- The Book of C (version 2022.08) »
- 4. Control Structures

4. Control Structures ¶
In this chapter, we encounter the set of "control" structures in C, such as conditional statements and looping constructs. As a preview, the control structures in C are nearly identical to those found in Java (since Java syntax is heavily based on C), and bear strong resemblance to control structures found in other programming languages.
4.1. if Statement ¶
Both an if and an if-else are available in C. The <expression> can be any valid C expression. The parentheses around the expression are required, even if it is just a single variable:
You should always use curly braces around the statement block associated with an if statement. Although C allows a programmer not to include the curly braces in the case of if statements and other control structures when there is only a single statement in the statement block, you should always use the curly braces . Why, you ask, should I type those additional characters? Just ask the unfortunate engineering group at Apple that introduced the "goto fail" bug into their SSL (secure sockets layer) library: a bug that affected the macOS and iOS operating systems quite severely [ 1 ] . The upshot of this security failure is that it could have been prevented with the rigorous use of curly braces for all statement blocks.
So, for the simple form of the if statement shown above, you should really write:
As in Java, the else keyword can be used to provide alternative execution for a conditional expression. Also similar to Java, multiple if ... else if statements can be chained together. There is no elif as in Python (or elsif as in Ruby).
4.2. The conditional expression (ternary operator) ¶
The conditional expression can be used as a shorthand for some if-else statements. The general syntax of the conditional operator is:
This is an expression , not a statement , so it represents a value. The operator works by evaluating expression1 . If it is true (non-zero), it evaluates and returns expression2 . Otherwise, it evaluates and returns expression3 .
The classic example of the ternary operator is to return the smaller of two variables. Instead of writing:
you can write:
The ternary operator is viewed by some programmers as "excessively tricky" since expressions with such operators can be hard to read. Use your best judgment, and don't do something this [Horrific] example.
4.3. switch statement ¶
The switch statement is a sort of specialized form of if with the goal of efficiently separating different blocks of code based on the value of an integer. The switch expression is evaluated, and then the flow of control jumps to the matching const-expression case . The case expressions are typically int or char constants (unfortunately, you cannot use strings as case expressions). The switch statement is probably the single most syntactically awkward and error-prone feature of the C language:
Each constant needs its own case keyword and a trailing colon (:). Once execution has jumped to a particular case, the program will keep running through all the cases from that point down --- this so called fall through operation is used in the above example so that expression-3 and expression-4 run the same statements. The explicit break statements are necessary to exit the switch . Omitting the break statements is a common error --- it compiles, but leads to inadvertent, unexpected, and likely erroneous fall-through behavior.
Why does the switch statement fall-through behavior work the way it does? The best explanation might be that C was originally developed for an audience of assembly language programmers. The assembly language programmers were used to the idea of a "jump table" with fall-through behavior, so that's the way C does it (it's also relatively easy to implement it this way). Unfortunately, the audience for C is now quite different, and the fall-through behavior is widely regarded as an unfortunate part of the language.
4.4. while loop ¶
The while loop evaluates the test expression before every loop, so it can execute zero times if the condition is initially false. The conditional expression requires parentheses like the if:
Although the curly braces are not technically required if there is only one statement in the body of the while loop, you should always use the curly braces. Again, see [ 1 ] for why.
4.5. do-while loop ¶
Like a while loop, but with the test condition at the bottom of the loop. The loop body will always execute at least once. The do-while tends to be an unpopular area of the language. Although many users of C use the straight while if possible, a do-while loop can be very useful in some situations:
4.6. for loop ¶
The for loop in C contains three components that are often used in looping constructs, making it a fairly convenient statement to use. The three parts are an initializer, a continuation condition, and an action, as in:
The initializer is executed once before the body of the loop is entered. The loop continues to run as long as the continuation condition remains true. After every execution of the loop, the action is executed. The following example executes 10 times by counting 0..9. Many loops look very much like the following:
C programs often have series of the form 0..(some_number-1). It's idiomatic in C for loops like the example above to start at 0 and use < in the test so the series runs up to but not equal to the upper bound. In other languages you might start at 1 and use <= in the test.
Each of the three parts of the for loop can be made up of multiple expressions separated by commas. Expressions separated by commas are executed in order, left to right, and represent the value of the last expression.
Note that in the C standard prior to C99, it was illegal to declare a variable in the initializer part of a for loop. In C99, however, it is perfectly legal. If you compile the above code using gcc without the -std=c99 flag, you will get the following error:
Once the -std=c99 flag is added, the code compiles correctly, as expected.
4.6.1. break ¶
The break statement causes execution to exit the current loop or switch statement. Stylistically speaking, break has the potential to be a bit vulgar. It is preferable to use a straight while with a single conditional expression at the top if possible, but sometimes you are forced to use a break because the test can occur only somewhere in the midst of the statements in the loop body. To keep the code readable, be sure to make the break obvious --- forgetting to account for the action of a break is a traditional source of bugs in loop behavior:
The break does not work with if ; it only works in loops and switches. Thinking that a break refers to an if when it really refers to the enclosing while has created some high-quality bugs. When using a break , it is nice to write the enclosing loop to iterate in the most straightforward, obvious, normal way, and then use the break to explicitly catch the exceptional, weird cases.
4.6.2. continue ¶
The continue statement causes control to jump to the bottom of the loop, effectively skipping over any code below the continue . As with break , this has a reputation as being vulgar, so use it sparingly. You can almost always get the effect more clearly using an if inside your loop:
4.6.3. Statement labels and goto ¶
Continuing the theme of statements that have a tendency of being a bit vulgar, we come to the king of vulgarity, the infamous goto statement [Goto] . The structure of a goto statement in C is to unconditionally jump to a statement label, and continue execution from there. The basic structure is:
The goto statement is not uncommon to encounter in operating systems code when there is a legitimate need to handle complex errors that can happen. A pattern that you might see is something like:
Notice the structure above: there are multiple steps being performed to carry out some initialization for an operation [ 2 ] . If one of those initialization operations fails, code execution transfers to a statement to handle deinitialization , and those de-init operations happen in reverse order of initialization . It is possible to rewrite the above code to use if / else structures, but the structure becomes much more complex (see an exercise below). Although goto has the reputation of leading to "spaghetti code", judicious use of this statement in situations like the above makes for cleaner and clearer code.
Rewrite the goto example code above (the last code example, above) to use if / else instead. Which code do you think exhibits a more clear structure?
Consider the following program snippet:
What is printed if the number 3 is entered?
Say that you want to write a program that repeatedly asks for a snowfall amount, and that you want to keep asking for another value until the sum of all values exceeds a certain value. What control structure would work best to facilitate entry of the snowfall values, and why?
Say you want to write a program that computes the average of quiz scores. You have a big stack of quizzes, so you do not know the number of quizzes up front. What control structure would work best to facilitate entry of the scores, and why?
Say that you want to simulate rolling a die (singular of dice) a fixed number of times and to compute and print the average value for the die rolls. What control structure would work best for this problem, and why?
http://thedailywtf.com/articles/One-Bad-Ternary-Operator-Deserves-Another
Dijkstra. Letters to the editor: go to statement considered harmful. Communications of the ACM, Volume 11, Issue 3, March 1968. https://dl.acm.org/citation.cfm?id=362947
Table of Contents
- 4.1. if Statement
- 4.2. The conditional expression (ternary operator)
- 4.3. switch statement
- 4.4. while loop
- 4.5. do-while loop
- 4.6.1. break
- 4.6.2. continue
- 4.6.3. Statement labels and goto
Previous topic
3. Basic Types and Operators
5. Arrays and Strings
Quick search
Got issues.
If you find a bug or have a suggestion for improvement, please report it .

