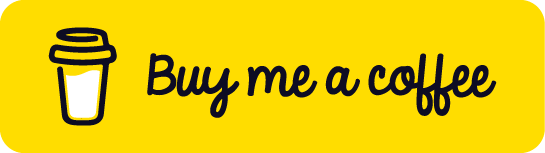
In Golang we have if-else statements to check a condition and execute the relevant code.
if statement
The if statement is used to check if a condition is met and execute the code inside the if block only if the condition is met.
The general syntax is
Let us write a example code to test if we need to take an umbrella based on if it's raining or not outside.
else statement
If the condition in a if statement is not met then we execute the else block if it is defined.
The syntax is
One thing to remember here is the else statement should always be in the same line as the closing } of the if statement.
In the last module, we checked if it's raining and we gave a message if it is raining. But our code doesn't print anything if it is not raining. Let us fix it by adding an else statement and providing more information.
Let's write an example code to check if the user's name is John or not
You can cascade if statement in an else statement to check for more conditions and run the relevant code.
Now let's write some code to check if a user age is below 20 or between 20 and 60 or above 60.
The if statement also provides an option to initialize a variable and test the condition within the if statement. The general syntax in this case is
An example code is given below.
In a country, a person is allowed to vote if his/her age is above or equal to 18. Check the userAge variable and print "Eligible" if the person is eligible to vote or "Not Eligible" if the person is not eligible.
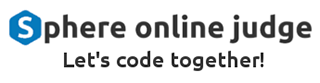
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Package conditional is go/golang replacement for ternary if/else operator
mileusna/conditional
Folders and files, repository files navigation, go/golang replacement for ternary if/else operator.
Package conditinal is go/golang replacement for ternary if/else operator
Many languages have ternary operator which makes it easy and short to assign value inline, for example
Unfortunately, there is no ternary testing operator in Go so the shortest way to write this code in go would be
or if you prefere perhapse more readable but longer version
This is where conditional package steps in. It provides fuctions that replaces ternary operator for each basic type in go. We can now write conditional assignment in only one Go line:
Package conditional also provides fuctions for all Go basic types

IMAGES
VIDEO
COMMENTS
The C conditional expression (commonly known as the ternary operator) has three operands: expr1 ? expr2 : expr3. If expr1 evaluates to true, expr2 is evaluated and is the result of the expression. Otherwise, expr3 is evaluated and provided as the result. This is from the ANSI C Programming Language section 2.11 by K&R.
If Statements. We will start with the if statement, which will evaluate whether a statement is true or false, and run code only in the case that the statement is true. In a plain text editor, open a file and write the following code: import "fmt" func main() {. grade := 70 if grade >= 65 {.
The ternary operator is the ?: operator used for the conditional value. Here is an example of how one would use it in code. 1. v = f > 0 ? 1 : 0 // if f > 0 then v is 1 else v is 0. This operator is absent in Golang.
Here is the syntax: Here is an example that prints "Pass" if the value of the marks variable is more than 50: fmt.Println( "Pass") You could also declare the marks variable in the if statement like this: fmt.Println( "Pass") When combining conditions, Go allows you to use logical operators familiar from other languages like AND (&&), OR ...
In computer programming, conditional statements help you make decisions based on a given condition. The conditional statement evaluates if a condition is true or false, therefore it is worth noting that if statements work with boolean values. Just like other programming languages, Go has its own construct for conditional statements.
Usually the If/else/else if condition when written with one condition makes the program lengthy and increases the complexity thus we can combine two conditions. The conditions can be combined in the following ways :-. Using && (AND) Operator. The And (&&) is a way of combining conditional statement has the following syntax:
Conditional statements are fundamental control flow constructs in programming languages, allowing developers to make decisions based on certain conditions. In GoLang, conditional statements provide a structured approach to executing code paths based on the evaluation of Boolean expressions. By effectively utilizing conditional statements, developers can create robust and flexible applications
Idiom #252 Conditional assignment. Assign to the variable x the string value "a" if calling the function condition returns true, or the value "b" otherwise. x = "a". x = "b".
Introduction. Conditional statements give programmers the ability to direct their programs to take some action if a condition is true and another action if the condition is false. Frequently, we want to compare some variable against multiple possible values, taking different actions in each circumstance. It's possible to make this work using if statements alone.
Introduction. Moving to the fourth part, we will be doing conditional statements and loops in golang. We will be seeing the basics of conditional statements like if-else and switch along with loops like for, while, and range-based loops. We won't be covering iterating over arrays in a loop as this requires an understanding of arrays.
After the execution of post statement, condition statement will be evaluated again. If condition returns true, code inside for loop will be executed again else for loop terminates. Let's get ...
Then you can also declare methods for NormalResult which will be promoted to AdminResult as well: func (r *NormalResult) doSomething() {. // Doing something. } Edit. And, no, it is not possible to have conditional types in Go as you suggested. A variable can only be of one type, be it NormalResult, AdminResult or interface{}
If with a short statement. Like for, the if statement can start with a short statement to execute before the condition.. Variables declared by the statement are only in scope until the end of the if. (Try using v in the last return statement.) < 6/14 >
Welcome to tutorial number 8 of our Golang tutorial series. if is a statement that has a boolean condition and it executes a block of code if that condition evaluates to true. It executes an alternate else block if the condition evaluates to false. In this tutorial, we will look at the various syntaxes and ways of using if statement.
else statement. If the condition in a if statement is not met then we execute the else block if it is defined. The syntax is. if <condition> { // code executes if condition is true } else { // code executes if condition is false } One thing to remember here is the else statement should always be in the same line as the closing } of the if ...
If you're still set on assignment in the conditional (e.g., if you've declared err previously above) then: ... So, it helps to know how Golang scopes work. An if statement would create a block scoped variable, only accessible from within the statements block. If you had to do something with "fp" in your example you couldn't unless it ...
14. No. Only one 'simple statement' is permitted at the beginning of an if-statement, per the spec. The recommended approach is multiple tests which might return an error, so I think you want something like: genUri := buildUri() if err := setRedisIdentity(genUri, email); err != nil {. return "", err.
Package conditional is go/golang replacement for ternary if/else operator License. MIT license 8 stars 0 forks Branches Tags Activity. Star ... It provides fuctions that replaces ternary operator for each basic type in go. We can now write conditional assignment in only one Go line: val:= conditional. String (x == y, "Value OK", "Value ...
Inside a function, the := short assignment statement can be used in place of a var declaration with implicit type. Outside a function, every statement begins with a keyword ( var, func, and so on) and so the := construct is not available. < 10/17 >. short-variable-declarations.go Syntax Imports.
9. According to the golang the documentation: An identifier declared in a block may be redeclared in an inner block. That's exactly what your example is showing, a is redeclared within the brackets, because of the ':=', and is never used. A solution is to declare both variable and then use it: var a, b int. {.