- Deep Learning
CONTROL STRUCTURES IN C
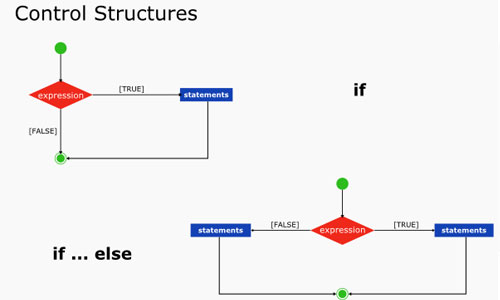
Control Structures specifies one or more conditions to be evaluated or tested by the program, along with a statement or statements to be executed if the condition is true and optionally, other statements to be executed if the condition is false.
See blow image to understand control (Decision making ) structure:-
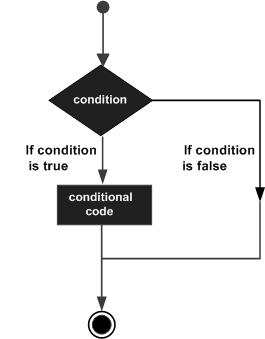
C programming language assumes any zero and null values as false , and if it is either non-zero or non-null , then it is assumed as true value.
C programming language provides the following types of decision making statements.
Table of Contents
If Statement OR If-else Statements:
Both an ‘if’ and ‘if-else’ are available in C. The <expression> can be any valid expression. The parentheses around the expression are not required, if it is just a single expression.
Conditional Expression -or- The Ternary Operator:
The conditional expression can be used as a shorthand for some if-else statement. The general syntax of the conditional operator is:
<expression1> ? <expression2> : <expression3>
Switch Statement:
The switch statement is a sort of specialized form of if used to efficiently separate different block of code based on the value of an integer. The case expressions are typically ‘int’ or ‘char’ constants, The switch statement is probably the single most syntactically awkward and error-prone features of the C language.
switch (<expression>) { case <const-expression-1>: <statement> break; case <const-expression-2>: <statement> break; case <const-expression-3>: // here we combine case 3 and 4 case <const-expression-4>: <statement> break; default: // optional <statement> Each constant needs its own case keyword and a trailing colon (:). Once execution has jumped to a particular case, the program will keep running through all the cases from that point down— this so called “fall through” operation is used in the above example so that expression-3 and expression-4 run the same statements. The explicit break statements are necessary to exit the switch.
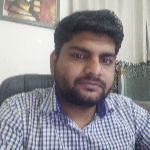
Pradeep Maurya is the Professional Web Developer & Designer and the Founder of “Tutorials website” . He lives in Delhi and loves to be a self-dependent person. As an owner, he is trying his best to improve this platform day by day. His passion, dedication and quick decision making ability to stand apart from others. He’s an avid blogger and writes on the publications like Dzone , e27.co
Related posts
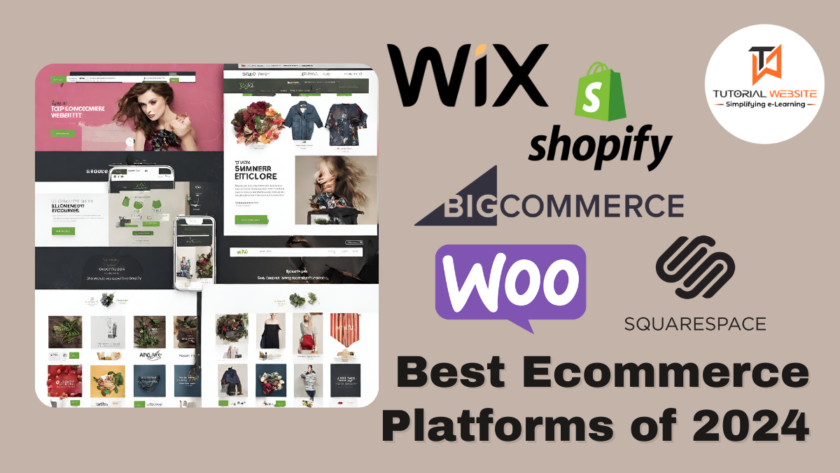
6 Best Ecommerce Platforms of 2024
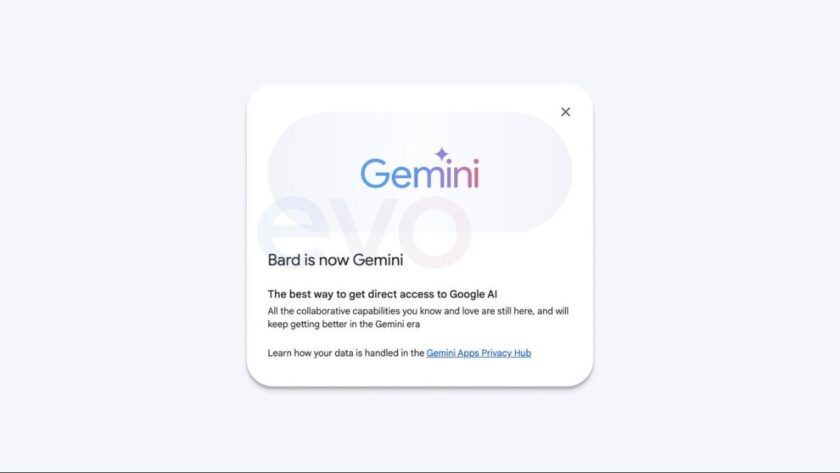
Google is preparing to rebrand Bard as Gemini
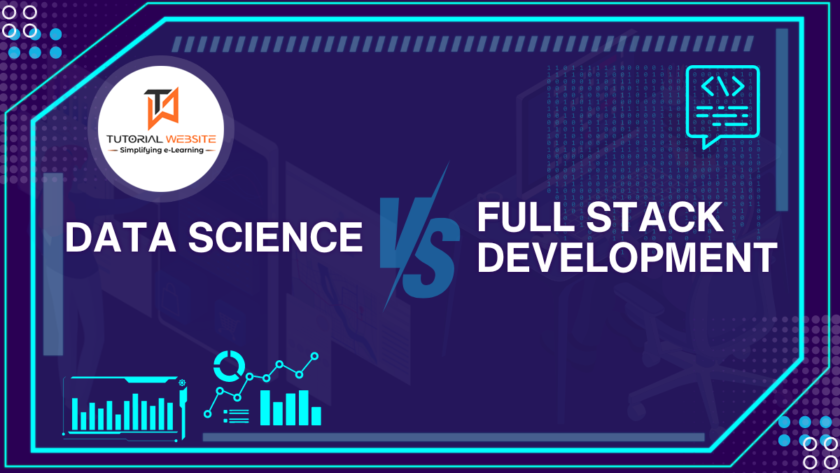
Data Scientist vs Full Stack Developer: Which You Should Choose
Privacy overview.
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- BCA 1st Semester Syllabus (2023)
Fundamentals of IT & Computers
- Basics of Computer and its Operations
- Characteristics of Computer System
- Types of Computers
- Number System and Base Conversions
- What is Algorithm | Introduction to Algorithms
- What is a Flowchart and its Types?
- What is an Operating System?
- DOS Full Form
- Types of Operating Systems
- Commonly Used Operating System
- Difference between Word Processor and Text Editor
- Introduction to Microsoft Word
- Introduction to MS Excel
- Introduction to Microsoft PowerPoint
C Programming
- C Programming Language Tutorial
- Operators in C
Control Structures in Programming Languages
- C if else if ladder
- Nested switch case
- Introduction to Divide and Conquer Algorithm - Data Structure and Algorithm Tutorials
- Understanding Time Complexity with Simple Examples
- What is PseudoCode: A Complete Tutorial
- Arithmetic Operators in C
- C Functions
- Parameter Passing Techniques in C
- Difference Between Call by Value and Call by Reference in C
- Scope rules in C
Basic Mathematics
- Determinant of a Matrix
- Mathematics | Limits, Continuity and Differentiability
- Advanced Differentiation
- Chain Rule Derivative - Theorem, Proof, Examples
- Taylor Series
- Relative Minima and Maxima
- Beta Function
- Gamma Function
- Reduction Formula
- Vector Algebra
Business Communication
- What is Communication?
- Communication and its Types
- BCA 2nd Semester Syllabus (2023)
- BCA 3rd Semester Syllabus (2023)
- BCA 4th Semester Syllabus (2023)
- BCA 5th Semester Syllabus (2023)
- BCA 6th Semester Subjects and Syllabus (2023)
- BCA Full Form
- Bachelor of Computer Applications: Curriculum and Career Opportunity
Control Structures are just a way to specify flow of control in programs. Any algorithm or program can be more clear and understood if they use self-contained modules called as logic or control structures. It basically analyzes and chooses in which direction a program flows based on certain parameters or conditions. There are three basic types of logic, or flow of control, known as:
- Sequence logic, or sequential flow
- Selection logic, or conditional flow
- Iteration logic, or repetitive flow
Let us see them in detail:
Sequential logic as the name suggests follows a serial or sequential flow in which the flow depends on the series of instructions given to the computer. Unless new instructions are given, the modules are executed in the obvious sequence. The sequences may be given, by means of numbered steps explicitly. Also, implicitly follows the order in which modules are written. Most of the processing, even some complex problems, will generally follow this elementary flow pattern.
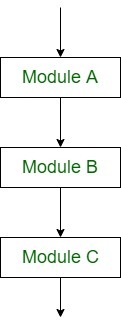
Sequential Control flow
Selection Logic simply involves a number of conditions or parameters which decides one out of several written modules. The structures which use these type of logic are known as Conditional Structures . These structures can be of three types:
Implementation:
- C/C++ if statement with Examples
- Java if statement with Examples
- C/C++ if-else statement with Examples
- Java if-else statement with Examples
- C/C++ if-else if statement with Examples
- Java if-else if statement with Examples
In this way, the flow of the program depends on the set of conditions that are written. This can be more understood by the following flow charts:
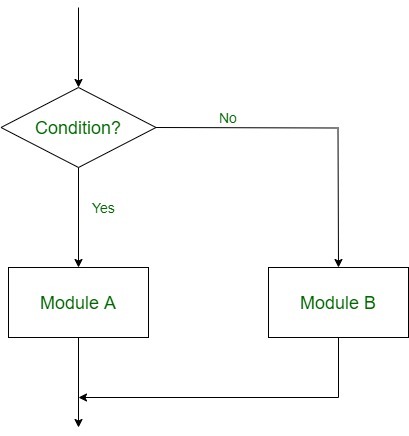
Double Alternative Control Flow
Here, A is the initial value, N is the end value and I is the increment. The loop ends when A>B. K increases or decreases according to the positive and negative value of I respectively.
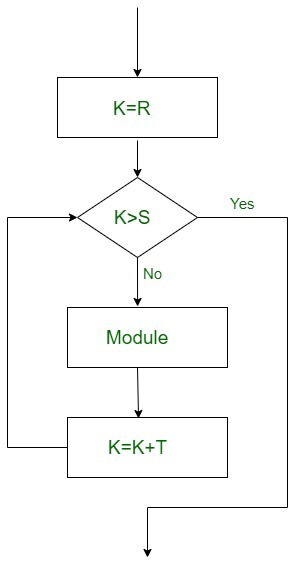
Repeat-For Flow
- C/C++ for loop with Examples
- Java for loop with Examples
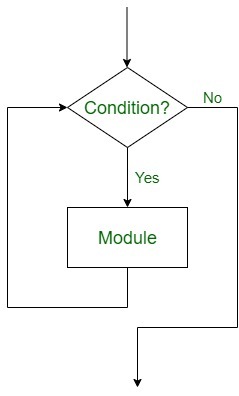
Repeat While Flow
- C/C++ while loop with Examples
- Java while loop with Examples
Please Login to comment...
Similar reads.
- Programming Language

Improve your Coding Skills with Practice
What kind of Experience do you want to share?
C Language Tutorial
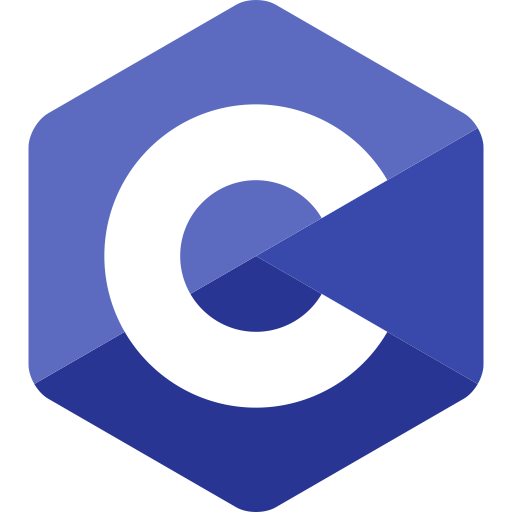
C Operators
C control structure, branch & jump stmt, control structures in c.
Control structures are an essential part of programming languages that allow you to control the flow of execution in a program. They determine the order in which statements are executed and enable you to make decisions and repeat blocks of code based on specific conditions.
Conditional Statements
- if statement: Executes a block of code if a specified condition is true.
- if-else statement: Executes a block of code if a condition is true; otherwise, executes a different block of code.
- else-if statement: Executes a block of code in which if condition become false then else if block will execute to check for next condition.
- nested if-else statement: Combines multiple if-else statements inside one another.
Nested if Statements in C
In C programming, nested "if" statements are used to create a hierarchy of conditional statements. They allow you to have an "if" statement within another "if" statement, creating multiple levels of decision-making.
When you have complex conditions or situations where multiple conditions need to be evaluated, you can use nested "if" statements. Each "if" statement is enclosed within another "if" or "else" statement, creating a nested structure.
The nested "if" statements are executed based on the result of the outer "if" statements. If the condition of the outer "if" statement is true, the program evaluates the condition of the inner "if" statement. This process continues for each nested "if" statement.
The syntax of nested "if" statements is as follows:
Let's consider an example where we check if a number is positive and even:
In this example, the program asks the user to enter a number. The program then uses nested "if" statements to determine if the number is positive and even.
If the number is greater than 0, the program evaluates the condition inside the nested "if" statement. If the number is divisible by 2 (i.e., it's even), it prints "Number is positive and even". Otherwise, it prints "Number is positive but not even".
If the number is not greater than 0, it directly prints "Number is not positive". The nested "if" statements allow the program to perform different checks based on the conditions in a hierarchical manner.
By using nested "if" statements, you can create complex decision-making structures in your C programs, allowing for more precise control and handling of different scenarios.
- Learn C Language
- Learn C++ Language
- Learn python Lang

TheCloudStrap
Learn Everyday!
Chapter 4: Control Structures in C Programming
Hello, coding enthusiasts!
As your guide in this adventurous journey of learning C, today we will dive into the thrilling realm of control structures. Don’t let the title intimidate you; it’s less ‘Lord of the Rings,’ more ‘Sherlock Holmes’. These are the fundamental building blocks that will help you manipulate the flow of your program, thereby solving any mystery your code might be hiding. With the right understanding of control structures – Conditional, Looping, and Jump Statements in C programming – you’ll be ready to navigate any labyrinth your code presents.
Conditional Statements in C Programming
Like a well-crafted choose-your-own-adventure book, a computer program also needs to make choices. And that’s where conditional statements come in handy.
Conditional statements in C (if, if-else, switch) are like the decision-making gears in your code. They help your program take one route over another, based on certain conditions. They’re like the ‘Y’ shaped fork on a hiking trail, where the path you take depends on what you wish to explore.
Let’s take a simple example:
In this code snippet, the ‘if’ statement evaluates whether your score is equal to or greater than 50. If it’s true, you passed. If not, you have another chance to try.
Looping Statements in C Programming
As you may have learned from life, some actions need to be repeated until a specific goal is achieved. The same is true in the world of programming, where we use loops to perform repeated actions. In C, there are three types of loop constructs: for, while, and do-while. They’re like the steady rhythm in your favorite song, repeating a beat until the melody reaches its desired end.
Take a look at this ‘for’ loop example:
This piece of code will print the phrase “Loop iteration: ” followed by the number of the current iteration, from 0 to 9, providing us with ten iterations in total. Notice how each loop construct brings its unique nuance to the rhythm, giving you the flexibility to choreograph your code’s flow as per the need.
Jump Statements in C Programming
Sometimes, in the grand narrative of our code, we need to abruptly jump from one point to another, bypassing the intervening steps. That’s where jump statements (break, continue, goto, return) make their dramatic entrance. Think of them as the plot twists in your favourite thriller novel, capable of drastically changing the storyline in an instant.
Let’s take a look at a ‘break’ statement:
In this case, when ‘i’ equals 5, the ‘break’ statement abruptly ends the loop, and the program control jumps out of the loop structure. The result? Our loop iterations will print only until 4, as the iteration for 5 will trigger the break and exit the loop.
Example Code – 1
Let’s develop an interesting C program which simulates a part of an aerospace software system. We’ll use this to demonstrate conditional statements, looping statements, and jump statements.
Imagine we have a spacecraft which has several systems that need to be checked before takeoff. We’ll use loops to iterate through each system, conditional statements to determine whether the system is ready or not, and jump statements to abort the launch if necessary.
Here’s a simplistic version of what such a code might look like:
In this code, the function check_systems iterates over each system (looping statement), checks if the system is ready or not (conditional statement), and aborts the launch if a system is not ready (jump statement). This simple program provides a nice, albeit simplified, representation of how these three key control structures might be used in a more complex aerospace software system. Remember, in real-world scenarios, system checks would be much more complex than a simple random assignment.
Example Code – 2
Let’s consider a more comprehensive example. Suppose we are working on a space shuttle control system. We will check various systems, such as engine systems, navigational systems, life support systems, and payload systems, and run a countdown before launching.
Here’s a simplified simulation of what such a code might look like:
In this code, the main function initiates a sequence of system checks. Each system is checked up to three times (looping statement), and if any check fails after three attempts, the mission is aborted (jump statement). If all checks pass, a final countdown begins, followed by lift-off. Note that this is a basic example and doesn’t cover the actual complexities and redundancies found in real-life aerospace software systems.
Understanding these control structures is like being handed the keys to a powerful, yet intricate machine. By knowing when and how to use conditional statements, loops, and jump statements, you can guide your program through any maze of complexity with ease and confidence.
Next time we’ll take these newly acquired skills and put them to the test. We will be exploring real-world applications of these control structures and writing code that feels like a symphony, with highs, lows, repeats, and occasional surprises.
Until then, keep coding, keep exploring, and remember, every great coder started right where you are now.
Happy coding!
This post was published by Admin.
Email: [email protected]
Related posts:
- Chapter 4: ADA Data Structures
- Chapter 2: Setting Up Your Development Environment for C Programming
- Chapter 3: The Fundamentals of C Programming
- Chapter 5: Object-Oriented Programming in ADA Programming Language
- Chapter 1: Introduction to C Programming
- Chapter 0: ADA Programming Language: Power, Safety, and Scalability
- Chapter 8: Advanced ADA Programming Language
- Chapter 9: Software Engineering with ADA Programming Language
- Chapter 10: Case Studies and Project Examples in ADA Programming Language
- Chapter 11: Troubleshooting and Best Practices in ADA Programming language
Privacy Overview
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar
Circuit Crush
Learn Electronics & Microcontrollers
C Programming Tutorial 4: Control Structures in C

Welcome to the 4 th installment of the C Programming Tutorials!
This time around, we’re going to talk about some common C control structures and conditional statements.
These let you do some amazing things with a little amount of code.
Normally, statements in a program execute one after the other in the order in which they appear. The word for this is sequential execution. The statements we will cover here enable the programmer to specify that the next line to execute may be other than the next one in sequence.
In other words, instead of executing statements in order, control structures will allow us to jump to other places in the program. This is a very powerful concept as we’ll soon see.
We’ll start with a quick intro to pseudocode and then jump into the if statement.
Pseudocode is an artificial, informal language that helps programmers develop algorithms.
It’s kind of like real code, but also close to every day English. Pseudocode is convenient and user friendly. It leaves out some of the details the processor or microcontroller would need, so it’s not an actual computer programming language. However, pseudocode is more human-readable and is a good starting point when writing complex programs in any language.
And a carefully written pseudocode “program” can easily be converted into C.
Below is an example of pseudocode that tells you if you’ve passed a test.
One advantage of pseudocode is that it enables you to concentrate on the logic and organization of a program and spares you from simultaneously worrying about how to express the ideas in a computer language. And it’s not tied to any particular language.
I know this seems sort of random, but since control structures allow you to write more complex C programs, now is a good time to introduce the concept of pseudocode.
Now let’s delve into some common C programming control structures.
C Programming Control Structures
C programming: the if statement.
The if statement checks for a condition and executes the proceeding statement or set of statements if the condition is true. If false, it skips the statement.
The if statement is called a branching statement or selection statement because it provides a junction where the program selects which of two paths to follow.
The syntax for an if statement is simple and goes something like this:
For example, let’s say you want to turn on a fan when the attic hits a certain temperature.
To read the temperature, a thermistor (heat sensitive resistor) connects to a microcontroller’s ADC.
Let’s assume a 10-bit ADC. The values the ADC will return will range from 0 to 1023. So, if it’s cold, the value with be on the small side and if it’s hot the value will be larger.
Become the Maker you were born to be. Try Arduino Academy for FREE!

Let’s look at an if statement that could accomplish this in pseudocode.
This pseudocode snippet reads the ADC with readADC (which is a function, we’ll talk more about functions in another tutorial) and then assigns that value to an integer named Temp.
As we can see below, translating the pseudocode into more realistic code isn’t difficult.
Note that the code inside the curly braces only executes if the conditional statement is true. If readADC() returns a value less than 500, the code does not execute.
An if…else statement is a power-up for your if statements. It allows you greater control over the flow of code than the basic if statement. This will allow you to test for more than one condition.
For example:
Here we turn on the fan if it’s too hot as before, but now if it gets too cold we sound an alarm. Note that we can use as many else…if statements as we like to test for as many conditions as we want.
C Programming: the for Loop
The for loop is one of the most useful and common pieces of code out there. It’s often used with arrays, a subject that you’ll want to become familiar with which we’ll cover in a future tutorial. The syntax is shown below.
The parentheses following the keyword for contain three expressions separated by two semicolons.
The first expression is the initialization. It happens just once, when the loop first starts.
The second expression is the test condition; it is evaluated before each potential execution of a loop. When the expression is false, the loop terminates.
The third expression, the incrementation, is evaluated at the end of each loop.
At first, the for loop can be confusing and intimidating, but after a while you’ll become good friends.
An example from the Arduino website is given here. The for loop in Arduino works the same way as in C and the syntax is the same. Remember that in Arduino main() is hidden and setup() and loop() are required.
Take a look at the for loop. First, we set or initialize the variable i to 0 (this part only happens once the first time we go through the loop; after the first time this statement is ignored and the other two are executed).
Then comes the condition. As long as i is less than or equal to 255 we run through the loop. Integer i starts at 0, which is less than 255, so we step into the loop and write the value of i to PWMpin.
Then we increment i (the i++ statement increments it by one each time it passes through the loop). Once i becomes greater than 255, we fall out of the loop and the code inside the loop ceases to execute.
The for loop has some amazing flexibility built into it.
For example, if we want to, we can decrement i (i–) instead of incrementing it (i++).
We can also count by two’s, three’s, ten’s or what ever number we want. The code fragment below starts at 2 and counts by 11 as long as n is less than 124.
Tired of numbers? Then count by characters:
This code fragment prints the ASCII value of each letter a though z. The code works because characters are stored as integers, so this loop is actually counting by integers.
You can leave one or more expressions within the for loop blank (don’t omit the semicolons). Just be sure to include within the loop itself some statement that eventually causes the loop to terminate.
And there is a myriad of other things one can do with a for loop.
C Programming: The while Loop
The while loop is another shiny diamond in the rough that you’ll often find yourself using.
It will loop continuously (and forever) until the expression inside the parenthesis becomes false. The syntax is shown here.
If condition is true (or nonzero, any nonzero value evaluates to true), the statement executes once and then the expression is tested again. This cycle of test and execution repeats until condition becomes false (or zero). Each cycle is an iteration of the loop.
Note that the condition is a Boolean expression and it either true or false. The condition can be many things like some sort of less than or equal comparison, the number 1 (which means true), or even a function.
This suggests an important point about while loops.
When you write a while loop, it must include something that changes the value of the test condition so that it eventually becomes false. Otherwise, the loop never terminates.
Consider the code snippet below.
This snippet will loop forever. Believe it or not, there are times when this is appropriate.
A more practical example is shown below.
In this case, the loop will run as long as readADC() returns some sort of value that’s not 0.
Here’s another partial example demonstrating the while loop:
The ++ or incrementation operator has reared its head again and increments clock every time we go through the while loop to keep count.
A while Loop Gotcha
We touched on this last time, but it’s worth repeating because at some point I guarantee you will make a mistake like this. I know I did.
Remember from C Programming Tutorial 3 that the assignment operator (=) is not the same thing as the equal sign (==).
When doing a comparison with a while loop, you’ll likely be using == rather than the assignment operator.
Consider the code fragment below.
The value of an assignment statement is the value of the left side, so status = 1 has the
same value as 1. So, this while loop is the same as using while (1). It never quits and loops infinitely.
Now consider the correct way.
Try not to confuse = for ==. Some computer languages, like BASIC do use the same symbol for both the assignment operator and the equality operator, but the two operations are quite different.
The assignment operator assigns a value to the left variable. The equality operator simply checks to see if the left and right sides are already equal. It doesn’t change the value of the variable on the left.
Of course, the compiler may let you use the wrong form, bringing results that are odd and not what you expect.
C Program Control Final Points
At this point, you may be asking how do I know which loop or control structure to use and when?
A for loop is appropriate when the loop involves initializing and updating a variable. It’s a better choice for loops involving counting with an index, like the loop below.
A while loop is better when the conditions are otherwise, like when we do not know what the initial value will be or how long it will be true. Consider the same code from earlier.
We may not know how long status will be 1 (or true) so using an index wouldn’t make sense here. Situations like this is where the while loop really shines.
Of course, we can also use the while loop when we do know how many iterations we’ll have, like the code snippet below.
Before we wrap up, here are some key concepts to remember when using loops or any sort:
- Clearly define the condition that causes the loop to terminate (unless you want it to run forever)
- Be sure to initialize the values in the loop test before the first use
- Make sure the loop does something to update the test each iteration
Also, below is a handy chart that can help you decide which of the three control structures we discussed in this post to use. And yes, there are some control structures we didn’t discuss but we’ll pick those up next time.
Figure 1: C control structures from this post.
“C” you in the next tutorial!
Until then comment and tell us what you favorite C programming control structure or loop is. Why do you like it?

Reader Interactions
October 13, 2018 at 4:31 pm
I’ve always been a fan of the for loop. As you pointed out, it’s very flexible.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Browse Course Material
Course info, instructors.
- Kyle Murray
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Introduction to c and c++, core c: control structures, variables, scope, and uninitialized memory, lecture notes.
Lecture 2: Core C (PDF)
Lab Exercises
The primary goal of this lab period is to make sure you know all of the basics of writing code in C and to avoid common pitfalls unique to C and C++.
Starting with an empty .c file, write a C program to calculate the first 100 triangular numbers (0, 1, 3, 6, 10, 15, 21, …). You may check back at previous example code and slides if you get stuck, but take this an opportunity to try to recall, from memory, the standard boilerplate code you’ll be writing often when starting a new C program, like the proper #include statements to be able to print to the console.
Find and fix the variable shadowing bug in the following C program, which is intended to calculate the factorial function:
Assignment 2
Rewrite the program below, replacing the for loop with a combination of goto and if statements. The output of the program should stay the same. That is, the program should print out the arguments passed to it on the command line.
If you ran the program like this: ./prog one two three , it would print
In lecture, we covered a variety of control structures for looping, such as for , while , do/while , goto , and several for testing conditions, such as if and switch .
Your task is to find seven different ways to print the odd numbers between 0 and 10 on a single line. You should give them names like amaze1 , amaze2 , etc. Here’s a bonus amaze (that you can use as one of your seven if you like):
You may need to get a little creative!
Put all of your functions in the same C file and call them in order from the main() function. We should be able to compile your program with this command and see no errors:
gcc -Wall -std=c99 -o amaze amaze.c
Solutions are not available for this assignment.

You are leaving MIT OpenCourseWare
Control Structures in C: Getting Started with Control Structures
- 17 videos | 1h 54m 18s
- Includes Assessment
- Earns a Badge
WHAT YOU WILL LEARN
In this course.
- Playable 1. Course Overview 2m 6s FREE ACCESS
- Playable 2. Understanding C Control Structures 6m 40s In this video, you will learn how to outline three types of control structures used in the C language. FREE ACCESS
- Locked 3. Using if Statements in C 7m 55s In this video, you will learn about the use of basic if statements. FREE ACCESS
- Locked 4. Using Relational Operators with if Statements in C 6m 55s In this video, learn how to use relational operators with if statements. FREE ACCESS
- Locked 5. Chaining Relational Operators with Logical Operators 4m 59s In this video, you will learn how to use logical operators to connect relational operators. FREE ACCESS
- Locked 6. Exploring Quirks of if Statements in C 8m 46s In this video, find out how to identify common mistakes with if statements. FREE ACCESS
- Locked 7. Using if-else Conditional Blocks in C 9m 17s Learn about the use of if-else blocks. FREE ACCESS
- Locked 8. Creating Nested if-else Blocks in C 6m 23s Learn about the use of if-else blocks within other if-else blocks. FREE ACCESS
- Locked 9. Exploring Nested if Statements in C 7m 12s In this video, you will learn how to run code with if-else blocks nested inside each other. FREE ACCESS
- Locked 10. Introducing the else-if Block in C 4m 51s During this video, you will learn how to outline the if-else-if control structure. FREE ACCESS
- Locked 11. Using the if-else-if Ladder in C 8m 39s In this video, you will learn how to run code with the if-else-if ladder. FREE ACCESS
- Locked 12. Understanding the switch Statement in C 7m 17s Upon completion of this video, you will be able to illustrate the mechanics of the switch statement. FREE ACCESS
- Locked 13. Running Code with the switch Statement in C 8m 30s In this video, you will learn how to run code with switch statements. FREE ACCESS
- Locked 14. Using switch Statements for Various Situations in C 9m 16s Learn how to use switch statements in various scenarios. FREE ACCESS
- Locked 15. Exploring Nuances of switch Statements in C 5m 28s In this video, you will learn about the use of characters in switch statements. FREE ACCESS
- Locked 16. Shortening if-else Blocks Using Ternary Operator 7m 2s Learn how to use the ternary operator to shorten if-else blocks. FREE ACCESS
- Locked 17. Course Summary 3m 2s 097f01e9-2d12-429e-974a-3ec42c4d9e53 FREE ACCESS

EARN A DIGITAL BADGE WHEN YOU COMPLETE THIS COURSE
Skillsoft is providing you the opportunity to earn a digital badge upon successful completion on some of our courses, which can be shared on any social network or business platform.
YOU MIGHT ALSO LIKE
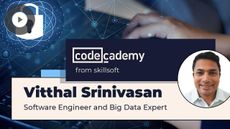
PEOPLE WHO VIEWED THIS ALSO VIEWED THESE
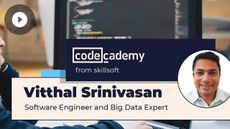
Next: Execution Control Expressions , Previous: Arithmetic , Up: Top [ Contents ][ Index ]
7 Assignment Expressions
As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues ) because they are locations that hold a value.
An assignment in C is an expression because it has a value; we call it an assignment expression . A simple assignment looks like
We say it assigns the value of the expression value-to-store to the location lvalue , or that it stores value-to-store there. You can think of the “l” in “lvalue” as standing for “left,” since that’s what you put on the left side of the assignment operator.
However, that’s not the only way to use an lvalue, and not all lvalues can be assigned to. To use the lvalue in the left side of an assignment, it has to be modifiable . In C, that means it was not declared with the type qualifier const (see const ).
The value of the assignment expression is that of lvalue after the new value is stored in it. This means you can use an assignment inside other expressions. Assignment operators are right-associative so that
is equivalent to
This is the only useful way for them to associate; the other way,
would be invalid since an assignment expression such as x = y is not valid as an lvalue.
Warning: Write parentheses around an assignment if you nest it inside another expression, unless that is a conditional expression, or comma-separated series, or another assignment.
![assignment in control structure c [Diagram:Pic/COMP9024-small.png]](https://www.cse.unsw.edu.au/Pic/COMP9024-small.png)

- PG Diploma in Embedded Systems Design & Development
- PG Diploma in Internet of Things (IoT)
- Full Stack Java With Placements
- Professional Diploma in Software Programming
- PG Diploma in Data Science – Coming Soon
- Diploma Programs- Working Professionals
- Programming in C
- MATLAB & Simulink
- Internet of Things – IoT
- Artificial Intelligence
- Machine Learning
- Embedded System
- Automotive Embedded System
- Basic Electronics
- College Workshop
- Internship Program
- Faculty Development Program (FDP)
- Placement Prepardness Program (PPP)
- Summer Training Programs (STP)
- Academic Projects
- R&D Lab Setup
- Corporate Training

Recruiters Area
- Entrance Test
- Student Login

Control Structures 101: A Fundamentals Guide for C Programming

Introduction
Control structures are programming constructs that enable developers to have control over the flow of a program. They determine the execution order of statements based on certain conditions. By using control structures, programmers can make decisions, repeat actions, and alter the flow of their code. Control structures are essential in programming as they allow for dynamic and efficient program execution. Control structures play a crucial role in directing the flow of a program. They facilitate branching and looping, allowing developers to execute specific code blocks based on conditions or to repeat a certain block of code multiple times. Control structures help in implementing decision-making, iteration, and execution control, which are fundamental to writing efficient and effective programs.
In C programming , there are several common control structures that are used extensively. These include conditional statements like the “if” statement and the “switch” statement, as well as looping structures like the “while” loop, the “for” loop, and the “do-while” loop. These control structures provide flexibility and allow developers to write code that can adapt to various scenarios and conditions.
Conditional Statements: Making Decisions in C
The “if” statement.
The “if” statement is one of the most basic and commonly used conditional statements in C programming. It enables the execution of a certain block of code based on a specified condition. The syntax of the “if” statement is straightforward, and it can be used to handle single as well as multiple conditions. Additionally, “if” statements can be nested, allowing for complex decision-making in programs.
The “else” Statement
The “else” statement is used in conjunction with the “if” statement to specify an alternative block of code to be executed when the condition in the “if” statement evaluates to false. By combining the “if” and “else” statements, developers can create decision-making structures that offer multiple paths of execution based on different conditions.
The “switch” Statement
The “switch” statement allows for multiple branches of execution based on the value of a variable. It provides an alternative to multiple consecutive “if” statements and offers a concise way to handle different cases. The “switch” statement compares the value of the variable against various “case” labels and executes the corresponding block of code. It also provides a way to handle default cases when none of the specified cases match.
Looping Structures: Repetition Made Easy
the “while” loop.
The “while” loop is used when a certain block of code needs to be repeated as long as a specified condition is true. It is ideal for situations where the number of iterations is not known beforehand. The syntax of the “while” loop is straightforward, and it allows developers to control the entry and exit conditions of the loop based on the value of the condition.
The “for” Loop
The “for” loop is a versatile looping structure that allows developers to define the initialization, condition, and iteration all within a single line. It is often used when the number of iterations is known or when iterating over a collection of elements. The “for” loop provides more control over the loop structure and also allows for loop optimization and best practices.
The “do-while” Loop
The “do-while” loop is similar to the “while” loop, but it guarantees that the loop code block is executed at least once before checking the condition. This can be useful in situations where the loop code block needs to be executed regardless of the initial condition. The “do-while” loop structure ensures that the loop body is executed first before evaluating the condition.
Breaking and Continuing Loop Execution
In C programming, the “break” and “continue” statements are used to alter the execution flow within loop structures . The “break” statement is used to exit the current loop and move on to the next section of code outside the loop. This can be useful to terminate a loop prematurely based on a certain condition. On the other hand, the “continue” statement allows programmers to skip the remaining code within a loop and move to the next iteration.
Jump Statements: Altering Control Flow
“goto” statement.
The “goto” statement is a powerful but controversial feature in C programming. It allows programmers to transfer control to a labeled statement within the same function. While the “goto” statement can simplify code flow in certain situations, it can also lead to difficult-to-maintain code and increase the risk of introducing bugs. It is generally recommended to use other control structures like loops and conditional statements instead of the “goto” statement.
“return” Statement
The “return” statement is used to exit a function and return a value to the caller. It plays a vital role in controlling the flow of a program and terminating functions when necessary. Returning values from functions allows developers to pass data back to the caller or to indicate the success or failure of a function. Understanding how to use the “return” statement correctly is essential for proper program execution.
“break” and “continue” Revisited
In addition to their usage in loops, the “break” and “continue” statements can also be used in nested loops. When used within nested loops, the “break” statement terminates the innermost loop and resumes execution from the first statement outside the loop. The “continue” statement, on the other hand, skips the remaining code for the current iteration of the innermost loop and moves to the next iteration.
Practical Examples: Applying Control Structures
Example 1: simple calculator.
In this example, we will design a program to create a simple calculator using control structures in C. We will utilize conditional statements to handle menu selection and perform various mathematical operations based on user input. By using control structures effectively, we can create a user-friendly calculator program with clear menu options and accurate calculations.
Example 2: Looping for Pattern Printing
In this example, we will explore how control structures can be used to create different patterns using nested loops. We will design a program that takes user input to determine the number of rows and columns for a pattern and then use nested looping structures to print the desired pattern. By utilizing “for” and “while” loops together, we can dynamically generate patterns of various shapes and sizes.
Example 3: Checking Prime Numbers
In this example, we will create a program that checks whether a given number is prime or not using control structures in C . We will utilize loop structures and conditional statements to implement an algorithm for verifying prime numbers. By carefully selecting the appropriate control structures and utilizing conditional statements, we can efficiently determine whether a number is prime or composite.
Best Practices for Using Control Structures
Readability and code formatting.
When utilizing control structures, it is essential to ensure code readability. Proper indentation, clear naming conventions, and consistent code formatting help improve the readability of the codebase. By following best practices for code formatting, developers can make their control structures more understandable and maintainable.
Avoiding Nested Loops and Complex Control Structures
While nested loops can be useful, excessive nesting can make the code difficult to understand and debug. It is generally recommended to avoid overly complex control structures and nested loops whenever possible. Simplifying control flow by breaking down complex structures into individual functions or utilizing helper variables can make the code more manageable and maintainable.
Comments and Documentation
Adding comments and documentation to control structures is crucial for future reference and collaboration. It helps other developers understand the intent and purpose of the control structures. By providing clear and concise comments, developers can improve code comprehension and enhance the overall maintainability of the project.
Testing and Debugging Control Flow
Thoroughly testing and debugging control structures is essential to ensure their proper functioning. By systematically designing test cases that cover all possible scenarios, developers can verify the correctness of their control structures. Additionally, using proper debugging techniques and tools can help in discovering and fixing any issues related to control flow.
Advanced Control Structures (Brief Overview)
“if-else if-else” ladder.
The “if-else if-else” ladder is an extension of the “if-else” statement that allows developers to check multiple conditions in a sequence. Each condition is evaluated in order, and the corresponding block of code is executed if the condition is true. This control structure is ideal when there are multiple mutually exclusive conditions to be checked.
Nested “switch” Statements
The “switch” statement can also be nested within another “switch” statement, allowing for more complex decision-making. By nesting “switch” statements, developers can handle multiple levels of cases and further refine the execution flow based on various conditions.
Multi-level Looping
Multi-level looping refers to the usage of nested loops with different termination conditions. This allows developers to iterate over multiple dimensions or nested data structures. By using multi-level looping, complex patterns or operations involving multiple dimensions can be easily implemented.
In this blog post, we discussed the fundamentals of control structures in C programming . We explored conditional statements like the “if” statement and the “switch” statement, as well as looping structures like the “while” loop, the “for” loop, and the “do-while” loop. We also delved into jump statements like the “goto” statement and the “return” statement. Additionally, we provided practical examples of applying control structures in C programming .
Mastering control structures is essential for any C programmer. Control structures allow for dynamic program flow, decision-making, and repetition. By understanding and effectively utilizing control structures, developers can create efficient and flexible programs that fulfill specific requirements. Understanding control structures is a fundamental aspect of learning C programming. To enhance your C programming skills further, consider exploring topics like data types, functions, arrays, and pointers. Additionally, practicing coding exercises and participating in programming projects can help solidify your understanding of control structures and improve your overall programming proficiency.
Must Read: Understanding and Utilizing Pointers in C Programming
Indian Institute of Embedded Systems – IIES
Admission
Privacy Policy
Certification Courses
- PG Diploma in Embedded Systems Design & Development
- Certification Courses– Freshers
- Institutional Training– Undergraduate
Get In Touch
- Indian Institute of Embedded Systems (IIES) - No 80, Ahad Pinnacle, Ground Floor, 5th Main, 2nd Cross, 5th Block, Koramangala Industrial Area Bangalore 560095, Karnataka, India
- Phone +91 80 4121 6422
- Mobile +91 98869 20008
- [email protected]
- [email protected]
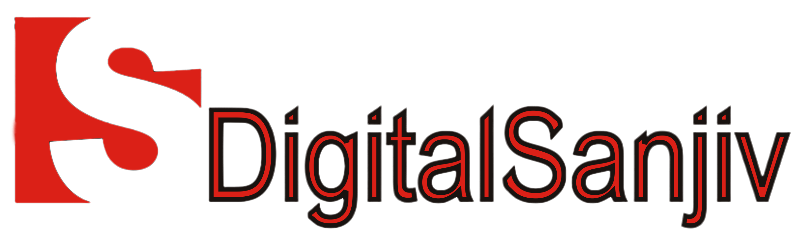
Online Digital Learning
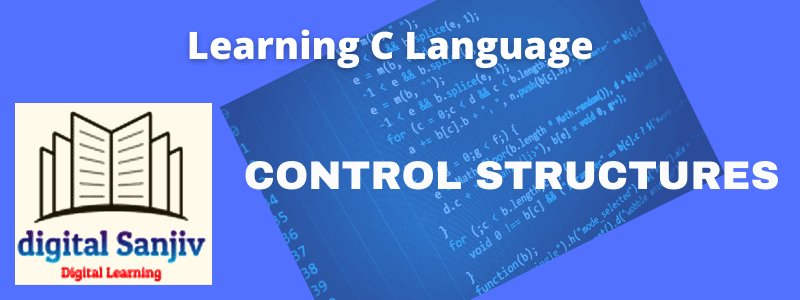
Control Structures in C
Table of Contents
The structures of a program which affects the order of execution of the statements are called control structures. The normal execution of statements is in sequential fashion but to change the sequential flow, various control structures are used. Broadly, control structures are of three types-
- Selection /conditional – executes as per choice of action.
- Iteration – Performs a repetitive action.
- Jumping – used to skip the action, or particular statements.
Selection/Conditional control structures
Also called conditional control constructs and are used to choose from alternative course of actions based on certain conditions. It has various forms
- nested if-else
- switch -case
If Statements
If statement tests a condition and if it results in non-zero(true) value then the statements following if are executed.
- There is no semi colon after if statement
- The conditional expression of c can have relational (<,<=, >,>=,==,)or logical(&&,||,!) operator but can’t have assignment operator.
Example to print the largest of three numbers
Explanation – In the first if statement, it will scan for three variables a,b and c. This if statement will be TRUE only if all three variables are entered through standard input device.
Then value of a is assigned to d.
if(b%d) d=b;
In the second if statement modulus value of b against d is calculated , it will result in non zero if b is greater than d(which is actually value of a). hence value of b would be assigned to d. It actually means that b is greater than a. Now
if(c%d) d=c;
value of c is compared with b and if it returns as non zero then c is greatest of all. Otherwise b is greatest.
In second and third if statements are false then a is the greatest of all.
if statement does not give any output if condition is false. To get the result for both true and false, if-else statement is used.
If-Else Statement
This control structure tests the if conditions and if it is true then the body of if is executed otherwise body of else is executed. If-else is more complete version as compare to simple if statement where you have statements for TRUE and FALSE conditions.
Note: Body of if -else statement should be enclosed in curly braces if it have more than one statements. The same is not mandatory for single statement.
Nested if-else statement
Sometimes, the body of if-else statement may be placed within another if-else statement then it is called nested if-else statement. This control structure is particularly helpful to simultaneously test more than one conditions. For example a group of friends are planning a picnic on Sunday if it is a sunny day. So here two conditions are simultaneously tested, the day should be sunday and second it should be a sunny day.
Example to find out the largest of three numbers.
If-else if Statement
When you have to evaluate more than two conditions then you need to use if-else if ladder. This control structure is particularly helpful when you have more conditions to evaluate.
Example to demonstrate grade of a student
Switch Statement
Switch control structure can cut short the long series of if-else if statements . The switch statement can select a case from several available cases. The case can be integral expression(int or char) only. If the case value matches with any of the available cases then statements below it are executed until break statement is encountered. If no matching case is found then default label is executed. If no default label has been defined then switch terminates.
What are the differences between if and switch statement?
- If statement can test any type of expression (integral, floating point, logical, relational etc.) but switch can test only integral expression only.
- If statement encloses multiple statements inside curly braces but you can never put multiple statements of switch inside curly braces.
- Break can be used inside switch body but can’t be used inside if body.
Iterations means repetitions. When you wish to repeat a task more than once then it is called iterative task. The iterative tasks can be performed in c language through loops. Looping means to execute a task repeatedly and simultaneously checking the condition when to stop.
Loops control structures can be used to –
- take same action on each character of a file until end of file is reached.
- take the same action until the number becomes zero.
- take the same action on each elements of an array until all array elements are processed.
- take the same action until newline character is reached
- take the same action on each item in the data set.
- take the same action until the file gets printed.
C Language has three types of loops
- do-while loop
For loop steps through a series of specified action once for each value monitoring the specified condition to terminate each time.
for(initial_exp;cond_exp;modifier_exp)
here initial_exp – initialization expression. It initializes a variable with initial value
cond_exp – conditional expression. This condition decides whether to continue the loop or exit it.
modifier_exp – modifier expression. It changes the value of the variable after each iteration.
Example to write a counting from 0 to the value of i
Infinite Loop
Infinite loop is a control structure which keep on executing it’s body indefinitely. Either it will keep on giving output continuously or no output at all. This kind of loops are also called indefinite or endless loop.
Note – A loop control structure should always be finite means it should terminate after some iterations, else it becomes infinite loop . Infinite loops are highly undesirable and due care should be taken to keep the loops finite.
for loop usage
1 for loop control structure is best suited for the situations where we know in advance how many times the loop will execute.
2 for loop control structure is natural choice for processing the array elements of all dimensions
Conditions for infinite for loop
- A for loop without conditional test is infinite for loop
- A for loop whose conditional test is true for loop variables initial value but it’s not modified then loop becomes infinite loop.
While loop is also called pre-test loop. It first tests the specified conditional expression and as long as the conditional expression evaluates to non-zero, action is taken.
while(conditional expression)
body of while
here conditional expression can be condition or expression. so
while(x=x+1)
while(n–) are all legal conditional expression in while loop.
Important points about while loop
- While loop is mostly suited in the situations where it is not known for how many times the loop will continue executing. e.g. while(number!=0)
- If conditional expression in a while loop is always evaluated as non zero then it infinite while loop. e.g. while(1) or while(!0).
- Never us assignment operator in place of equality operator in the test condition
- If the conditional expression evaluates to zero in the very starting then loop body will not execute even once.
Example – to reverse a positive number
Do-while loop
Also called post test loop because it executes it’s body at least once without testing specified conditional expression and then makes the test. Do-while terminates when the conditional expression evaluates to zero.
Notice the semicolon after condition
Important points about do-while loop
- A do-while loop tests the condition after executing the body at least once.
- A do-while will make minimum iteration as 1 even if the condition is false.
- A do-while loop is good choice for processing menus because of it’s first task then test nature.
Difference between while and do-while loops
3 Jumping
There are three jumping statements
Break Statement
Break is a jumping statement that when encountered in a –
- Switch block – skips the rest of the switch block at once and jump to the next statement just after the switch block
- Loop- skips the rest of the loop statements and jumps to the next statement just after loop body
Example – To find the given number as prime
Continue Statement
Continue is also a jump statement which skips rest of the statements in the loop body and jumps to the next iteration. It differs from break in a way that break simply comes out of a block in which it is defined but continue just skips the rest of statements after it and continue to execute the loop body with next iteration.
Goto statement
Although goto statement is avoided in c language still it is available in two forms unconditional goto and conditional goto. it is a jump statement which causes the control to jump from the place where goto is encountered to the place indicated by label.
- Unconditional goto – Used to make jump to the specified label without testing a condition.
- Conditional goto – Used to make jump to the specified label after testing condition.
Similar Posts : Input/Output functions in C Operators and Expressions in C Variables in C
You may also like :
Let us Learn JavaScript from Step By Step Reverse Charge Mechanism in Tally.ERP9 What is New in HTML5
To Download Official TurboC Compiler from here
Difference between break and continue
1) Break causes exit from the loop leaving rest of the iterations while continue causes control to continue with the next iteration 2) break can be used in all control structures (for, while, do-while loops and switch statements ) except constructs of if family. Continue can be used only in the looping constructs but not in switch and if family. 3) Break can be used inside an infinite loop to stop them. Continue can be used inside infinite loops to skip one iterations but it can not stop it.
Similarities between break and continue
1) Both are used to jump from one place to another by skipping rest of the statements after them. 2) Both can be used inside the loops. 3) Inside a loop both can be used with if or switch statements.
Infinite Loops
An infinite loop is a loop that keeps on executing its body as there is not control . Reason for loop to be infinite are – 1 When conditional expression always evaluates to TRUE 2 No condition is imposed to come out of the loop 3 No modifier expression to modify the loop variable
How to stop an infinite loop
By pressing Ctrl+break keys
[…] in C &n…Input/Output functions in […]
[…] Control Structures in C Arrays in […]

Please Subscribe to Stay Connected
You have successfully subscribed to the newsletter
There was an error while trying to send your request. Please try again.
Introduction to C Programming Language
- Introduction
- Setting up your environment
- The Linux programming environment
- Compiling & Debugging C
- Introduction to Git
- The C programming language
- Integer types in C
- Floating-point types
- Operator precedence in C
- Input and output
Statements and control structures
- Structured data types
- Type aliases using typedef
- Data structures and programming techniques
- Asymptotic notation
- Dynamic Arrays
- Linked lists
- Deques and doubly-linked lists
- Circular linked lists
- Abstract data types
- Hash tables
- Generic containers
- Object-oriented programming
- Binary Search Trees
- Augmented trees
- Balanced trees
- Red-black trees
- Dynamic programming
- Randomization
- String processing
- Unit testing C programs
- Bit Manipulation
- Persistence
- What's next?
- C Language Limitations and How It Compares to Other Languages
Related Books
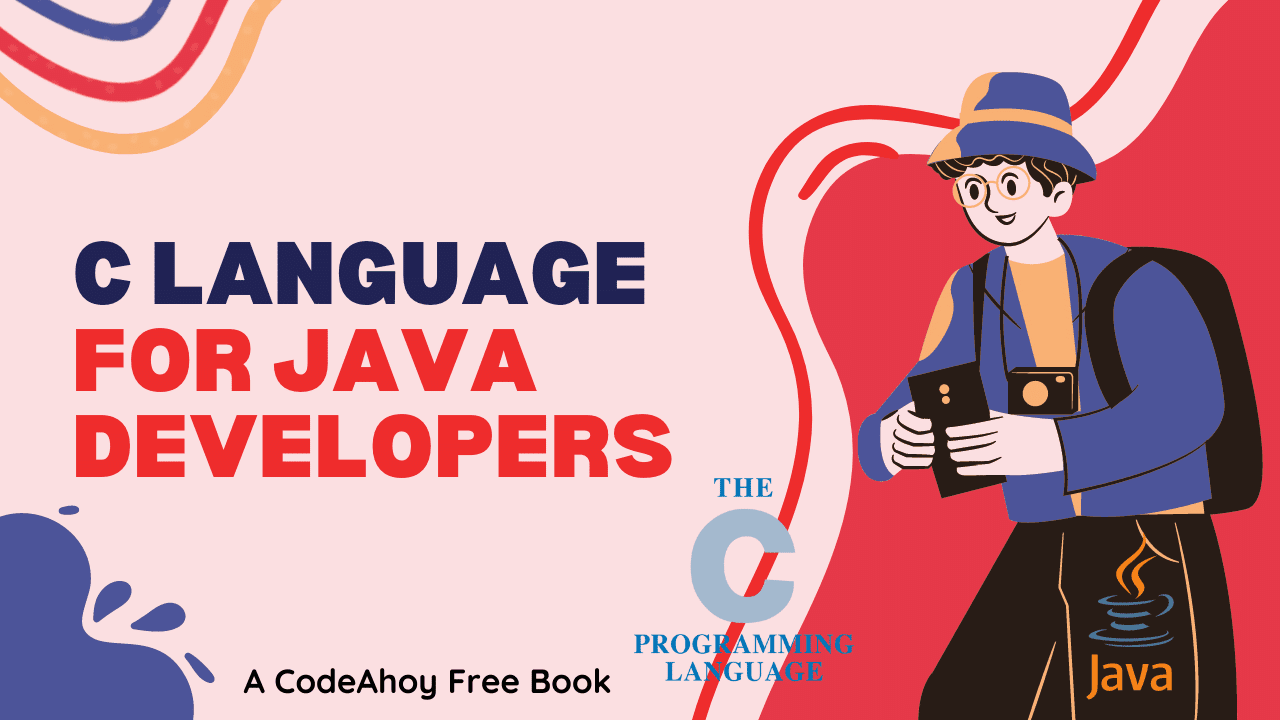
C Language Primer for Java Developers
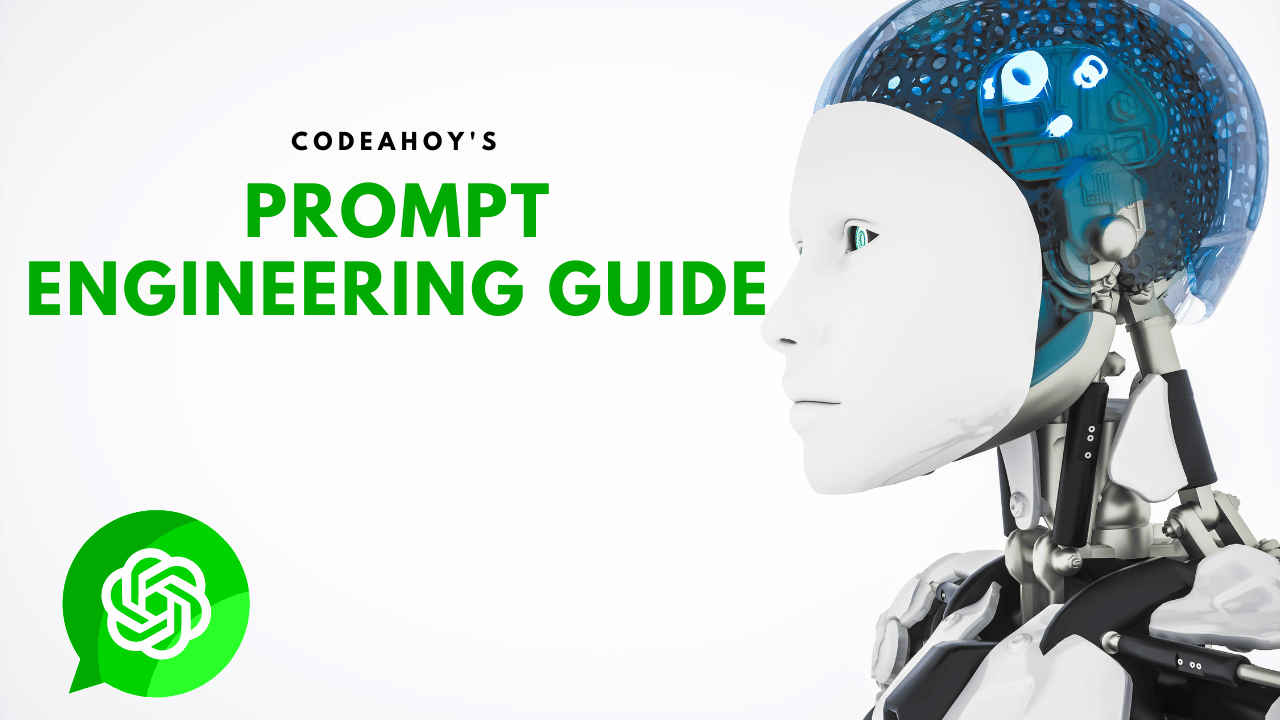
Guide to Prompt Engineering
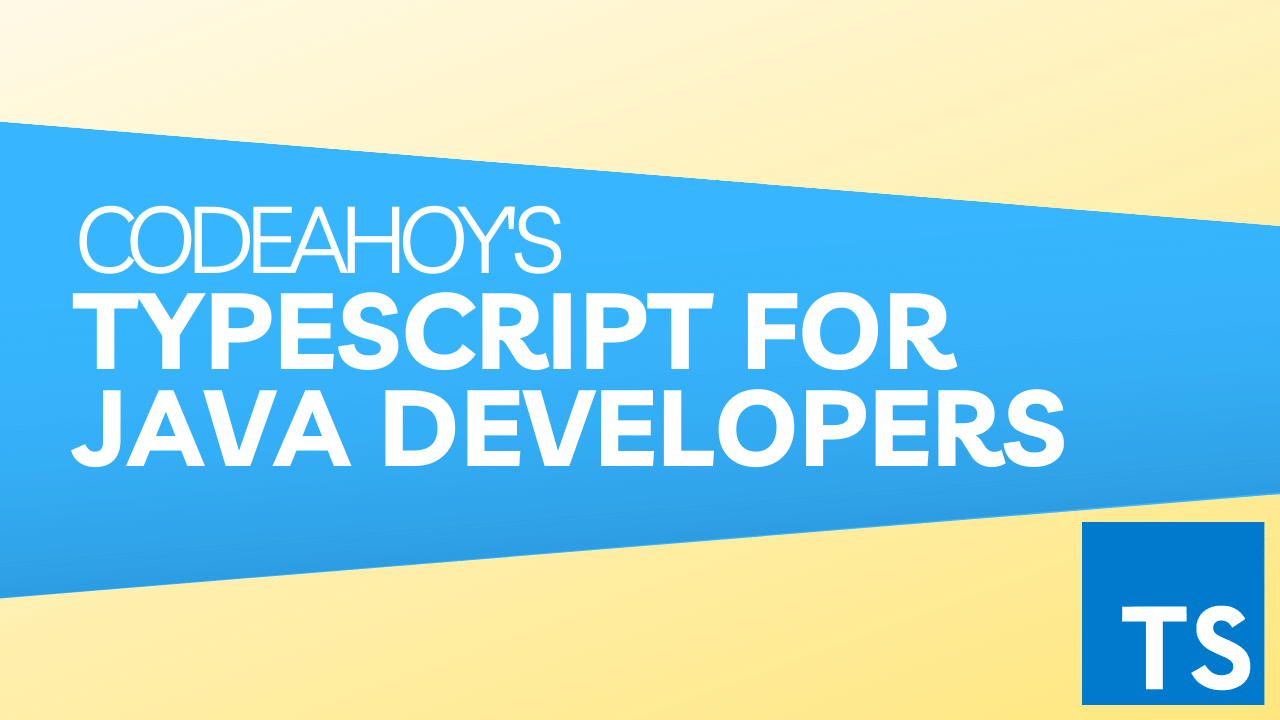
TypeScript for Java Developers by CodeAhoy
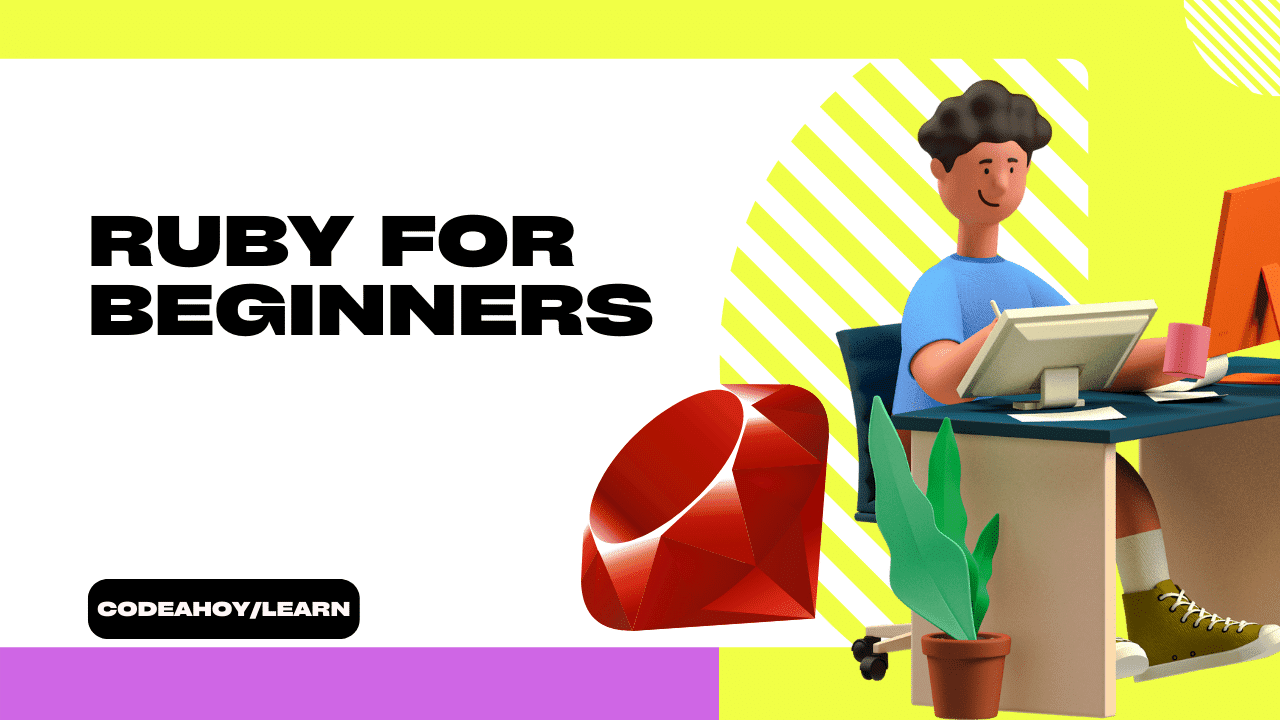
Ruby for Beginners
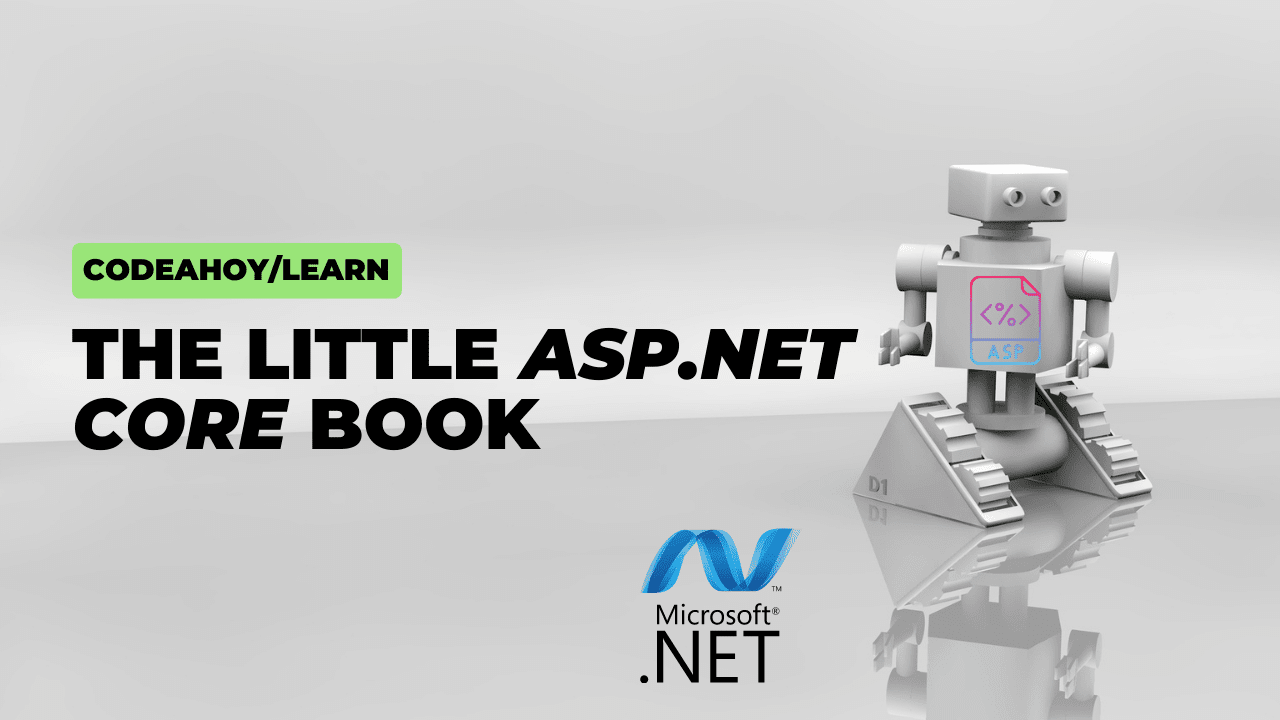
The Little ASP.NET Core Book
Books / Introduction to C Programming Language / Chapter 12
In this chapter, simple & compound statements, conditionals, the while loop, the do..while loop, the for loop, loops with break, continue, and goto, choosing where to put a loop exit.
The bodies of C functions (including the main function) are made up of statements . These can either be simple statements that do not contain other statements, or compound statements that have other statements inside them. Control structures are compound statements like if/then/else, while, for, and do..while that control how or whether their component statements are executed.
Simple statements
The simplest kind of statement in C is an expression (followed by a semicolon, the terminator for all simple statements). Its value is computed and discarded. Examples:
Most statements in a typical C program are simple statements of this form.
Other examples of simple statements are the jump statements return , break , continue , and goto . A return statement specifies the return value for a function (if there is one), and when executed it causes the function to exit immediately. The break and continue statements jump immediately to the end of a loop (or switch ; see below) or the next iteration of a loop; we’ll talk about these more when we talk about loops. The goto statement jumps to another location in the same function, and exists for the rare occasions when it is needed. Using it in most circumstances is a sin.
Compound statements
Compound statements come in two varieties: conditionals and loops, which we’ll explore in depth in the next sections.
These are compound statements that test some condition and execute one or another block depending on the outcome of the condition. The simplest is the if statement:
The body of the if statement is executed only if the expression in parentheses at the top evaluates to true (which in C means any value that is not 0).
The braces are not strictly required, and are used only to group one or more statements into a single statement. If there is only one statement in the body, the braces can be omitted:
This style is recommended only for very simple bodies. Omitting the braces makes it harder to add more statements later without errors.
In the example above, the lack of braces means that the hideInBunker() statement is not part of the if statement, despite the misleading indentation. This sort of thing is why I generally always put in braces in an if .
An if statement may have an else clause, whose body is executed if the test is false (i.e. equal to 0).
A common idiom is to have a chain of if and else if branches that test several conditions:
This can be inefficient if there are a lot of cases, since the tests are applied sequentially. For tests of the form _ _ `==` _ _, the `switch` statement may provide a faster alternative. Here’s a typical `switch` statement:
This prints the string “cow” if there is one cow, “cowen” if there are two cowen, and “cows” if there are any other number of cows. The switch statement evaluates its argument and jumps to the matching case label, or to the default label if none of the cases match. Cases must be constant integer values.
The break statements inside the block jump to the end of the block. Without them, executing the switch with numberOfCows equal to 1 would print all three lines. This can be useful in some circumstances where the same code should be used for more than one case:
or when a case “falls through” to the next:
Note that it is customary to include a break on the last case even though it has no effect; this avoids problems later if a new case is added. It is also customary to include a default case even if the other cases supposedly exhaust all the possible values, as a check against bad or unanticipated inputs.
Though switch statements are better than deeply nested if/else-if constructions, it is often even better to organize the different cases as data rather than code. We’ll see examples of this when we talk about function pointers .
Nothing in the C standards prevents the case labels from being buried inside other compound statements. One rather hideous application of this fact is Duff’s device .
There are three kinds of loops in C.
A while loop tests if a condition is true, and if so, executes its body. It then tests the condition is true again, and keeps executing the body as long as it is. Here’s a program that deletes every occurrence of the letter e from its input.
Note that the expression inside the while argument both assigns the return value of getchar to c and tests to see if it is equal to EOF (which is returned when no more input characters are available). This is a very common idiom in C programs. Note also that even though c holds a single character, it is declared as an int . The reason is that EOF (a constant defined in stdio.h ) is outside the normal character range, and if you assign it to a variable of type char it will be quietly truncated into something else. Because C doesn’t provide any sort of exception mechanism for signalling unusual outcomes of function calls, designers of library functions often have to resort to extending the output of a function to include an extra value or two to signal failure; we’ll see this a lot when the null pointer shows up in the chapter on pointers .
The do .. while statement is like the while statement except the test is done at the end of the loop instead of the beginning. This means that the body of the loop is always executed at least once.
Here’s a loop that does a random walk until it gets back to 0 (if ever). If we changed the do .. while loop to a while loop, it would never take the first step, because pos starts at 0.
examples/statements/randomWalk.c
The do .. while loop is used much less often in practice than the while loop.
It is theoretically possible to convert a do .. while loop to a while loop by making an extra copy of the body in front of the loop, but this is not recommended since it’s almost always a bad idea to duplicate code.
The for loop is a form of syntactic sugar that is used when a loop iterates over a sequence of values stored in some variable (or variables). Its argument consists of three expressions: the first initializes the variable and is called once when the statement is first reached. The second is the test to see if the body of the loop should be executed; it has the same function as the test in a while loop. The third sets the variable to its next value. Some examples:
A for loop can always be rewritten as a while loop.
The break statement immediately exits the innermmost enclosing loop or switch statement.
The continue statement skips to the next iteration. Here is a program with a loop that iterates through all the integers from -10 through 10, skipping 0:
examples/statements/inverses.c
Occasionally, one would like to break out of more than one nested loop. The way to do this is with a goto statement.
The target for the goto is a label , which is just an identifier followed by a colon and a statement (the empty statement ; is ok).
The goto statement can be used to jump anywhere within the same function body, but breaking out of nested loops is widely considered to be its only genuinely acceptable use in normal code.
Choosing where to put a loop exit is usually pretty obvious: you want it after any code that you want to execute at least once, and before any code that you want to execute only if the termination test fails.
If you know in advance what values you are going to be iterating over, you will most likely be using a for loop:
Most of the rest of the time, you will want a while loop:
The do .. while loop comes up mostly when you want to try something, then try again if it failed:
Finally, leaving a loop in the middle using break can be handy if you have something extra to do before trying again:
(Note the empty for loop header means to loop forever; while(1) also works.)
Licenses and Attributions
This book is licensed under Creative Commons Attribution-ShareAlike 4.0
Original Content CC-BY-SA 4.0 International
- This work is a derivative of "Notes on Data Structures and Programming Techniques (CPSC 223, Spring 2022)" by James Aspnes , used under Creative Commons Attribution-ShareAlike 4.0. Original copyright notice: Copyright © 2001–2021 by James Aspnes. Distributed under a Creative Commons Attribution-ShareAlike 4.0 International license: https://creativecommons.org/licenses/by-sa/4.0/.
Other Content, as indicated:
- "GitHub" , "Git" from Wikipedia under the Creative Commons Attribution-ShareAlike License 3.0 / Lightly modified from original
- "Linked list" , "Git" from Wikipedia under the Creative Commons Attribution-ShareAlike License 3.0 / Lightly modified from original
- Foto from Wikipedia, by Jonn Leffmann.
Speak Your Mind Cancel reply
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, introduction.
- Getting Started with C
- Your First C Program
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
Flow Control
- C if...else Statement
- C while and do...while Loop
C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
Programming Strings
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
String Examples in C Programming
Structure and Union
- C structs and Pointers
- C Structure and Function
Programming Files
- C File Handling
- C Files Examples
Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
- Display Armstrong Number Between Two Intervals
C Function Examples
- Display Prime Numbers Between Two Intervals
- Check Whether a Number is Prime or Not
- Check Whether a Number can be Expressed as Sum of Two Prime Numbers
- Check Prime or Armstrong Number Using User-defined Function
C Control Flow Examples
To understand all the examples on this page, you should know about the following topics:
- if...else Statement
- break and continue
- switch...case
Sorry about that.
Related Tutorials
C Introduction Examples
Control Structures I (if, if-else, and switch)
Learn how to write the if-else and switch statements in C++.
Relational operators
- Logical operators
- Example: Finding the maximum
- Example: Letter case conversion
- Example: Calculating the grades of students
Control structures
Control structures are fundamental in programming languages that allow developers to control the flow of code execution based on certain conditions. These structures enable programmers to create programs that can make decisions, iterate over collections of data, and execute blocks of code repeatedly. This lesson will cover three such structures:
The if statement
The else if statement
The switch statement
Since all control structures rely on conditional statements that involve relational and logical operators, it’s a good idea to start by refreshing our knowledge of these operators.
Relational operators , also known as comparison operators , are used in programming to compare two values and return a boolean result. C++ provides six comparison operators, which are == , != , > , >= , < , and <= . When a comparison operator is executed, it returns either 1 or 0 , depending on whether the comparison is true or false , respectively. Let’s take a look at an example of a comparison expression and see how it works with different relational operators.
Get hands-on with 1200+ tech skills courses.

IMAGES
VIDEO
COMMENTS
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
Control Structures — The Book of C (version 2022.08) 4. Control Structures ¶. In this chapter, we encounter the set of "control" structures in C, such as conditional statements and looping constructs. As a preview, the control structures in C are nearly identical to those found in Java (since Java syntax is heavily based on C), and bear ...
15.13 Structure Assignment. Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example: Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
The structure in C is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define the structure in the C programming language. The items in the structure are called its member and they can be of any valid data type.
CONTROL STRUCTURES IN C. Control Structures specifies one or more conditions to be evaluated or tested by the program, along with a statement or statements to be executed if the condition is true and optionally, other statements to be executed if the condition is false. See blow image to understand control (Decision making ) structure:-.
Last Updated : 16 Jan, 2020. Control Structures are just a way to specify flow of control in programs. Any algorithm or program can be more clear and understood if they use self-contained modules called as logic or control structures. It basically analyzes and chooses in which direction a program flows based on certain parameters or conditions.
Control Structures In C. Control structures are an essential part of programming languages that allow you to control the flow of execution in a program. They determine the order in which statements are executed and enable you to make decisions and repeat blocks of code based on specific conditions.
With the right understanding of control structures - Conditional, Looping, and Jump Statements in C programming - you'll be ready to navigate any labyrinth your code presents. ... Remember, in real-world scenarios, system checks would be much more complex than a simple random assignment. Example Code - 2. Let's consider a more comprehensive ...
The assignment operator assigns a value to the left variable. The equality operator simply checks to see if the left and right sides are already equal. ... Figure 1: C control structures from this post. "C" you in the next tutorial! Until then comment and tell us what you favorite C programming control structure or loop is. Why do you like it?
Assignment 2 Problem 1. Rewrite the program below, replacing the for loop with a combination of goto and if statements. The output of the program should stay the same. ... In lecture, we covered a variety of control structures for looping, such as for, while, do/while, goto, and several for testing conditions, such as if and switch.
A control structure in C is any code construct that changes the flow of control, such as the order of execution in a program. The three main types of control structures in C are decision-making control structures, looping control structures, and unconditional control structures. Decision-making control structures include if-else blocks and ...
7 Assignment Expressions. As a general concept in programming, an assignment is a construct that stores a new value into a place where values can be stored—for instance, in a variable. Such places are called lvalues (see Lvalues) because they are locations that hold a value. An assignment in C is an expression because it has a value; we call it an assignment expression.
6 System.out.println(" axˆ2 + bx + c = 0 \n"); 7 8 //the values of a,b and c need to be stored in variables 9 double a, b, c; 10 //we also need variables to store our two solutions 11 double x1, x2; 12 13 //we need to read the values of a, b and c from the user 14 //so we need to use the Scanner object 15 Scanner in = new Scanner (System.in ); 16
with questions related to the exercises and the lecture. Programs and quizzes contribute 8% + 8% to overall mark. Strict deadlines (no late submissions possible, sample solutions posted right after deadline) First assessment (week 1) is programming practice and will not count. Deadline: Tuesday, 6 June, 5:00:00pm.
To review the structure and semantics of loops refer to section 7.5 of Touch of class. It happens very often that you want to iterate through all the items of a container, for example all lines in Zurich or all stations of a particular line.
In C programming, there are several common control structures that are used extensively. These include conditional statements like the "if" statement and the "switch" statement, as well as looping structures like the "while" loop, the "for" loop, and the "do-while" loop. These control structures provide flexibility and allow ...
12. A control structure is any mechanism that departs from the default of straight-line execution. » selection. • if statements • case statements. » iteration. • while loops (unbounded) • for loops • iteration over collections. » other. • goto • call/return • exceptions • continuations. Control Structures (1/2)
The normal execution of statements is in sequential fashion but to change the sequential flow, various control structures are used. Broadly, control structures are of three types-. Selection /conditional - executes as per choice of action. Iteration - Performs a repetitive action.
The bodies of C functions (including the main function) are made up of statements. These can either be simple statements that do not contain other statements, or compound statements that have other statements inside them. Control structures are compound statements like if/then/else, while, for, and do..while that control how or whether their ...
Nested Structures. You can create structures within a structure in C programming. For example, struct complex { int imag; float real; }; struct number { struct complex comp; int integers; } num1, num2; Suppose, you want to set imag of num2 variable to 11.
Reverse a number. Calculate the power of a number. Check whether a number is a palindrome or not. Check whether an integer is prime or Not. Display prime numbers between two intervals. Check Armstrong number. Display Armstrong numbers between two intervals. Display factors of a number. Print pyramids and triangles.
C programming related to structures [9 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.Go to the editor]. From Wikipedia - A struct (Structures) in the C programming language (and many derivatives) is a composite data type (or record) declaration that defines a physically grouped list of variables under one name in a block of memory ...
Control structures. Control structures are fundamental in programming languages that allow developers to control the flow of code execution based on certain conditions. These structures enable programmers to create programs that can make decisions, iterate over collections of data, and execute blocks of code repeatedly. This lesson will cover ...